How to Serialize a Python Class Object to JSON
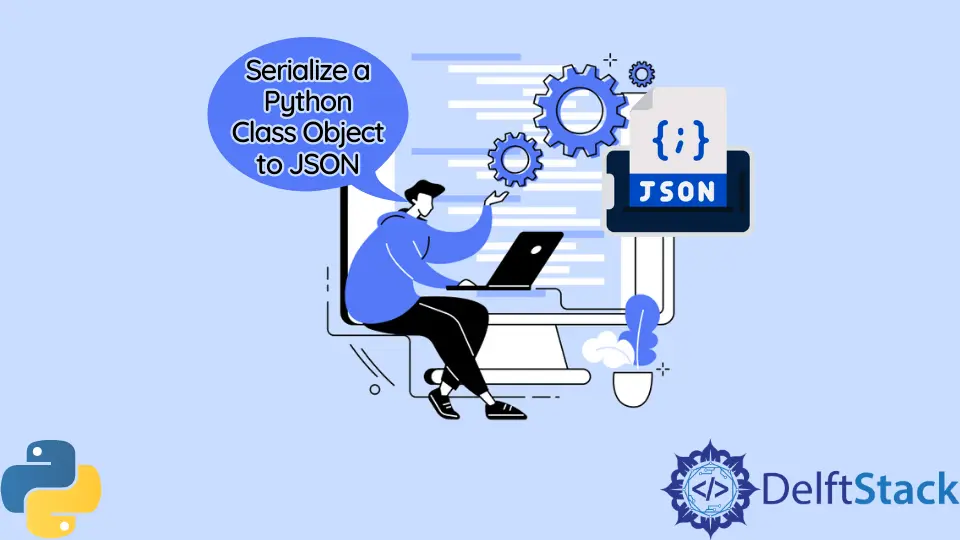
This tutorial educates about the serialization process. It also illustrates how we can make a JSON class serializable using the toJSON()
method and wrapping JSON to dump in its class.
Serialization in Python
The serialization process involves transforming an object into a format that can be preserved and retrieved later. Such as by recording the state of an object into a file.
All developers must do serialization at some point for all projects that are even remotely sophisticated. The Python programming language’s simplicity in many typical programming jobs is one of its excellent features.
With a few lines of code, file IO, drawing graphs, and accessing online content are all made simple. Likewise, python makes serialization simple, except when trying to serialize a custom class.
Let’s learn how to serialize your class objects to JSON objects below.
Make a Python Class JSON Serializable
The built-in json
module of Python can handle only Python primitive types with a direct JSON counterpart.
Expressly, the default functionality of the JSON encoder, json.dump()
and json.dumps()
is limited to serializing the most basic set of object types (e.g., dictionary, lists, strings, numbers, None, etc.).
To fix this, we must create a unique encoder that allows our class JSON to be serializable.
It is possible to make a Python class JSON serializable in various methods. Choose the option that best fits the complexity of your situation.
There are two primary methods of making a JSON class serializable that are given below:
- The
toJSON()
method - Wrap JSON to dump in its class
Use the toJSON()
Method to Make a JSON Class Serializable
We can implement the serializer method instead of the JSON serializable class. For example, in this method, the Object
class might have a JSON function that returns a dictionary of the Object
information.
Since all of the Object
attributes are native types, we can send this dictionary into an encoding function to obtain the JSON notation. Finally, we return the values of the attributes that make up an object.
import json
class Object:
def toJSON(self):
return json.dumps(self, default=lambda o: o.__dict__, sort_keys=True, indent=4)
me = Object()
me.name = "kelvin"
me.age = 20
me.dog = Object()
me.dog.name = "Brunno"
print(me.toJSON())
Output:
{
"age": 20,
"dog": {
"name": "Brunno"
},
"name": "kelvin"
}
Wrap JSON to Dump in Its Class
Another solution is to subclass a JsonSerializable
class to create a FileItem
class that wraps JSON dumping, as shown in the following code.
Example Code:
from typing import List
from dataclasses import dataclass, asdict, field
from json import dumps
@dataclass
class Students:
id: 1
name: "stu1"
@property
def __dict__(self):
return asdict(self)
@property
def json(self):
return dumps(self.__dict__)
test_object_1 = Students(id=1, name="Kelvin")
print(test_object_1.json)
Output:
{"id": 1, "name": "Kelvin"}
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python JSON
- How to Get JSON From URL in Python
- How to Pretty Print a JSON File in Python
- How to Append Data to a JSON File Using Python
- How to Compare Multilevel JSON Objects Using JSON Diff in Python
- How to Flatten JSON in Python