Pandas DataFrame sort_index() Function
-
pandas.DataFrame.sort_index()
Method -
Example: Sort a Pandas DataFrame Based on Index Using
sort_index()
Method -
Example: Sort Columns of a Pandas DataFrame Using
sort_index()
Method
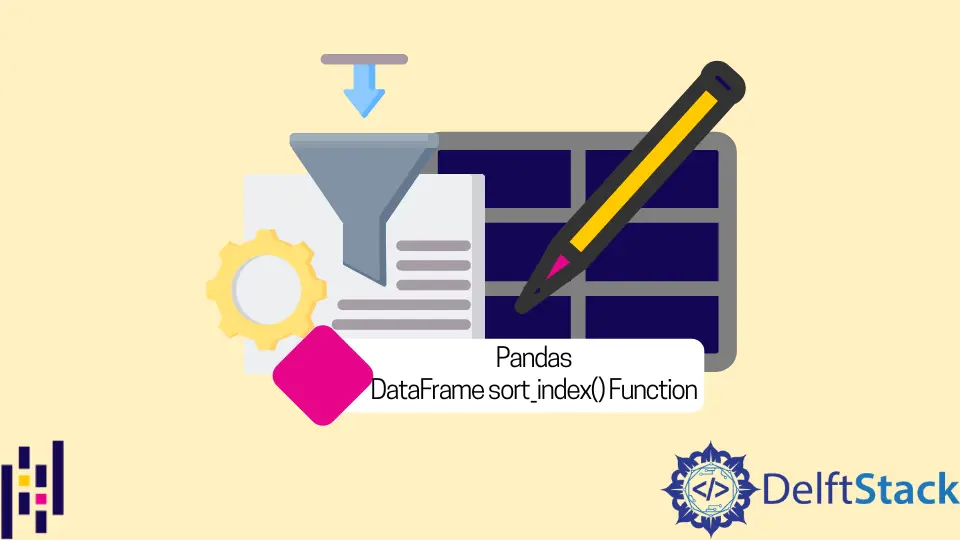
This tutorial explains how we can sort a Pandas DataFrame based on an index using the pandas.DataFrame.sort_index()
method.
We will use the DataFrame displayed in the above example to explain how we can sort a Pandas DataFrame based on index values.
import pandas as pd
pets_df = pd.DataFrame(
{
"Pet": ["Dog", "Cat", "Rabbit", "Fish"],
"Name": ["Rocky", "Luna", "Coco", "Finley"],
"Age(Years)": [3, 5, 5, 4],
},
index=["4", "2", "1", "3"],
)
print(pets_df)
Output:
Pet Name Age(Years)
4 Dog Rocky 3
2 Cat Luna 5
1 Rabbit Coco 5
3 Fish Finley 4
pandas.DataFrame.sort_index()
Method
Syntax
DataFrame.sort_index(axis=0,
level=None,
ascending=True,
inplace=False,
kind='quicksort',
na_position='last',
sort_remaining=True,
ignore_index=False
key=None)
Parameters
axis |
sort along the row (axis=0 ) or column (axis=1 ) |
level |
Int or List. Sort on values in specified index levels |
ascending |
sort in ascending order (ascending=True ) or descending order (ascending=False ) |
inplace |
Boolean. If True , modify the caller DataFrame in-place |
kind |
which sorting algorithm to use. default:quicksort |
na_position |
Put NaN value at the beginning (na_position = 'first' ) or the end (na_position = 'last' ) |
sort_remaining |
Boolean. If True , sort by other levels too (in order) after sorting by specified level for index=multilevel |
ignore_index |
Boolean. If True , the label of the resulting axis will be 0,1,…n-1. |
key |
Callable. If not None, apply this key function to the index values before sorting. |
Return
If inplace
is True
, returns the sorted DataFrame
by index along the specified axis; otherwise, None
.
By default, we have axis=0
, representing the DataFrame will be sorted along the row
axis or sorted by index values. If we set axis=1
, it will sort the columns of the DataFrame. By default, the method will sort the DataFrame in ascending order. To sort the DataFrame in descending order, we set ascending=False
.
Example: Sort a Pandas DataFrame Based on Index Using sort_index()
Method
import pandas as pd
pets_df = pd.DataFrame(
{
"Pet": ["Dog", "Cat", "Rabbit", "Fish"],
"Name": ["Rocky", "Luna", "Coco", "Finley"],
"Age(Years)": [3, 5, 5, 4],
},
index=["4", "2", "1", "3"],
)
sorted_df = pets_df.sort_index()
print("Initial DataFrame:")
print(pets_df, "\n")
print("DataFrame Sorted by Index Values:")
print(sorted_df)
Output:
Initial DataFrame:
Pet Name Age(Years)
4 Dog Rocky 3
2 Cat Luna 5
1 Rabbit Coco 5
3 Fish Finley 4
DataFrame Sorted by Index Values:
Pet Name Age(Years)
1 Rabbit Coco 5
2 Cat Luna 5
3 Fish Finley 4
4 Dog Rocky 3
It sorts the pet_df
DataFrame in ascending order based on the index values. To sort the DataFrame based on index values, we need to specify the index
parameter. By default, the value of axis
is 0
, which sorts the rows of the DataFrame i.e. sort DataFrame based on index values.
To sort the DataFrame based on index values in descending order, we set ascending=False
in the sort_index()
method.
import pandas as pd
pets_df = pd.DataFrame(
{
"Pet": ["Dog", "Cat", "Rabbit", "Fish"],
"Name": ["Rocky", "Luna", "Coco", "Finley"],
"Age(Years)": [3, 5, 5, 4],
},
index=["4", "2", "1", "3"],
)
sorted_df = pets_df.sort_index(ascending=False)
print("Initial DataFrame:")
print(pets_df, "\n")
print("DataFrame Sorted in Descending order based Index Values:")
print(sorted_df)
Output:
Initial DataFrame:
Pet Name Age(Years)
4 Dog Rocky 3
2 Cat Luna 5
1 Rabbit Coco 5
3 Fish Finley 4
DataFrame Sorted in Descending order based Index Values:
Pet Name Age(Years)
4 Dog Rocky 3
3 Fish Finley 4
2 Cat Luna 5
1 Rabbit Coco 5
It sorts the pets_df
DataFrame in descending order based on the index values.
Example: Sort Columns of a Pandas DataFrame Using sort_index()
Method
To sort the columns of a Pandas DataFrame, we set axis=1
in the sort_index()
method.
import pandas as pd
pets_df = pd.DataFrame(
{
"Pet": ["Dog", "Cat", "Rabbit", "Fish"],
"Name": ["Rocky", "Luna", "Coco", "Finley"],
"Age(Years)": [3, 5, 5, 4],
},
index=["4", "2", "1", "3"],
)
sorted_df = pets_df.sort_index(axis=1)
print("Initial DataFrame:")
print(pets_df, "\n")
print("DataFrame with sorted Columns:")
print(sorted_df)
Output:
Initial DataFrame:
Pet Name Age(Years)
4 Dog Rocky 3
2 Cat Luna 5
1 Rabbit Coco 5
3 Fish Finley 4
DataFrame with sorted Columns:
Age(Years) Name Pet
4 3 Rocky Dog
2 5 Luna Cat
1 5 Coco Rabbit
3 4 Finley Fish
It sorts the columns of the pets_df
DataFrame. The columns are sorted in ascending order by the name of columns.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn