Pandas DataFrame DataFrame.to_csv() Function
-
Syntax of
pandas.DataFrame.to_csv()
-
Example Codes:
DataFrame.to_csv()
-
Example Codes:
DataFrame.to_csv()
to Specify a Separator for CSV Data -
Example Codes:
DataFrame.to_csv()
to Select Few Columns and Rename the Columns
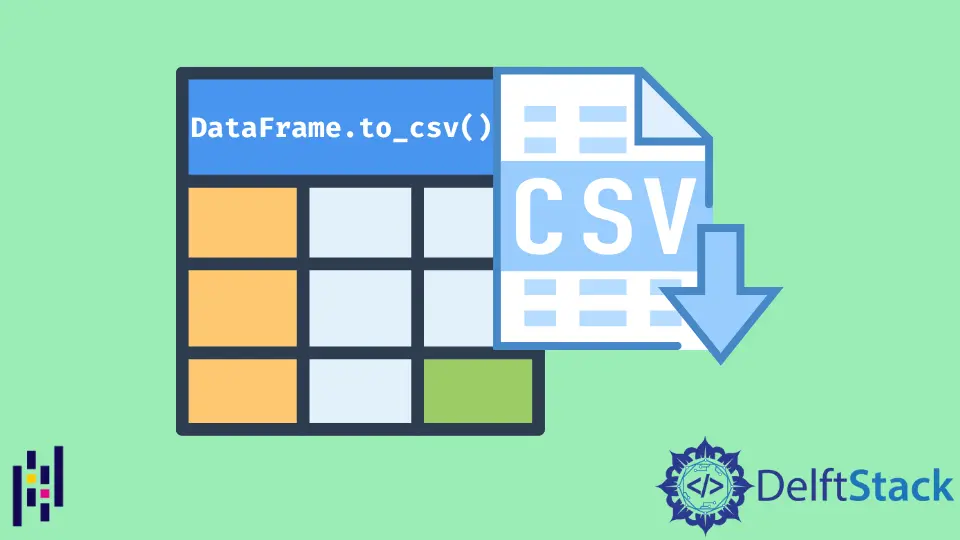
Python Pandas DataFrame.to_csv()
function saves the values contained in rows and columns of a DataFrame
into a CSV file. We can also convert a DataFrame
into a CSV string.
Syntax of pandas.DataFrame.to_csv()
DataFrame.to_csv(
path_or_buf=None,
sep=",",
na_rep="",
float_format=None,
columns=None,
header=True,
index=True,
index_label=None,
mode="w",
encoding=None,
compression="infer",
quoting=None,
quotechar='""',
line_terminator=None,
chunksize=None,
date_format=None,
doublequote=True,
escapechar=None,
decimal=".",
)
Parameters
This function has several parameters. The default values of all the parameters are mentioned above.
path_or_buf |
It is a string or a file handle. It represents the name of a file or a file object. If its value is None then the DataFrame is converted to a CSV string. |
sep |
It is a string. It represents the separator used in the CSV file. |
na_rep |
It is a string. It represents the missing data. |
float_format |
It is a string. It represents the format for the floating-point numbers. |
columns |
It is a sequence. It represents the columns of the DataFrame that will be saved in the CSV file. |
header |
It is a boolean value or a list of strings. If its value is set to False then the names of columns are not saved in the CSV file. If a list of strings is passed then these strings are saved as column names. |
index |
It is a boolean value. If its value is True then the names of rows i.e index are saved. |
index_label |
It is a string or a sequence. It represents the column name for a specific index. |
mode |
It is a string. It represents the mode of the process. As we are writing a DataFrame to a CSV file its value is Python write mode w . |
encoding |
It is a string. It represents the encoding scheme to use in the CSV file. The default encoding scheme is utf-8 . |
compression |
It is a string or a dictionary. If it is a string then it represents the compression mode. If it is a dictionary then the value at the method represents the compression mode. There are several compression modes. You can check here. |
quoting |
It represents a constant from a CSV module. |
quotechar |
It is a string. It has a length of 1. It represents the character used to quote fields. |
line_terminator |
It is a string. It represents the character for a new line in the CSV file. |
chunksize |
It is an integer. It represents the number of rows to write in the CSV file at a time. |
date_format |
It is a string. It represents the format for DateTime objects. |
doublequote |
It is a boolean value. It controls the quoting of quotechar . |
escapechar |
It is a string. It has a length of 1. It represents the character that is used to escape sep and quotechar . |
decimal |
It is a string. It represents the character used for a decimal point. |
Return
It returns None
or a string. If path_or_buf
is None then it converts the DataFrame
to a string and returns the string. Otherwise, it returns None
.
Example Codes: DataFrame.to_csv()
We will implement this function in different ways in the next few codes.
import pandas as pd
dataframe=pd.DataFrame({
'Attendance':
{0: 60,
1: 100,
2: 80,
3: 78,
4: 95},
'Name':
{0: 'Olivia',
1: 'John',
2: 'Laura',
3: 'Ben',
4: 'Kevin'},
'Obtained Marks':
{0: 90,
1: 75,
2: 82,
3: 64,
4: 45}
})
print(dataframe)
The example DataFrame
is,
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
All the parameters of this function are optional. If we execute this function without passing any parameter then it produces the following output.
import pandas as pd
dataframe=pd.DataFrame({
'Attendance':
{0: 60,
1: 100,
2: 80,
3: 78,
4: 95},
'Name':
{0: 'Olivia',
1: 'John',
2: 'Laura',
3: 'Ben',
4: 'Kevin'},
'Obtained Marks':
{0: 90,
1: 75,
2: 82,
3: 64,
4: 45}
})
csvstring = dataframe.to_csv()
print(csvstring)
Output:
,Attendance,Name,Obtained Marks
0,60,Olivia,90
1,100,John,75
2,80,Laura,82
3,78,Ben,64
4,95,Kevin,45
The function has produced the output using all the default values. It has returned a CSV string. Now we will save the data in the CSV file.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
returnValue = dataframe.to_csv("myfile.csv")
print(returnValue)
Output:
None
The function has created a new CSV file in the directory where this program is saved.
Example Codes: DataFrame.to_csv()
to Specify a Separator for CSV Data
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
returnValue = dataframe.to_csv(sep="@")
print(returnValue)
Output:
@Attendance@Name@Obtained Marks
0@60@Olivia@90
1@100@John@75
2@80@Laura@82
3@78@Ben@64
4@95@Kevin@45
Example Codes: DataFrame.to_csv()
to Select Few Columns and Rename the Columns
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
returnValue = dataframe.to_csv(
"myfile.csv", columns=["Name", "Obtained Marks"], header=["Full Name", "Marks"]
)
print(returnValue)
Output:
None
Just like the above codes, we can customize our CSV file using different parameters. This function provides several parameters to use.