Pandas DataFrame.resample() Function
Minahil Noor
Jan 30, 2023
-
Syntax of
pandas.DataFrame.resample()
: -
Example Codes:
DataFrame.resample()
Method to Resample the Data of Series on Weekly Basis -
Example Codes:
DataFrame.resample()
Method to Resample the Data of Series on Monthly Basis
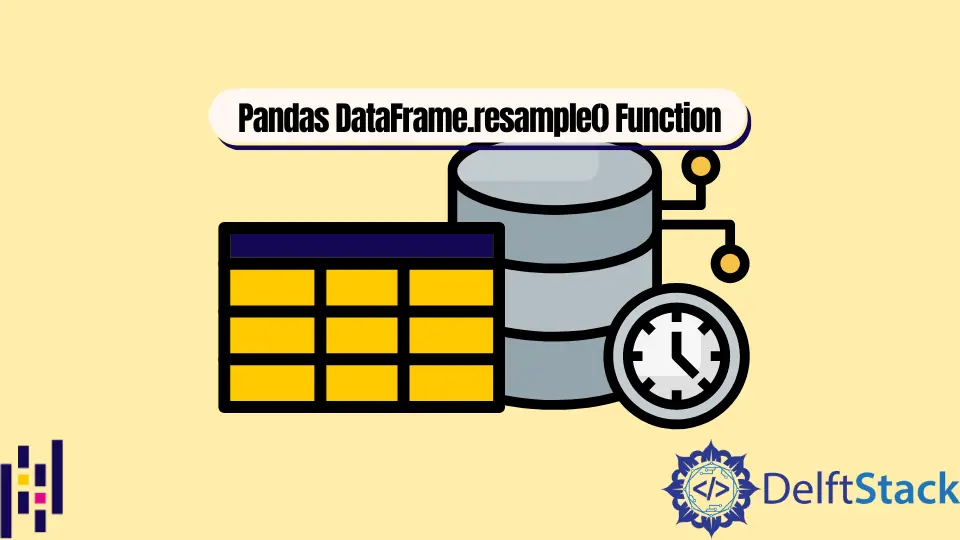
Python Pandas DataFrame.resample()
function resamples the time-series data.
Syntax of pandas.DataFrame.resample()
:
DataFrame.resample(
rule,
axis=0,
closed=None,
label=None,
convention="start",
kind=None,
loffset=None,
base=None,
on=None,
level=None,
origin="start_day",
offset=None,
)
Parameters
rule |
It is the offset string or object representing target conversion. |
axis |
It specifies which axis to use for up or down-sampling. For Series, this will default to 0, which means along the rows. |
closed |
It specifies which side of the bin interval is closed. It has two options: right or left . |
label |
It specifies bin edge label to label bucket with. It has two options: right or left . |
convention |
It has four options: start , end , s , or e . For PeriodIndex only, it uses the start or end of the rule. |
kind |
It specifies the kind of resulting index. It has two options: timestamp or period . Timestamp converts the resulting index to a DateTimeIndex, and period converts it to a PeriodIndex. |
loffset |
It adjusts the resampled time labels. |
base |
It is an integer. Its default value is 0. |
on |
It represents the name of the column to use instead of the index for resampling. The column must be datetime-like. |
level |
It represents the name of level to use for resampling. The level must be datetime-like. |
origin |
It is the timestamp on which to adjust the grouping. It has three options: epoch , start , or start_day . |
offset |
It represents an offset timedelta added to the origin parameter. |
Return
It returns the resampled object.
Example Codes: DataFrame.resample()
Method to Resample the Data of Series on Weekly Basis
import pandas as pd
index = pd.date_range('1/1/2021', periods=30, freq='D')
series = pd.Series(range(30), index=index)
print("The Original Series is: \n")
print(series)
series1= series.resample('W').sum()
print("The Resampled Data is: \n")
print(series1)
Output:
The Original Series is:
2021-01-01 0
2021-01-02 1
2021-01-03 2
2021-01-04 3
2021-01-05 4
2021-01-06 5
2021-01-07 6
2021-01-08 7
2021-01-09 8
2021-01-10 9
2021-01-11 10
2021-01-12 11
2021-01-13 12
2021-01-14 13
2021-01-15 14
2021-01-16 15
2021-01-17 16
2021-01-18 17
2021-01-19 18
2021-01-20 19
2021-01-21 20
2021-01-22 21
2021-01-23 22
2021-01-24 23
2021-01-25 24
2021-01-26 25
2021-01-27 26
2021-01-28 27
2021-01-29 28
2021-01-30 29
Freq: D, dtype: int64
The Resampled Data is:
2021-01-03 3
2021-01-10 42
2021-01-17 91
2021-01-24 140
2021-01-31 159
Freq: W-SUN, dtype: int64
The function has returned the resampled sum on a weekly basis.
Example Codes: DataFrame.resample()
Method to Resample the Data of Series on Monthly Basis
import pandas as pd
index = pd.date_range('1/1/2021', periods=30, freq='D')
series = pd.Series(range(30), index=index)
print("The Original Series is: \n")
print(series)
series1= series.resample('M').sum()
print("The Resampled Data is: \n")
print(series1)
Output:
The Original Series is:
2021-01-01 0
2021-01-02 1
2021-01-03 2
2021-01-04 3
2021-01-05 4
2021-01-06 5
2021-01-07 6
2021-01-08 7
2021-01-09 8
2021-01-10 9
2021-01-11 10
2021-01-12 11
2021-01-13 12
2021-01-14 13
2021-01-15 14
2021-01-16 15
2021-01-17 16
2021-01-18 17
2021-01-19 18
2021-01-20 19
2021-01-21 20
2021-01-22 21
2021-01-23 22
2021-01-24 23
2021-01-25 24
2021-01-26 25
2021-01-27 26
2021-01-28 27
2021-01-29 28
2021-01-30 29
Freq: D, dtype: int64
The Resampled Data is:
2021-01-31 435
Freq: M, dtype: int64
The function has returned the resampled sum on a monthly basis.