How to Pretty Print a JSON File in Python
- Understanding JSON and Its Importance
- Method 1: Using the json Module
- Method 2: Pretty Printing JSON from a File
- Method 3: Using json.tool for Command-Line Pretty Printing
- Conclusion
- FAQ
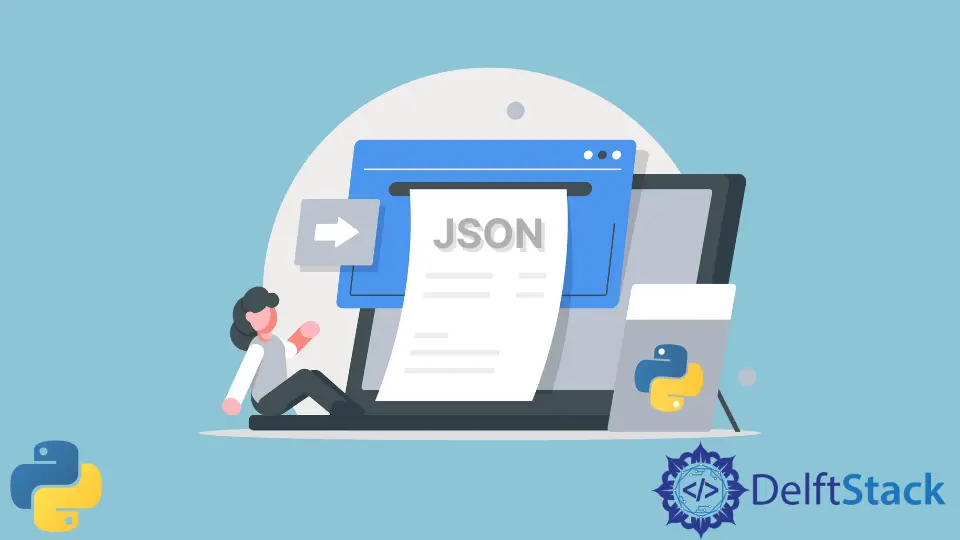
In the world of programming, JSON (JavaScript Object Notation) is a popular format for data interchange. It’s lightweight, easy to read, and perfect for web applications. However, when working with JSON files, the raw format can be hard to read and understand. This is where pretty printing comes into play. Pretty printing formats the JSON data in a way that is more visually appealing, making it easier to navigate and comprehend.
In this tutorial, we will explore how to pretty print a JSON file using Python. Whether you’re a beginner or an experienced developer, this guide will help you enhance the readability of your JSON files effortlessly.
Understanding JSON and Its Importance
JSON is widely used for data transfer between a server and a client in web applications. Its structure is straightforward, consisting of key-value pairs, arrays, and objects. However, when JSON files grow large, their readability diminishes. Pretty printing not only makes JSON files easier to read but also helps in debugging and data analysis. By using Python, we can easily manipulate and format JSON data to suit our needs. Let’s dive into the methods for pretty printing JSON files.
Method 1: Using the json Module
Python’s built-in json
module provides a simple way to pretty print JSON data. The json.dumps()
function can be utilized to convert a Python object into a JSON string with indentation for better readability. Here’s how you can do it:
import json
data = {
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": False,
"courses": ["Math", "Science", "History"]
}
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
Output:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": false,
"courses": [
"Math",
"Science",
"History"
]
}
In this code, we first import the json
module. We then define a Python dictionary named data
, which contains various key-value pairs. The json.dumps()
function is called with the indent
parameter set to 4, which specifies the number of spaces to use for indentation. This results in a well-formatted JSON string that is easy to read. Finally, we print the pretty-printed JSON to the console.
Method 2: Pretty Printing JSON from a File
If you have a JSON file that you want to pretty print, you can read the file, load its content, and then use the same json.dumps()
method to format it. Here’s an example:
import json
with open('data.json', 'r') as file:
data = json.load(file)
pretty_json = json.dumps(data, indent=4)
print(pretty_json)
Output:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": false,
"courses": [
"Math",
"Science",
"History"
]
}
In this example, we open a JSON file named data.json
and read its contents using json.load()
. This function parses the JSON data into a Python dictionary. After that, we apply json.dumps()
with the indent
parameter to create a pretty-printed version of the JSON data. This method is particularly useful for larger JSON files, as it allows you to format and view the contents without manually editing the file.
Method 3: Using json.tool for Command-Line Pretty Printing
For those who prefer working directly in the command line, Python also offers a handy tool called json.tool
. This allows you to pretty print JSON files without writing any code. Here’s how you can use it:
cat data.json | python -m json.tool
Output:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"is_student": false,
"courses": [
"Math",
"Science",
"History"
]
}
In this command, we use cat
to read the contents of data.json
, and then we pipe (|
) that output into the json.tool
module. The python -m json.tool
command processes the incoming JSON data and pretty prints it directly to the terminal. This method is particularly useful for quick checks on JSON files without needing to write a full script.
Conclusion
Pretty printing JSON files in Python is a straightforward process that enhances readability and helps with data management. Whether you choose to use the json
module or the command-line tool, each method provides a clear and efficient way to format your JSON data. By following the techniques outlined in this tutorial, you can ensure that your JSON files are not only functional but also visually appealing. So go ahead, try these methods out, and make your JSON data more accessible!
FAQ
-
What is JSON?
JSON stands for JavaScript Object Notation and is a lightweight data interchange format that is easy for humans to read and write. -
Why should I pretty print JSON?
Pretty printing JSON enhances its readability, making it easier to understand and debug, especially for large files. -
Can I pretty print JSON in other programming languages?
Yes, many programming languages offer libraries or built-in functions to pretty print JSON, including JavaScript, Ruby, and PHP. -
Is pretty printing JSON necessary for all applications?
While not strictly necessary, pretty printing is highly recommended for better readability and debugging, especially during development. -
How can I pretty print a JSON file without using Python?
You can use command-line tools likejq
or online JSON formatters to pretty print JSON files without any programming.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook