Python Class Equality
- Equality of Class Objects in Python
-
Python Class Equality Using the
__eq__()
Method -
Python Class Equality Using the
id()
Method
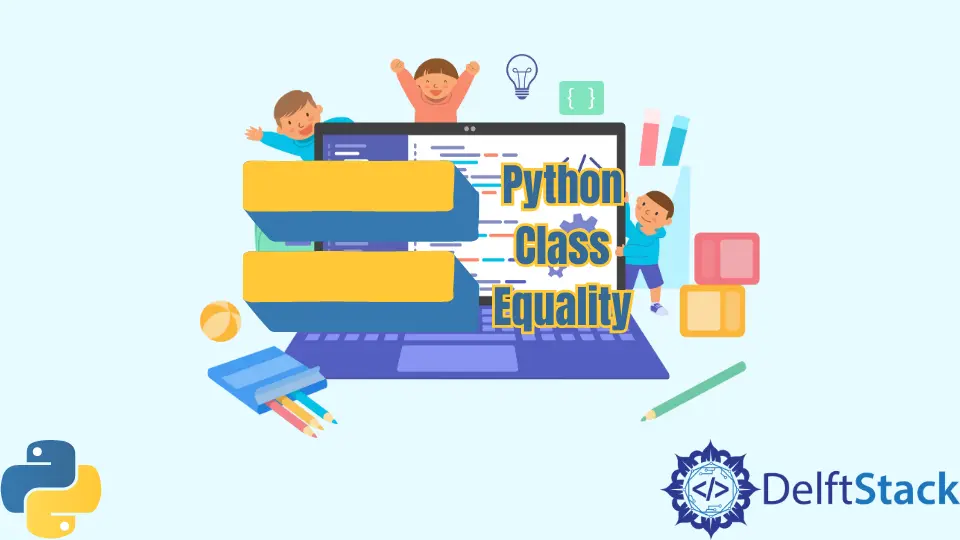
In Python, we can compare different data types using comparison operators. However, we cannot simply compare them using the comparison operators when creating custom classes.
This article will discuss different ways to check the equality of objects defined using custom classes in Python.
Equality of Class Objects in Python
When we have built-in objects like integers or strings, we can easily check for their equality using the ==
operator, as shown below.
num1 = 12
num2 = 10
result = num1 == num2
print("{} and {} are equal:{}".format(num1, num2, result))
Output:
12 and 10 are equal:False
Here, the ==
operator gives the correct value as output because the values 12 and 10 are integers. However, when we have objects of custom classes, the Python interpreter works differently.
For instance, suppose that we have a Length
class with only one attribute, length
, as shown below.
class Length:
def __init__(self, value):
self.length = value
We will create two instances of the class Length
with the same value in the length
attribute.
class Length:
def __init__(self, value):
self.length = value
len1 = Length(10)
len2 = Length(10)
If you compare the objects using the ==
operator, the result will be False
even though both the instances have the same value in the length
attribute. You can observe this in the following code.
class Length:
def __init__(self, value):
self.length = value
len1 = Length(10)
len2 = Length(10)
result = len1 == len2
print("len1 and len2 are equal:", result)
Output:
len1 and len2 are equal: False
The above behavior of the Python interpreter can be described using the way it compares two objects of user-defined classes. When we check the equality of two class objects in Python using the ==
operator, the result will be True
only if both the objects refer to the same memory location.
In other words, there will be two variables but only a single Python object. You can observe this in the following example.
class Length:
def __init__(self, value):
self.length = value
len1 = Length(10)
len2 = len1
result = len1 == len2
print("len1 and len2 are equal:", result)
Output:
len1 and len2 are equal: True
You might have understood that the equality operator will return True
only when both the variables refer to the same instance of the user-defined class.
What should we do if we need to check the equality of different instances of a class in Python? Let us find out.
Python Class Equality Using the __eq__()
Method
By overriding the __eq__()
method, we can modify how the ==
operator works with custom classes. For instance, to check the length of two instances of the Length
class, we can override the __eq__()
method.
We will use the steps discussed below inside the __eq__()
method.
-
The
__eq__()
method, when invoked on an instance of theLength
class, will take another object as its input argument. -
Inside the
__eq__()
method, we will first check if the input object is an instance of theLength
class or not. For this, we can use theisinstance()
function. -
The
isinstance()
function takes a Python object as its first input argument and the class name as its second input argument. After execution, it returnsTrue
if the object is an instance of the class provided in the input argument. -
We will pass the
Length
class as the second input argument in our program. If the object passed in the first argument is not an instance of theLength
class, it will returnFalse
.Otherwise, we will proceed ahead.
-
To check for class equality of the two objects, we will compare the attribute
length
value in both objects. If the values are equal, we will returnTrue
.Otherwise, we will return
False
.
Once the __eq__()
method is implemented in the Length
class, we can correctly compare two instances of the Number
class using the ==
operator.
Suppose we have two instances of the Length
class, say len1
and len2
. When we perform len1==len2
, the len1.__eq__(len2)
method will be executed.
Similarly, when we perform len2==len1
, the len2.__eq__(len1)
method will be executed.
After executing the code, len1==len2
will return True
if both objects’ length value has the same value. Otherwise, it will return False
.
You can observe this in the following example.
class Length:
def __init__(self, value):
self.length = value
def __eq__(self, other):
isLength = isinstance(other, self.__class__)
if not isLength:
return False
if self.length == other.length:
return True
else:
return False
len1 = Length(10)
len2 = Length(10)
result = len1 == len2
print("len1 and len2 are equal:", result)
Output:
len1 and len2 are equal: True
Python Class Equality Using the id()
Method
You can also check if two variables having objects of custom classes refer to the same object or not. For this, you can use the id()
function.
The id()
function takes an object as its input argument and returns a unique identity number at any memory location. You can observe this in the following example.
class Length:
def __init__(self, value):
self.length = value
def __eq__(self, other):
isLength = isinstance(other, self.__class__)
if not isLength:
return False
if self.length == other.length:
return True
else:
return False
len1 = Length(10)
len2 = Length(10)
result1 = id(len1)
result2 = id(len2)
print("ID of len1 is ", result1)
print("ID of len2 is ", result2)
Output:
ID of len1 is 140057455513712
ID of len2 is 140057454483488
If two objects refer to the same memory location, the id()
function will give the same output for both objects. By comparing the output of the id()
function, we can check if the objects refer to the same memory location or not.
You can observe this in the following example.
class Length:
def __init__(self, value):
self.length = value
def __eq__(self, other):
isLength = isinstance(other, self.__class__)
if not isLength:
return False
if self.length == other.length:
return True
else:
return False
len1 = Length(10)
len2 = Length(10)
result1 = id(len1)
result2 = id(len2)
result = result1 == result2
print("len1 and len2 are equal:", result)
Output:
len1 and len2 are equal: False
Here, you can observe that we haven’t checked the value of the attributes in the objects to check for class equality.
In this case, we are only checking if the objects refer to the same memory location or not. Thus, this approach to checking Python class equality is equivalent to using the ==
operator without implementing the __eq__()
method in the class definition.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub