How to Flatten JSON in Python
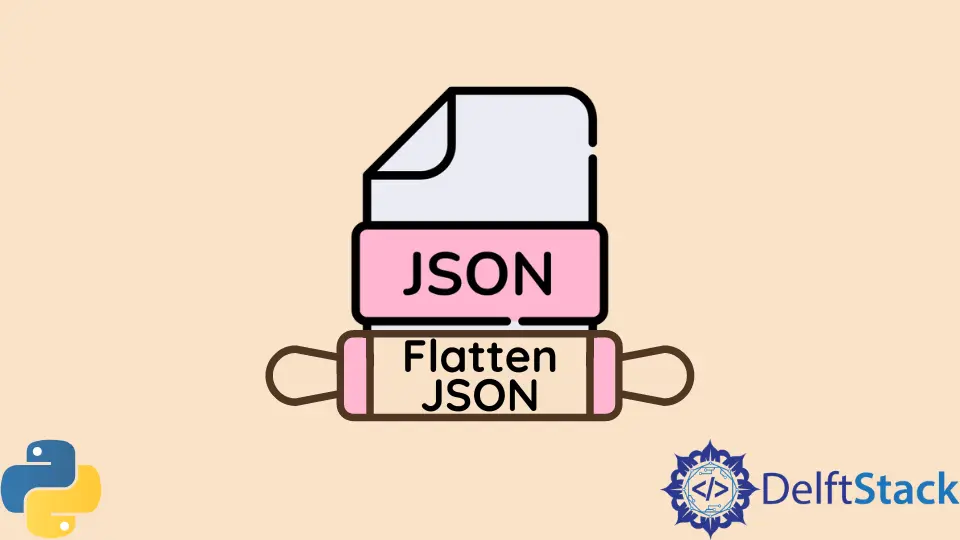
This tutorial will discuss flattening JSON using conditional statements, loops, and the type()
function in Python.
Flatten JSON in Python
JSON is a file format, and it stands for JavaScript Object Notation. JSON stores and transmits data that a human can read and understand.
We see different attributes with values separated by arrays or lists in a JSON file. For example, we can use a JSON file to store students’ data like their name, age, address, and class.
Each student’s data will be stored in an array containing attributes and values of different data types. For example, an example JSON file is given below.
{"Ali" : {
"age": 18,
"profession": "student"},
"Ammar" : {
"age" : "nineteen",
"profession": "mechanic"}
}
The above file contains information about two persons, which we can read using the json
library of Python. For example, read the above file using the json
library and display its content.
See the code below.
import json
json_file = open("people.json")
data = json.load(json_file)
print("Original Data\n", data)
Output:
Original Data
{'Ali': {'age': 18, 'profession': 'student'}, 'Ammar': {'age': 'nineteen', 'profession': 'mechanic'}}
We have to provide the file name and its extension in the open()
function to read it, and if the file is not in the same directory as the Python file, we have to provide the full path of the file along with its name and extension inside the open()
function. We used the load()
function of json
library to read the JSON data.
We can get a single person’s data using his name inside the data variable like data['Ali']
. If we have a multilevel JSON file containing a large amount of data, it becomes difficult to read the value of a specific attribute.
Inside a multilevel JSON file, we have attributes inside other attributes like lists or dicts
. We can combine attribute names if we want to convert the multilevel JSON file to a single level.
For example, in the above code, if we want to get the age of Ali
, we can access it using data['Ali']['age']
, but if we combine the attribute names, we can use data['Ali_age']
to get the age of Ali
.
We can use a recursive function that will call itself multiple times to flatten the JSON data. We have to read data from the JSON file and use two loops to check for list
and dict
in the data.
We will use loops to combine the attributes’ names with a delimiter and save its value in a dict
format. The loops will stop when no more data is in the JSON file, and the result will be returned.
For example, let’s flatten the above JSON file. See the code below.
import json
json_file = open("people.json")
data = json.load(json_file)
print("Original Data\n", data)
def flatten_json_data(json_data):
out = {}
def flatten_json(d, name=""):
if type(d) is dict:
for a in d:
flatten_json(d[a], name + a + "_")
elif type(d) is list:
i = 0
for a in d:
flatten_json(a, name + str(i) + "_")
i += 1
else:
out[name[:-1]] = d
flatten_json(json_data)
return out
flattened_data = flatten_json_data(data)
print("\nflatten Data\n", flattened_data)
Output:
Original Data
{'Ali': {'age': 18, 'profession': 'student'}, 'Ammar': {'age': 'nineteen', 'profession': 'mechanic'}}
flatten Data
{'Ali_age': 18, 'Ali_profession': 'student', 'Ammar_age': 'nineteen', 'Ammar_profession': 'mechanic'}
In the above code, we use the if-else
conditional statement to check the input data type and the type()
function, which returns the data type of objects. While reading the data, if a dict
comes, the first loop will work; if a list comes, the second loop will work.
In the above output, we can see that the original data contains two dicts
, but the flattened data contains only one dict
. The example JSON file does not contain any lists, but the above code will also work with JSON files containing lists.
There is a difference in the original data and the flattened data; we can get the age and profession of a person at the same time using the original data because age and profession have the same parent attribute, which is the name of the person, but we cannot do the same using the flattened data.
To get the age and profession of a person using the flattened data, we have to get them separately one by one.
A flattened JSON file is useful when we only have to get a single value, but we can use the original JSON file if we want to get all the information about an object or attribute. Check this link for more details about the JSON library.