파이썬은 CSV를 여러 파일로 분할
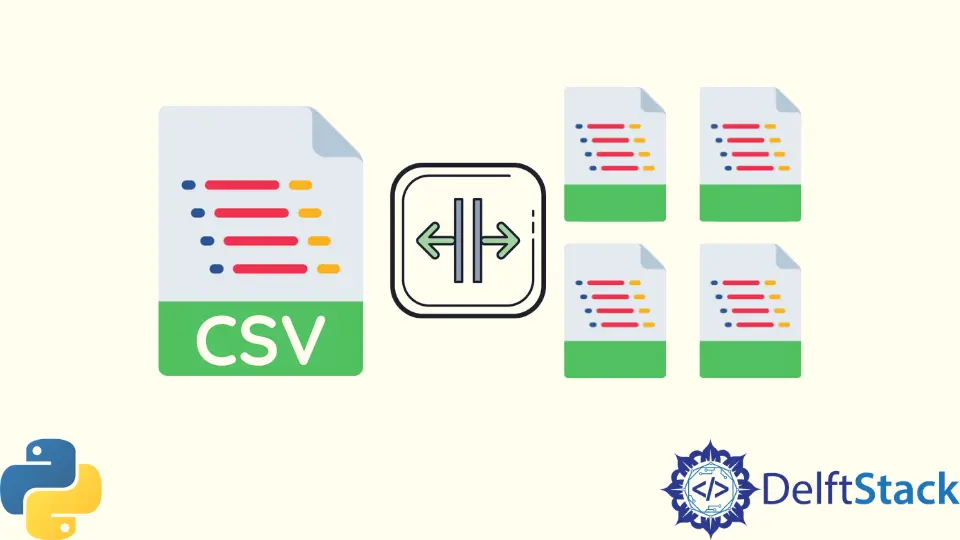
이 기사에서는 Python에서 CSV 파일을 여러 파일로 분할하는 방법을 배웁니다. Pandas를 사용하여 CSV 파일을 만들고 여러 다른 파일로 분할합니다.
Pandas를 사용하여 Python에서 CSV 파일 만들기
Pandas를 사용하여 Python에서 CSV를 생성하려면 먼저 명령줄 인터페이스(CLI)를 통해 Pandas를 설치해야 합니다.
pip install pandas
이 명령은 Pandas를 로컬 컴퓨터에 다운로드하여 설치합니다. import
키워드를 사용하여 현재 Python 프로그램으로 쉽게 가져올 수 있습니다.
Pandas가 설치되어 있는지 확인합시다.
코드 예:
import pandas as pd
print("The Version of Pandas is: ", pd.__version__)
출력:
The Version of Pandas is: 1.3.5
이제 CSV
파일을 만들어 보겠습니다.
코드 예:
import pandas as pd
# create a data set
data_dict = {
"Roll no": [1, 2, 3, 4, 5, 6, 7, 8],
"Gender": ["Male", "Female", "Female", "Male", "Male", "Female", "Male", "Female"],
"CGPA": [3.5, 3.3, 2.7, 3.8, 2.4, 2.1, 2.9, 3.9],
"English": [76, 77, 85, 91, 49, 86, 66, 98],
"Mathematics": [78, 87, 54, 65, 90, 59, 63, 89],
"Programming": [99, 45, 68, 85, 60, 39, 55, 88],
}
# create a data frame
data = pd.DataFrame(data_dict)
# convert the data frame into a csv file
data.to_csv("studesnts.csv")
# Print the output
print(data)
출력:
Roll no Gender CGPA English Mathematics Programming
0 1 Male 3.5 76 78 99
1 2 Female 3.3 77 87 45
2 3 Female 2.7 85 54 68
3 4 Male 3.8 91 65 85
4 5 Male 2.4 49 90 60
5 6 Female 2.1 86 59 39
6 7 Male 2.9 66 63 55
7 8 Female 3.9 98 89 88
Python에서 CSV 파일을 여러 파일로 분할
CSV
파일을 성공적으로 생성했습니다. 여러 파일로 분할해 보겠습니다. 그러나 다른 행렬을 사용하여 열 또는 행을 기준으로 CSV를 분할할 수 있습니다.
행을 기준으로 CSV 파일 분할
Python에서 행을 기준으로 CSV 파일을 분할해 보겠습니다.
코드 예:
import pandas as pd
# read DataFrame
data = pd.read_csv("students.csv")
# number of csv files along with the row
k = 2
size = 4
for i in range(k):
df = data[size * i : size * (i + 1)]
df.to_csv(f"students{i+1}.csv", index=False)
file1 = pd.read_csv("students1.csv")
print(file1)
print("\n")
file2 = pd.read_csv("students2.csv")
print(file2)
출력:
Roll no Gender CGPA English Mathematics Programming
0 1 Male 3.5 76 78 99
1 2 Female 3.3 77 87 45
2 3 Female 2.7 85 54 68
3 4 Male 3.8 91 65 85
Roll no Gender CGPA English Mathematics Programming
4 5 Male 2.4 49 90 60
5 6 Female 2.1 86 59 39
6 7 Male 2.9 66 63 55
7 8 Female 3.9 98 89 88
위의 코드는 students.csv
파일을 student1.csv
및 student2.csv
라는 두 개의 여러 파일로 분할했습니다. 파일은 행별로 구분됩니다. 행 0~3은 student.csv
에 저장되고 행 4~7은 student2.csv
파일에 저장됩니다.
열을 기준으로 CSV 파일 분할
groupby()
기능을 사용하여 열 행렬을 기반으로 CSV 파일을 분할할 수 있습니다. groupby()
함수는 Pandas 라이브러리에 속하며 그룹 데이터를 사용합니다.
이 경우 성별
을 기준으로 학생
데이터를 그룹화합니다.
코드 예:
import pandas as pd
# read DataFrame
data = pd.read_csv("students.csv")
for (gender), group in data.groupby(["Gender"]):
group.to_csv(f"{gender} students.csv", index=False)
print(pd.read_csv("Male students.csv"))
print("\n")
print(pd.read_csv("Female students.csv"))
출력:
Roll no Gender CGPA English Mathematics Programming
0 1 Male 3.5 76 78 99
1 4 Male 3.8 91 65 85
2 5 Male 2.4 49 90 60
3 7 Male 2.9 66 63 55
Roll no Gender CGPA English Mathematics Programming
0 2 Female 3.3 77 87 45
1 3 Female 2.7 85 54 68
2 6 Female 2.1 86 59 39
3 8 Female 3.9 98 89 88
결론
데이터 분할은 데이터를 이해하고 효율적으로 정렬하는 데 도움이 되는 유용한 데이터 분석 기술입니다.
이 기사에서는 Pandas 라이브러리를 사용하여 CSV 파일을 만드는 방법에 대해 설명했습니다. 또한 두 가지 일반적인 데이터 분할 기술인 행 방향 및 열 방향 데이터 분할에 대해 설명했습니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn