How to Write to CSV Line by Line in Python
- Write Data to a CSV File With Traditional File Handling in Python
-
Write Data to a CSV File With the
csv.writer()
Function in Python
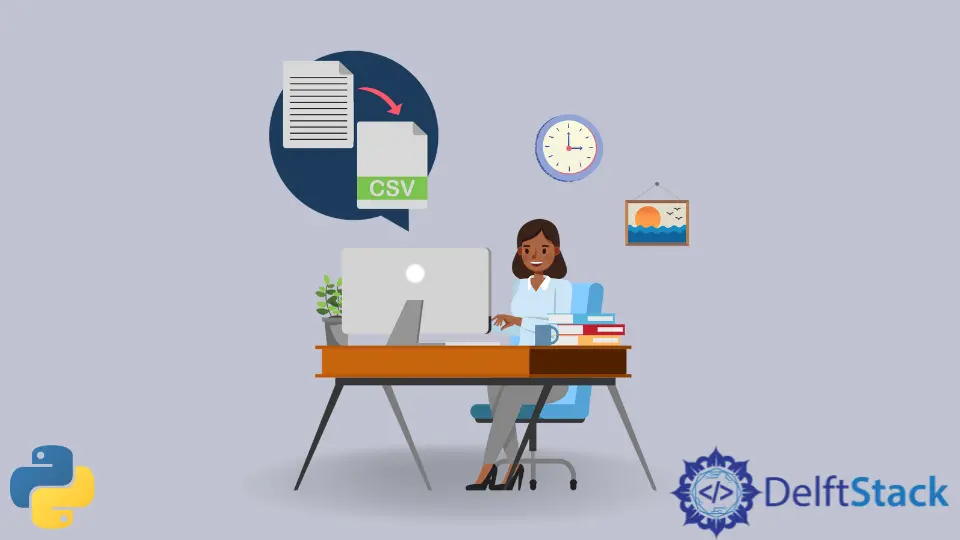
This tutorial will discuss how to write tabular data to a CSV file in Python.
Write Data to a CSV File With Traditional File Handling in Python
This method is reserved for when our data is already written in a comma-separated format, and we have to write it inside some file.
It isn’t a very pythonic way of handling CSV data, but it works. We have to open a CSV file in write mode and write our data into the file line by line. The following code snippet shows a working implementation of this method.
data = [["var112"], ["var234"], ["var356"]]
with open("csvfile.csv", "w") as file:
for line in data:
for cell in line:
file.write(cell)
file.write("\n")
CSV file:
We wrote a single column inside the csvfile.csv
with the file.write()
function in Python.
This method isn’t very user-friendly when it comes to handling CSV files. This very barebone method only works when our data is simple. As our data starts to grow and become increasingly complex to handle, this method becomes very difficult to use.
To save ourselves from the headache of dealing with different file handling problems, we must use the method discussed in the next section.
Write Data to a CSV File With the csv.writer()
Function in Python
We’ll use the csv
module in Python in this method.
The csv
module handles CSV files and data in Python. The writer()
function inside the csv
library takes the CSV file as an input parameter and returns a writer object responsible for converting user data into CSV format and writing it into the file.
We can write data into our CSV file with the writerows()
function inside this writer object. The following code snippet shows a working implementation of this approach.
import csv
data = [["var1", "val1", "val2"], ["var2", "val3", "val4"], ["var3", "val5", "val6"]]
with open("csvfile2.csv", "w") as file:
writer = csv.writer(file)
writer.writerows(data)
CSV file:
We wrote the values stored in the nested list data
to the csvfile2.csv
file. Each list in the data
corresponds to a unique row in the output file.
This method is preferred over our previous approach because the csv
module makes it easier to handle CSV data in Python. Note that we still need to use file handling with this method, but it is much easier than simply using the traditional file handling technique.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn