Python で JSON ファイルをきれいに出力する方法
胡金庫
2023年1月30日
Python
Python JSON
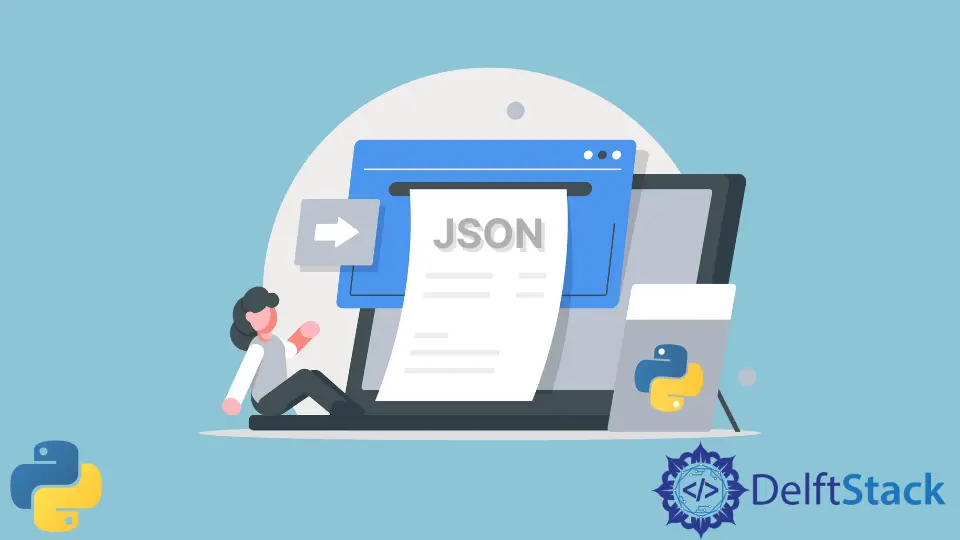
JSON ファイルを文字列に読み込むかロードすると、JSON ファイルの内容が乱雑になる可能性があります。
たとえば、1つの JSON ファイルでは、
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
ロードしてから出力する場合。
import json
with open(r"C:\test\test.json", "r") as f:
json_data = json.load(f)
print(json_data)
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
結果は、通常見られる標準形式に比べて読みやすくなっています。
[
{
"foo": "Etiam",
"bar": [
"rhoncus",
0,
"1.0"
]
}
]
json.dumps
メソッド
json.dumps()
関数は、指定された obj
を JSON 形式の str
にシリアル化します。
指定されたインデントレベルで obj
をきれいに出力するには、json.dumps()
関数のキーワードパラメーターindent
に正の整数を与える必要があります。ident
が 0 に設定されている場合、新しい行のみが挿入されます。
import json
with open(r"C:\test\test.json", "r") as f:
json_data = json.load(f)
print(json.dumps(json_data, indent=2))
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
pprint
メソッド
pprint
モジュールは Python データ構造をきれいに出力する機能を提供します。pprint.pprint
は Python オブジェクトをストリームにきれいに出力し、その後に改行が続きます。
import json
import pprint
with open(r"C:\test\test.json", "r") as f:
json_data = f.read()
json_data = json.loads(json_data)
pprint.pprint(json_data)
JSON ファイルのデータコンテンツはきれいに出力されます。また、indent
パラメーターを割り当てることでインデントを定義することもできます。
pprint.pprint(json_data, indent=2)
注意
pprint
は一重'
と二重引用符"
を同じように扱いますが、JSON
は"
のみを使用するため、pprinted
JSON
ファイルの内容をファイルに直接保存することはできません。
そうしないと、新しいファイルは有効な JSON
形式として解析されません。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