How to Write List to CSV Columns in Python
- Method 1: Using the CSV Module
- Method 2: Using Pandas Library
- Method 3: Writing to CSV with NumPy
- Conclusion
- FAQ
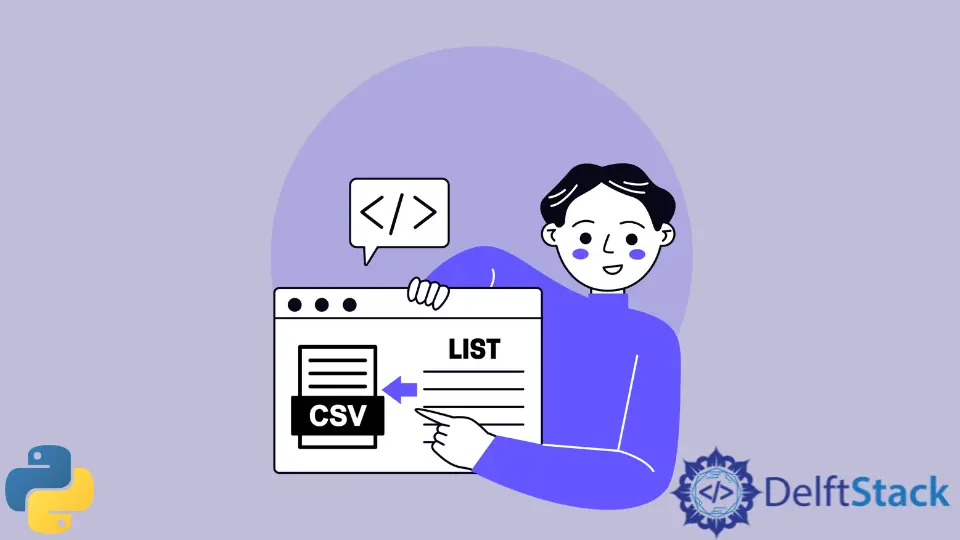
Writing data to CSV files is a common task in Python, especially when dealing with data analysis, data storage, or data sharing. If you have a list of data and want to write it to specific columns in a CSV file, you’re in the right place.
In this tutorial, we will explore various methods to accomplish this task seamlessly. Whether you’re a beginner or an experienced Python programmer, this guide will provide you with clear examples and explanations to help you understand how to write lists to CSV columns effectively. Let’s dive in and make your data management tasks easier!
Method 1: Using the CSV Module
The built-in csv
module in Python is a powerful tool for handling CSV files. It provides functionality to read from and write to CSV files with ease. To write a list to CSV columns, you can utilize the csv.writer
class. Here’s how you can do it:
import csv
data = [['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles'],
['Charlie', 35, 'Chicago']]
with open('output.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
Output:
The CSV file 'output.csv' will contain the following data:
Name,Age,City
Alice,30,New York
Bob,25,Los Angeles
Charlie,35,Chicago
In this example, we first import the csv
module. We then create a list of lists, where each inner list represents a row in the CSV file. Using open()
, we create a new file named output.csv
in write mode. The csv.writer
object is initialized, allowing us to call the writerows()
method to write our data to the file. Each inner list is written as a separate row, resulting in a well-structured CSV file.
Method 2: Using Pandas Library
If you’re dealing with larger datasets or prefer a more flexible approach, the pandas
library is an excellent choice. It provides powerful data manipulation capabilities, making it easy to write lists to CSV columns. Here’s an example:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [30, 25, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
df.to_csv('output_pandas.csv', index=False)
Output:
The CSV file 'output_pandas.csv' will contain the following data:
Name,Age,City
Alice,30,New York
Bob,25,Los Angeles
Charlie,35,Chicago
In this method, we first import the pandas
library. We create a dictionary where each key represents a column name and the associated values are lists of data. By passing this dictionary to pd.DataFrame()
, we create a DataFrame object. Finally, we use the to_csv()
method to write the DataFrame to a CSV file named output_pandas.csv
. The parameter index=False
ensures that the index column is not included in the output.
Method 3: Writing to CSV with NumPy
If you’re working with numerical data, the numpy
library can be very handy. It allows you to work with arrays and matrices efficiently, and it can also be used to write lists to CSV files. Here’s how you can do it:
import numpy as np
data = np.array([['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles'],
['Charlie', 35, 'Chicago']])
np.savetxt('output_numpy.csv', data, delimiter=',', fmt='%s')
Output:
The CSV file 'output_numpy.csv' will contain the following data:
Name,Age,City
Alice,30,New York
Bob,25,Los Angeles
Charlie,35,Chicago
In this example, we import the numpy
library and create a 2D array using np.array()
. This array contains our data structured in rows and columns. The np.savetxt()
function is then used to write the array to a CSV file named output_numpy.csv
. The delimiter=','
argument specifies that we want to separate values with commas, and fmt='%s'
ensures all data is treated as strings.
Conclusion
Writing lists to CSV columns in Python can be accomplished using various methods, each suited for different use cases. Whether you prefer the simplicity of the built-in csv
module, the advanced capabilities of pandas
, or the efficiency of numpy
, Python provides you with powerful tools to manage your data effectively. By following the methods outlined in this tutorial, you can easily create well-structured CSV files that meet your data storage and sharing needs.
FAQ
-
What is a CSV file?
A CSV (Comma-Separated Values) file is a simple text file that uses commas to separate values, making it easy to store tabular data. -
Do I need to install any libraries to write to CSV in Python?
No, the built-incsv
module is included with Python. However, for advanced data manipulation, you may want to installpandas
ornumpy
. -
Can I write a nested list to a CSV file?
Yes, you can write a nested list to a CSV file using thecsv.writer
or other libraries likepandas
andnumpy
. -
How can I read a CSV file in Python?
You can use thecsv.reader
from thecsv
module or theread_csv()
function from thepandas
library to read CSV files. -
Is it possible to append data to an existing CSV file?
Yes, you can open a CSV file in append mode (‘a’) and use thewriterow()
orwriterows()
methods to add new data.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python