How to Compare the Contents of Two String Objects in PowerShell
-
Compare String Objects in PowerShell Using the Equal Operator (
-eq
) -
Compare String Objects in PowerShell Using the
-like
Operator -
Compare String Objects in PowerShell Using the
Equals()
Method -
Compare String Objects in PowerShell Using the Not Equal Operator (
-ne
) - Conclusion
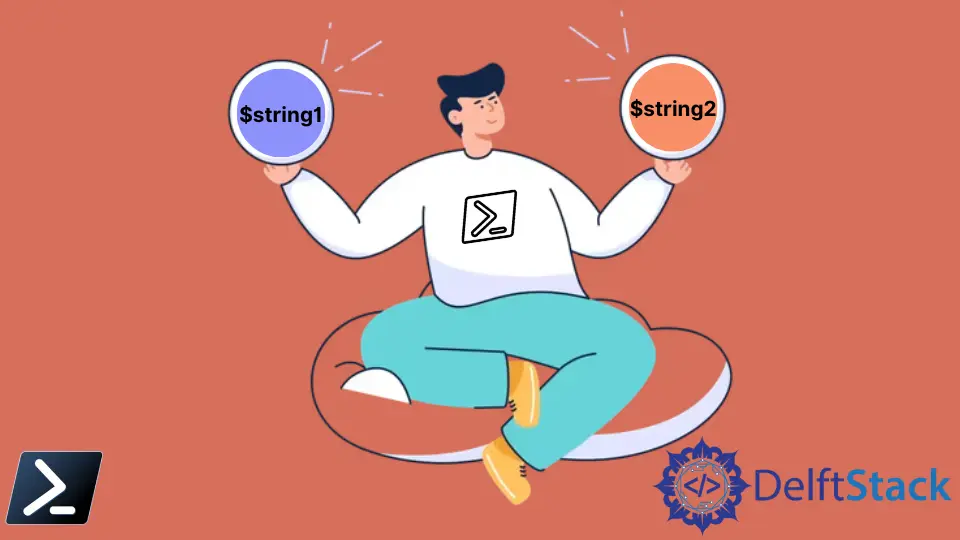
In PowerShell, effective string comparison is a fundamental skill that plays an important role in various scripting and automation tasks. Understanding the different methods and operators available for comparing the contents of two string objects is essential for PowerShell practitioners.
This article explores several approaches, from basic equality and inequality checks using operators like -eq
and -ne
to more advanced techniques involving the method Equals()
. By delving into these methods, you can tailor your string comparisons to meet the specific requirements of your scripts.
Compare String Objects in PowerShell Using the Equal Operator (-eq
)
In PowerShell, comparing the contents of two string objects can be achieved using the Equal operator (-eq
). This operator checks for the equality of two values, making it a straightforward and commonly used method for string comparisons.
The syntax for using the Equal operator is as follows:
$String1 -eq $String2
Here, $String1
and $String2
are the two string objects being compared.
The -eq
operator evaluates whether the contents of these two strings are identical. If the strings match, it returns True
; otherwise, it returns False
.
Let’s walk through the examples demonstrating the use of the Equal operator to compare string objects:
Example 1: Basic String Equality Check
$String1 = "Hello, PowerShell"
$String2 = "Hello, World"
# Compare the contents of the string objects
$Result = $String1 -eq $String2
Write-Host "Are the strings equal? $Result"
In this example, we start by defining two string objects, $String1
and $String2
. These strings contain different content for the purpose of demonstrating the comparison.
Next, we use the Equal operator (-eq
) to compare the contents of these two strings:
$Result = $String1 -eq $String2
The result of this comparison is stored in the variable $Result
. If the strings are equal, $Result
will be set to True
; otherwise, it will be set to False
. Finally, we output the result using Write-Host
:
Write-Host "Are the strings equal? $Result"
This line will display a message indicating whether the strings are equal or not.
Code Output:
Example 2: Case-Insensitive Comparison
It’s important to note that the -eq
operator is case-insensitive by default, treating uppercase and lowercase letters as equivalent. We’ll demonstrate that the -eq
operator is case-insensitive when comparing string objects in PowerShell:
$String1 = "PowerShell is Case-Insensitive"
$String2 = "powershell is case-insensitive"
# Compare the contents of the string objects
$Result = $String1 -eq $String2
Write-Host "Are the strings equal? $Result"
Here, we begin by defining two string objects, $String1
and $String2
, with different casing in their content. The key observation here is that the -eq
operator is case-insensitive, meaning it will treat uppercase and lowercase letters as equivalent during the comparison.
$Result = $String1 -eq $String2
The result of the comparison is stored in the variable $Result
. Since the -eq
operator is case-insensitive, it will consider the content of $String1
and $String2
as equal, resulting in $Result
being set to True
.
Code Output:
This output confirms that despite the difference in the casing between the two string objects, the -eq
operator treats them as equal. Keep this case insensitivity in mind when utilizing the -eq
operator for string comparisons in PowerShell.
Example 3: Case-Sensitive String Comparison
As we have shown above, the Equal operator is case-insensitive by default. To perform a case-sensitive comparison, we can use the -ceq
operator:
$FirstString = "PowerShell"
$SecondString = "powershell"
$Result = $FirstString -ceq $SecondString
Write-Host "Are the strings equal (case-sensitive)? $Result"
In this example, two strings, $FirstString
and $SecondString
, with varying cases, are compared using the -ceq
operator. Unlike the default behavior of the Equal operator, -ceq
ensures a case-sensitive comparison, providing an accurate evaluation of string equality.
