Python を使用して JSON ファイルにデータを追加する
- Python を使用して JSON ファイルにデータを追加する
-
Python
list
オブジェクトを使用してデータを JSON ファイルに更新する -
Python
dict
オブジェクトを使用してデータを JSON ファイルに更新する
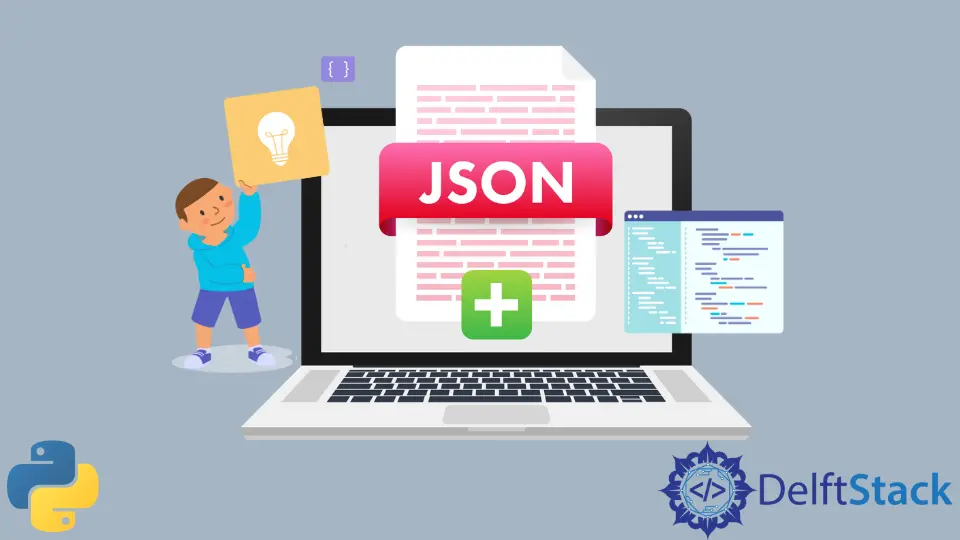
JSON 形式のデータは、Web アプリケーションで広く使用されており、理解しやすいため、ほとんどの Web アプリケーションと Rest API によってユーザーに提供されます。 そのため、Web アプリケーションで Python を使用している場合は、Python を使用して JSON ファイルにデータを追加することに関心があるかもしれません。
このチュートリアルでは、Python を使用して JSON ファイルにデータを追加する方法について説明します。
Python を使用して JSON ファイルにデータを追加する
Python を使用して JSON ファイルを直接追加することはできませんが、上書きすることはできます。 では、JSON ファイルにデータを追加する方法は?
そのためには、以下の手順に従う必要があります。
-
Python
dict
またはlist
オブジェクトで JSON ファイルを読み取ります。 -
その
dict
またはlist
オブジェクトを追加します。 -
その更新された
dict
またはlist
オブジェクトを元の JSON ファイルに書き込みます (ここでは、以前のコンテンツは更新されたコンテンツで上書きされます)。
JSON ファイル (data.json
) には、この記事の今後のコード例で使用する以下のデータが含まれています。
JSON ファイルの内容 (data.json
ファイルに保存):
{
"student_details":[
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
}
]
}
Python list
オブジェクトを使用してデータを JSON ファイルに更新する
次の生徒を data.json
ファイルに追加するとします。
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
それでは、次のコードを実行してみましょう。
コード例 (demo.py
に保存):
import json
def write_json(new_student, filename="./data.json"):
with open(filename, "r+") as file:
file_content = json.load(file)
file_content["student_details"].append(new_student)
file.seek(0)
json.dump(file_content, file, indent=4)
new_student = {
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com",
}
write_json(new_student)
まず、json
モジュールをインポートして、JSON ファイルを操作します。 次に、write_json()
関数を記述します。この関数は、追加する生徒の詳細を含む new_student
と filename
(ここでファイル パスを指定することもできます) の 2つのパラメーターを受け取ります。
この関数内では、open()
メソッドを使用して、指定されたファイルを読み取りモードで開きます。 次に、json.loads()
を使用して、file_content
内の JSON 文字列を解析 (既存のデータをロード) します。
次に、list オブジェクトを使用して、.append()
を使用して、file_content
と student_details
内の new_student
を結合します。 file.seek(0)
は、ファイルの現在位置をオフセットに設定します。
最後に、.dump()
を使用して JSON に変換します。
次に、次のように python demo.py
を使用して上記のコードを実行します。
PS E:\Code> python demo.py
data.json
ファイルは、上記のプログラムが正常に実行されると上書きされます。 以下の更新された data.json
ファイルを参照してください。
出力 (data.json
のファイル内容):
{
"student_details": [
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
},
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
]
}
Python dict
オブジェクトを使用してデータを JSON ファイルに更新する
ここで、すべての生徒に"section": "A"
というプロパティをもう 1つ追加するとします。 次のように実行できます。
サンプルコード (demo.py
ファイルに保存):
import json
def write_json(section, filename="./data.json"):
with open(filename, "r+") as file:
file_content = json.load(file)
file_content.update(section)
file.seek(0)
json.dump(file_content, file, indent=4)
section = {"section": "A"}
write_json(section)
この例は、list
オブジェクトを使用してデータを JSON ファイルに更新した最後の例と似ていますが、1つの違いがあります。 ここでは、dict
オブジェクトを使用して .update()
メソッドを使用します。このメソッドは、dict
(辞書) を別の dict
オブジェクトまたは反復可能なキーと値のペアの要素で更新します。
python demo.py
コマンドを使用してこのプログラミングを実行すると、data.json
ファイルに次の内容が含まれます。
出力 (data.json
ファイルに保存):
{
"student_details": [
{
"student_first_name": "Mehvish",
"student_last_name": "Ashiq",
"student_email": "mehvish@gmail.com"
},
{
"student_first_name": "Tahir",
"student_last_name": "Raza",
"student_email": "tahir@yahoo.com"
},
{
"student_first_name": "Aftab",
"student_last_name": "Raza",
"student_email": "Aftab@gmail.com"
}
],
"section": "A"
}