How to Append Data to a JSON File Using Python
- Append Data to a JSON File Using Python
-
Use a Python
list
Object to Update Data to a JSON File -
Use a Python
dict
Object to Update Data to a JSON File
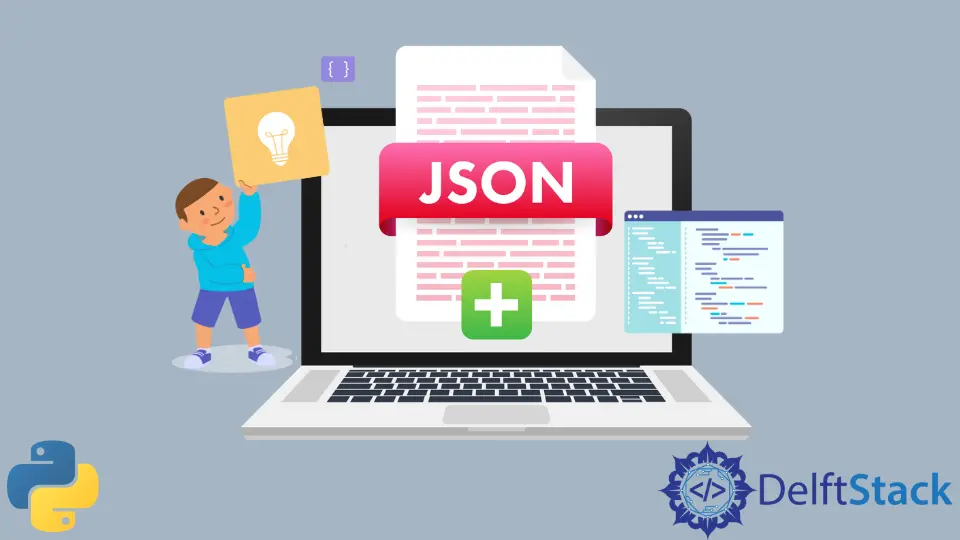
Users are provided with data in JSON format by most of the web applications & Rest APIs because it is widely used and easy to understand in web applications. So, if you are using Python in web applications, you may be interested in appending data to a JSON file using Python.
This tutorial educates possible ways to append data to a JSON file using Python.
Append Data to a JSON File Using Python
We cannot append a JSON file directly using Python, but we can overwrite it. So, how to append data in a JSON file?
For that, we will have to follow the steps given below:
-
Read the JSON File in a Python
dict
or alist
object. -
Append that
dict
orlist
object. -
Write that updated
dict
orlist
object to the original JSON file (Here, the previous content will be overwritten with the updated content).
Our JSON file (data.json
) holds the data given below that we will be using in upcoming code examples in this article.
JSON File Content (saved in data.json
file):
{
"student_details":[
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
}
]
}
Use a Python list
Object to Update Data to a JSON File
Suppose we want to add the following student in the data.json
file:
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
So, let’s run the following code to do it.
Example Code (saved in demo.py
):
import json
def write_json(new_student, filename="./data.json"):
with open(filename, "r+") as file:
file_content = json.load(file)
file_content["student_details"].append(new_student)
file.seek(0)
json.dump(file_content, file, indent=4)
new_student = {
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com",
}
write_json(new_student)
First, we import the json
module to work with JSON files. Next, we write a write_json()
function, which takes two parameters: the new_student
having the student details we want to append and the filename
(we can also specify the file path here).
Inside this function, we use the open()
method to open the specified file in reading mode. Then, we use json.loads()
to parse the JSON string (load the existing data) in the file_content
.
Next, we use list object to use .append()
to join the file_content
with the new_student
inside the student_details
. The file.seek(0)
sets the file’s current position at offset.
Finally, we use .dump()
to convert it back to JSON.
Now, we run the above code using python demo.py
as follows:
PS E:\Code> python demo.py
Our data.json
file will be overwritten on successful execution of the above program. See the updated data.json
file below.
OUTPUT (file content of data.json
):
{
"student_details": [
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
},
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
]
}
Use a Python dict
Object to Update Data to a JSON File
Now, suppose we want to add one more property, "section": "A"
for all students. We can do that as follows:
Example Code (saved in demo.py
file):
import json
def write_json(section, filename="./data.json"):
with open(filename, "r+") as file:
file_content = json.load(file)
file_content.update(section)
file.seek(0)
json.dump(file_content, file, indent=4)
section = {"section": "A"}
write_json(section)
This example is similar to the last one, which used the list
object to update data to a JSON file, except for one difference. Here, we use a dict
object to use .update()
method which updates a dict
(dictionary) with elements from another dict
object or from an iterable key-value pair.
We will have the following content in the data.json
file after running this programming using the python demo.py
command.
OUTPUT (saved in data.json
file):
{
"student_details": [
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
},
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
],
"section": "A"
}