How to Remove Spaces From a Variable Using PowerShell
-
Use the
Replace
Operator to Remove Spaces From a Variable Using PowerShell -
Use the
Replace()
Method to Remove Spaces From a Variable Using PowerShell -
Use the
Trim()
Method to Remove Spaces From a Variable Input Using PowerShell - Use Regular Expression to Remove Spaces From a Variable Using PowerShell
-
Use the
TrimEnd()
andTrimStart()
Methods to Remove Spaces From a Variable Using PowerShell - Conclusion
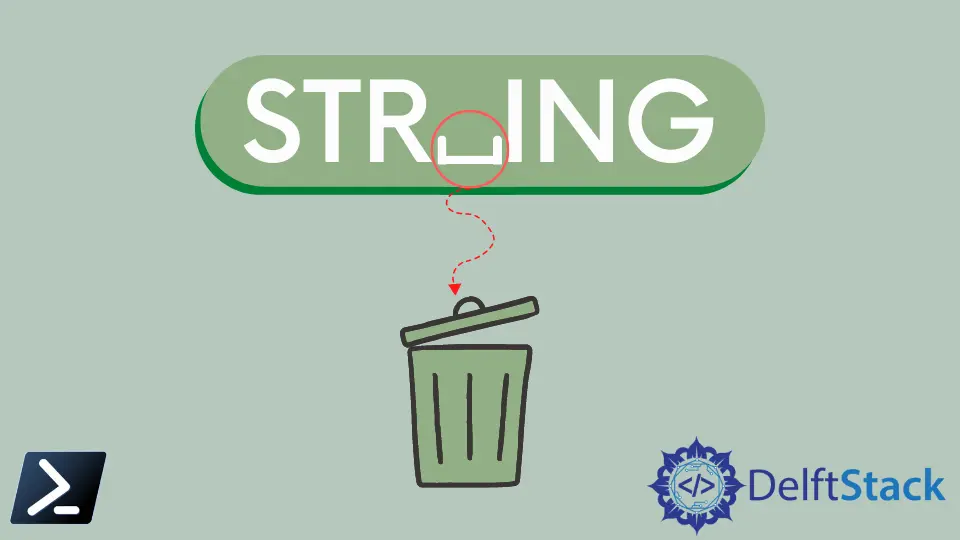
A string is one of the most common data types used in PowerShell. It contains the sequence of characters or texts.
You can perform different string operations in PowerShell, such as replacing strings, concatenating strings, splitting strings, comparing strings, and extracting substrings.
This tutorial will teach you to remove spaces from a variable by replacing them with an empty string in PowerShell.
Use the Replace
Operator to Remove Spaces From a Variable Using PowerShell
The -Replace
operator replaces texts or characters in PowerShell. You can also use it to remove texts or characters from the string.
The -Replace
operator takes two arguments: the string to find and the string to replace from the given input.
$string = "Power Shell"
$string -replace " ", ""
In this code, We start with a string variable named $string
initialized with the value "Power Shell"
.
Then, we use the -replace
operator in PowerShell. This operator is used for pattern-based replacements in strings.
In the pattern " "
, the space inside the double quotes represents the substring we want to replace. Essentially, we are searching for all occurrences of spaces in the $string
variable.
The replacement part of the -replace
operator is specified as an empty string ""
. This means we want to replace all occurrences of spaces with nothing, effectively removing them.
When we execute $string -replace " ",""
, we are performing the replacement operation on the string. All spaces in the string are replaced with an empty string.
Output:
PowerShell
In the above command, the -Replace
operator finds all space characters " "
and replaces them with an empty string ""
.
Use the Replace()
Method to Remove Spaces From a Variable Using PowerShell
The Replace()
method is similar to the -Replace
operator. It also takes two arguments: the string to find and the string to replace.
In the following example, the Replace()
method finds all space characters " "
and replaces them with an empty string ""
.
$string = "P o w e r S h e l l"
$string.replace(" ", "")
We start with a string variable named $string
initialized with the value "P o w e r S h e l l"
. In this string, there are spaces between each letter.
Next, we use the Replace()
method on the $string
variable. The Replace()
method is used to replace all occurrences of a specified substring with another substring.
In this case, we are replacing the space " "
with an empty string ""
. By doing so, we are effectively removing all the spaces within the string.
Output:
PowerShell
As we can see in the output, all the spaces were removed using the Replace()
Method.
Use the Trim()
Method to Remove Spaces From a Variable Input Using PowerShell
Another way to remove spaces from strings is the Trim()
method of the System.String
.NET class. It allows you to remove all whitespaces or unwanted characters from the front and end of strings.
For example, the following command trims the leading and trailing white space characters from the given string object.
$string = " PowerShell String "
$string.Trim()
In this code, we start with a string variable named $string
containing the value " PowerShell String "
. This value has leading and trailing spaces.
Next, we use the .Trim()
method on the $string
variable. The .Trim()
method is used to remove any leading and trailing whitespace (spaces, tabs, line breaks) from a string.
When the .Trim()
method is called, it scans the input string from both ends and removes any whitespace characters it encounters. In this case, it removes the leading and trailing spaces.
Output:
PowerShell String
The output is the value of $string
that is now a modified string without leading or trailing spaces.
Use Regular Expression to Remove Spaces From a Variable Using PowerShell
We can also use Regular Expressions (regex) to remove all spaces from a variable in PowerShell. Let’s give an example.
$string = " Power Shell Str ing "
$string = [regex]::Replace($string, '\s', '')
In this code, we start with the variable $string
, which contains spaces within and around the text. Then, we use [regex]::Replace
to perform a regex-based replacement on $string
.
Next, we specify the pattern '\s'
, which matches all whitespace characters, including spaces. Now, we replace all the matches with an empty string ''
, effectively removing all spaces.
Output:
PowerShellString
It shows in the output that the modified value of $string
becomes "PowerShellString"
, with all spaces removed.
Use the TrimEnd()
and TrimStart()
Methods to Remove Spaces From a Variable Using PowerShell
The TrimStart()
method removes characters from the beginning of the strings, whereas TrimEnd()
removes characters from the end of the strings.
$string = " Power Shell Str ing "
$string = $string.TrimStart().TrimEnd()
In this code, we first use the .TrimStart()
method to remove leading spaces and then the .TrimEnd()
method to remove trailing spaces. The result will be a string without any leading or trailing spaces.
Output:
Power Shell Str ing
Please note that this approach retains the spaces within the string and only removes the leading and trailing spaces. If you want to remove all spaces, you should use the regular expression method, as shown in the previous example.
Conclusion
In this article, we’ve explored various techniques to remove spaces from text in PowerShell. These methods provide us with the flexibility to handle spaces in different ways.
Whether we prefer simple operations like -Replace
, detailed control with Replace()
, the power of regular expressions, or easy trimming with Trim()
, these tools can help us tidy up our text. Depending on our specific requirements, we can choose the best method for efficiently manipulating strings and removing spaces.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell