How to Compare Multilevel JSON Objects Using JSON Diff in Python
- Compare Multilevel JSON Objects in Python
- Use the Equality Operator to Compare Multilevel JSON Objects in Python
-
Use
jsondiff
to Compare Multilevel JSON Objects in Python
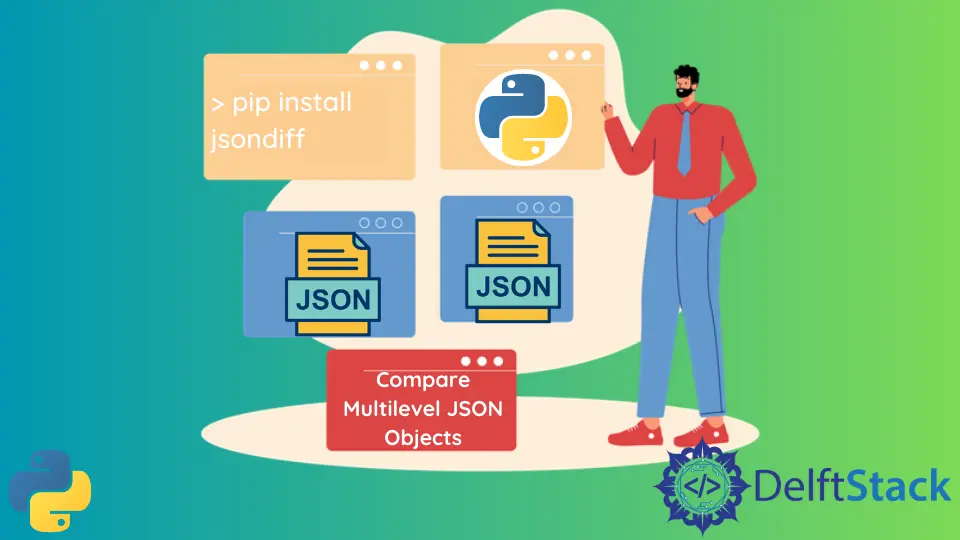
This article aims to demonstrate how we can compare two multilevel JSON objects and determine whether they are identical.
Compare Multilevel JSON Objects in Python
Short for JavaScript Object Notation, JSON objects are mainly used for exchanging data and are generally considered very lightweight. One of its main advantages is that it can be easily understood by humans and machines – as in easily parsed, allowing the device to extract valuable information.
Due to its frequent usage, we may need to compare two objects for whatever reason. It can be to check if their contents are identical or for another purpose.
Consider the following code:
obj1 = {
"countries": [
{
"name": "Great Britian",
"cities": [{"name": "Manchester"}, {"name": "London"}],
}
]
}
obj2 = {"countries": [{"name": "Great Britian", "cities": [{"name": "London"}]}]}
The code snippet shows that we have two JSON objects storing information about countries and their cities. An interesting thing to note here is that they are multi-leveled, meaning the objects contain more JSON objects that are nested within.
Use the Equality Operator to Compare Multilevel JSON Objects in Python
Before delving into any third-party libraries, checking if our task is doable via the standard Python functions is best. Fortunately for us, equality works just as well for our use case.
Although, if you need more control over variables, such as filtering data or looking for specific changes, you might need to use a third-party library since the equality operator does not provide as much flexibility as one might need. For more straightforward cases, using the equality operator is more than enough.
Consider the following code:
obj1 = {
"errors": [{"error": "err1", "fld": "mail"}, {"error": "err2", "fld": "name"}],
"success": "false",
}
obj2 = {
"errors": [{"error": "err1", "fld": "mail"}, {"error": "err2", "fld": "name"}],
"success": "false",
}
obj3 = {
"errors": [
{"error": "err1", "fld": "mail"},
{"error": "err2", "fld": "name"},
{"error": "err3", "fld": "time"},
],
"success": "false",
}
print(obj1 == obj2)
print(obj2 == obj3)
Output:
True
False
In the following, it can be seen that three JSON objects are declared, out of which two are the same while one is different. Using the equality operator, we can determine whether they are the same.
This approach is more accessible to implement than any third-party library and saves the hassle of reviewing the libraries’ documentation. On the other hand, it does not provide much functionality, which, if required, can be achieved using a third-party module/library.
Use jsondiff
to Compare Multilevel JSON Objects in Python
jsondiff
is a third-party, open-source module that can be used to differentiate between JSON and JSON-like structures. Using this library, it can become pretty easy to find differences between JSON objects, be they multi-leveled or unordered.
Before jumping to see how we can do so, let’s first install jsondiff
since it’s a third-party module and does not come built-in with any standard Python installation.
Guide to Install jsondiff
Open the command prompt and execute the below command to start installing jsondiff
:
pip install jsondiff
Output:
Collecting jsondiff
Downloading jsondiff-2.0.0-py3-none-any.whl (6.6 kB)
Installing collected packages: jsondiff
Successfully installed jsondiff-2.0.0
After jsondiff
has been installed, we can start using it to accomplish our task.
Consider the following code:
import jsondiff
obj1 = {
"countries": [
{
"name": "Great Britian",
"cities": [{"name": "Manchester"}, {"name": "London"}],
}
]
}
obj2 = {
"countries": [
{
"name": "Great Britian",
"cities": [{"name": "Manchester"}, {"name": "London"}],
}
]
}
res = jsondiff.diff(obj1, obj2)
if res:
print("Diff found")
else:
print("Same")
Output:
Same
In the following code, we used the third-party library jsondiff
to find whether the two objects are the same or not. To be more specific, we used the function jsondiff.diff()
, passing our JSON objects named obj1
and obj2
.
How jsondiff.diff
work is that if it finds any changes, it returns them, which we save in a variable named res
. Whether there are any differences, we can quickly determine if the compared objects are identical or different.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn