How to Split CSV Into Multiple Files in Python
Zeeshan Afridi
Feb 02, 2024
Python
Python CSV
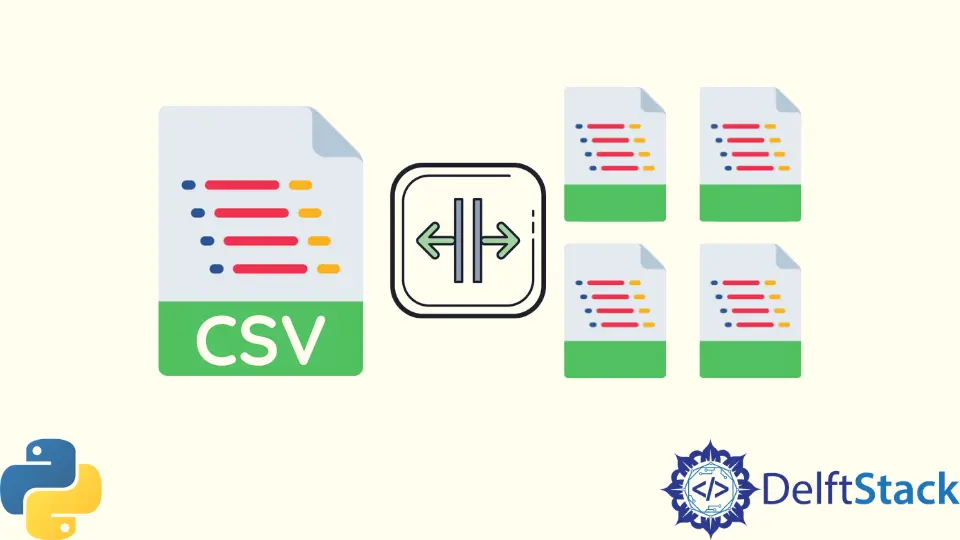
In this article, we will learn how to split a CSV file into multiple files in Python. We will use Pandas to create a CSV file and split it into multiple other files.
Create a CSV File in Python Using Pandas
To create a CSV in Python using Pandas, it is mandatory to first install Pandas through Command Line Interface (CLI).
pip install pandas
This command will download and install Pandas into your local machine. Using the import
keyword, you can easily import it into your current Python program.
Let’s verify Pandas if it is installed or not.
Code Example:
import pandas as pd
print("The Version of Pandas is: ", pd.__version__)
Output:
The Version of Pandas is: 1.3.5
Now, let’s create a CSV
file.
Code example:
import pandas as pd
# create a data set
data_dict = {
"Roll no": [1, 2, 3, 4, 5, 6, 7, 8],
"Gender": ["Male", "Female", "Female", "Male", "Male", "Female", "Male", "Female"],
"CGPA": [3.5, 3.3, 2.7, 3.8, 2.4, 2.1, 2.9, 3.9],
"English": [76, 77, 85, 91, 49, 86, 66, 98],
"Mathematics": [78, 87, 54, 65, 90, 59, 63, 89],
"Programming": [99, 45, 68, 85, 60, 39, 55, 88],
}
# create a data frame
data = pd.DataFrame(data_dict)
# convert the data frame into a csv file
data.to_csv("studesnts.csv")
# Print the output
print(data)