Code Output:
Compare String Objects in PowerShell Using the -like
Operator
The -like
operator is another versatile tool for comparing the contents of two string objects. This operator is particularly useful for pattern matching, allowing you to find elements that match a specified pattern within strings.
The -like
operator finds elements that match or do not match a given pattern. When used for string comparison, it returns a Boolean value (True
or False
) based on whether the specified pattern is found in the string.
The syntax for using the -like
operator in string comparison is as follows:
$String1 -like $String2
Here, $String1
is the target string, and $String2
is the pattern to be matched.
Example 1: Basic String Matching
Let’s begin with a straightforward example where we use the -like
operator to check if one string contains another:
$MainString = "PowerShell is powerful"
$Pattern = "*Power*"
$Result = $MainString -like $Pattern
Write-Host "Does the string match the pattern? $Result"
In this example, we start by defining a variable $MainString
containing the text PowerShell is powerful
. Additionally, we establish a pattern variable $Pattern
with the value *Power*
.
The -like
operator is then applied to check whether the $MainString
contains the specified pattern. The result of this comparison is stored in the variable $Result
.
Finally, we use Write-Host
to display the outcome of the string matching.
Code Output:
Example 2: Pattern Matching with Wildcards
Explore a scenario where we compare a string against multiple patterns using the -like
operator:
$TargetString = "Files: Document1.txt, Image.png, Code.ps1"
$Pattern1 = "*Document*"
$Pattern2 = "*.png"
$Pattern3 = "*Code*"
$Result1 = $TargetString -like $Pattern1
$Result2 = $TargetString -like $Pattern2
$Result3 = $TargetString -like $Pattern3
Write-Host "Contains Document: $Result1"
Write-Host "Contains .png file: $Result2"
Write-Host "Contains Code: $Result3"
In this scenario, we explore a more complex use case of the -like
operator.
The $TargetString
variable is defined as Files: Document1.txt, Image.png, Code.ps1
. Subsequently, three pattern variables, $Pattern1
, $Pattern2
, and $Pattern3
, are established with values *Document*
, *.png
, and *Code*
, respectively.
The -like
operator is then applied to check whether each pattern is present in the $TargetString
. The results of these individual comparisons are stored in variables $Result1
, $Result2
, and $Result3
.
Finally, we use Write-Host
to display the outcomes.
Code Output:
Compare String Objects in PowerShell Using the Equals()
Method
The Equals()
method is another powerful tool for comparing the contents of two string objects.
This method determines whether the values in two objects are equal or not, unlike comparison operators like -eq
or -like
; the Equals()
method provides a more nuanced approach to string comparison.
The Equals()
method is invoked on a string object and takes another string as an argument. The syntax for using the Equals()
method in string comparison is as follows:
$String1.Equals($String2)
Here, $String1
and $String2
are the variables or expressions representing the strings to be compared.
Example: Case-Sensitive String Comparison
Let’s delve into a code example that showcases the usage of the Equals()
method for string comparison:
$String1 = "PowerShell"
$String2 = "powershell"
$Result = $String1.Equals($String2)
Write-Host "Are the strings equal? $Result"
In this example, we have two string variables, $String1
and $String2
, with different cases. The Equals()
method is then applied to $String1
with $String2
as the argument.
The result of this comparison is stored in the variable $Result
. Finally, we use Write-Host
to display the outcome of the string comparison.
Code Output:
The Equals()
method in PowerShell offers a versatile approach to string comparison, allowing for more nuanced evaluations. Its capability to consider factors like case sensitivity makes it a valuable tool in scenarios where precise string matching is required.
Compare String Objects in PowerShell Using the Not Equal Operator (-ne
)
In PowerShell, the Not Equal operator (-ne
) is a straightforward and commonly used tool for comparing the contents of two string objects. This operator checks if the values of two expressions are not equal and returns a Boolean result.
The syntax for using the Not Equal operator (-ne
) in string comparison is as follows:
$String1 -ne $String2
Here, $String1
and $String2
are the variables or expressions representing the strings to be compared.
Let’s delve into complete code examples that showcase the usage of the Not Equal operator (-ne
) for string comparison:
Example 1: Basic Not Equal Check
$FirstString = "Hello"
$SecondString = "World"
if ($FirstString -ne $SecondString) {
Write-Host "Strings are not equal"
}
else {
Write-Host "Strings are equal"
}
In this example, we have two string variables, $FirstString
and $SecondString
, with distinct values.
The -ne
operator is then used to check if the strings are not equal. Based on the result of the comparison, either Strings are not equal
or Strings are equal
is displayed using Write-Host
.
Code Output:
The output confirms that the strings Hello
and World
are not equal.
Example 2: Case-Sensitive Not Equal Check
$String1 = "PowerShell"
$String2 = "powershell"
if ($String1 -cne $String2) {
Write-Host "Strings are not equal (case-sensitive)"
}
else {
Write-Host "Strings are equal (case-sensitive)"
}
In this additional example, the -cne
operator is used to perform a case-sensitive not equal check between two string variables, $String1
and $String2
. The result is displayed using Write-Host
, indicating whether the strings are equal or not with consideration for case sensitivity.
Code Output:
The output reflects that the strings PowerShell
and powershell
are not equal when case sensitivity is considered.
Conclusion
Comparing the contents of two string objects in PowerShell involves employing various methods and operators, each tailored to specific use cases. Whether using simple operators like -eq
and -ne
for equality and inequality checks or the Equals()
method for nuanced comparisons, PowerShell offers a versatile toolkit.
The choice of method depends on the specific requirements of the comparison, such as case sensitivity, pattern complexity, or the need for detailed result information. By understanding these options, users can navigate diverse string comparison scenarios with precision and efficiency in PowerShell.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell