Python Class Factory
- How to Create a Class Factory in Python
-
Use the
class
Keyword to Create a Class Factory in Python -
Use the
type
Keyword to Create a Class Factory in Python
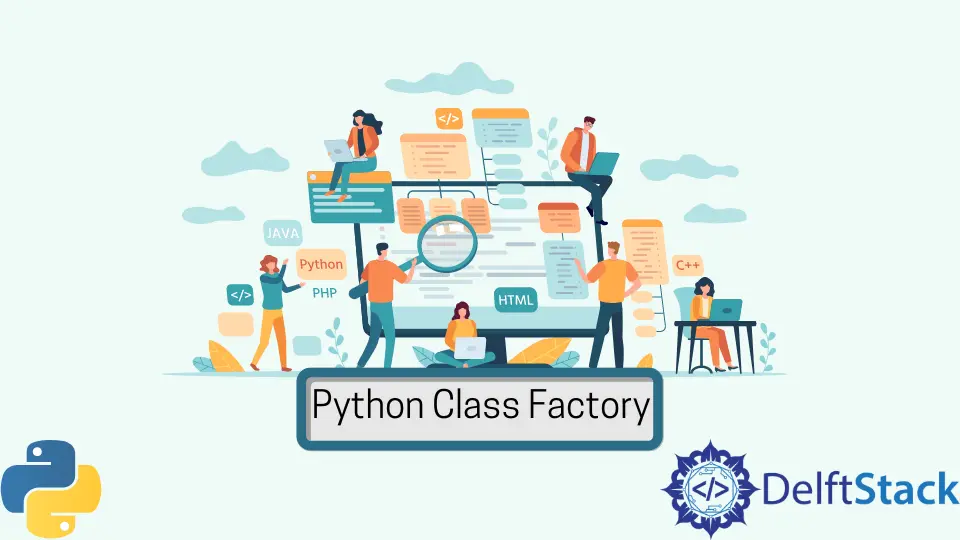
A simple function whose purpose is to create a class and return it is known as a Class Factory. A class factory, being one of the powerful patterns, is extensively utilized in Python.
This tutorial demonstrates the different ways available to create a class factory.
How to Create a Class Factory in Python
There are two methods of designing a class factory; one creates a class during the coding time while the other creates a class during the run time.
The former uses the class
keyword, while the latter uses the type
keyword. Both of these methods are explained and contrasted in the article below.
Use the class
Keyword to Create a Class Factory in Python
We can create a class factory using the class
keyword. For this, we need to create a function and hold a class within the definition of the function.
The following code uses the class
keyword to create a class factory in Python.
def ballfun():
class Ball(object):
def __init__(self, color):
self.color = color
def getColor(self):
return self.color
return Ball
Ball = ballfun()
ballObj = Ball("green")
print(ballObj.getColor())
The code above provides the following output.
green
Use the type
Keyword to Create a Class Factory in Python
The type
keyword allows the dynamic creation of classes. We need to utilize the type
keyword to make a class factory in Python.
However, we should note that with the utilization of the type
keyword, the functions will be left in the namespace only, right with the class.
The following code uses the type
keyword to create a dynamic class in Python.
def init(self, color):
self.color = color
def getColor(self):
return self.color
Ball = type(
"Ball",
(object,),
{
"__init__": init,
"getColor": getColor,
},
)
ballGreen = Ball(color="green")
print(ballGreen.getColor())
The code above provides the following output.
green
The type
keyword allows dynamic classes and effective creation at run time and disadvantages. As you can see in the above code, both the init
and the getColor
functions are cluttered in the namespace.
Moreover, the prospect of reusability of these functions is also lost when the dynamic classes are created using the type
keyword.
An easy solution to this would be the introduction of a class factory. It helps both ways as it decreases the cluttering in the code and promotes the reusability of the functions.
The following code uses the type
keyword to create a class factory in Python.
def create_ball_class():
def init(self, color):
self.color = color
def getColor(self):
return self.color
return type(
"Ball",
(object,),
{
"__init__": init,
"getColor": getColor,
},
)
Ball = create_ball_class()
ballObj = Ball("green")
print(ballObj.getColor())
The code above provides the following output.
green
Now that we have seen how to create a class factory, it is also important to distinguish when to and when not to use the newly learned concept of class factories.
In general, Class Factories are useful when we are unaware of what attributes are to be assigned when the coding occurs.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn