How to Extract a Substring in PowerShell
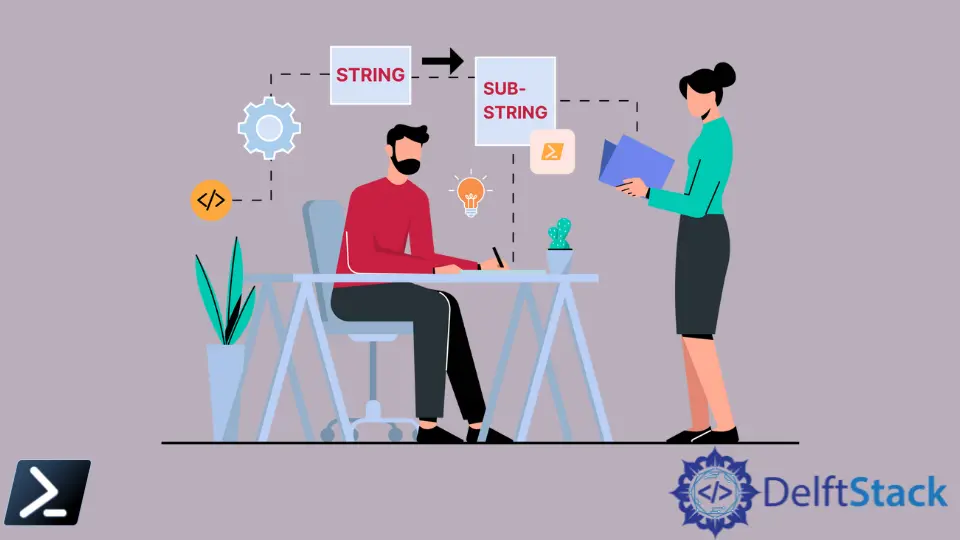
Extracting substrings in PowerShell is a common task that can help you manipulate and analyze strings effectively. Whether you’re working with file names, logs, or any other text data, knowing how to extract specific portions of strings can save you time and effort.
In this article, we’ll explore various methods to efficiently extract substrings using PowerShell’s powerful string library. You’ll learn about different techniques, including using the Substring
method, regular expressions, and string manipulation functions, all tailored for practical use cases. So, let’s dive into the world of PowerShell substrings and discover how to make your string handling tasks smoother and more efficient.
Using the Substring Method
One of the most straightforward ways to extract a substring in PowerShell is by using the Substring
method. This method allows you to specify the starting index and the length of the substring you want to extract. Here’s how it works:
$string = "PowerShell is a powerful scripting language"
$substring = $string.Substring(0, 10)
$substring
Output:
PowerShell
In this example, we first define a string that contains a sentence. The Substring
method is called on the $string
variable, with 0
as the starting index and 10
as the length of the substring to extract. The result is “PowerShell,” which is the first ten characters of the original string.
The Substring
method is particularly useful when you know the exact position and length of the substring you want. However, if you need to extract substrings based on specific patterns or conditions, other methods may be more suitable.
Using Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and can be very useful when extracting substrings in PowerShell. By utilizing the -match
operator or the [regex]::Match()
method, you can target specific patterns in your strings. Here’s an example:
$string = "Email: user@example.com"
$pattern = "(\w+)@(\w+)\.(\w+)"
if ($string -match $pattern) {
$substring = $matches[0]
}
$substring
Output:
user@example.com
In this example, we define a string that contains an email address. The regex pattern (\w+)@(\w+)\.(\w+)
is used to match the email format. The -match
operator checks if the string fits the pattern, and if it does, the matched substring is stored in the $matches
automatic variable. The first match, which is the entire email address, is then assigned to the $substring
variable.
Using regex is particularly advantageous when dealing with complex strings where the position of the substring might vary. This method allows for greater flexibility and precision in extracting the desired information.
Using Split Method
Another effective way to extract substrings in PowerShell is by using the Split
method. This method divides a string into an array based on specified delimiters, allowing you to access individual segments easily. Here’s how it works:
$string = "apple,banana,cherry"
$array = $string.Split(',')
$substring = $array[1]
$substring
Output:
banana
In this example, we have a string containing fruit names separated by commas. The Split
method is called with a comma as the delimiter, resulting in an array of fruit names. We then access the second element in the array (index 1), which is “banana,” and assign it to the $substring
variable.
The Split
method is particularly useful when dealing with strings that have consistent delimiters. It allows you to easily break down complex strings into manageable parts, making it simple to extract the substrings you need.
Conclusion
In conclusion, extracting substrings in PowerShell can be accomplished using various methods, each suited for different scenarios. Whether you choose the straightforward Substring
method, the powerful regex capabilities, or the convenient Split
method, PowerShell provides you with the tools to manipulate strings effectively. By mastering these techniques, you can enhance your scripting skills and streamline your workflow when handling string data.
FAQ
-
What is a substring in PowerShell?
A substring is a portion of a string that can be extracted based on specific criteria, such as starting index and length. -
Can I use regular expressions in PowerShell?
Yes, PowerShell supports regular expressions, allowing you to match and extract substrings based on complex patterns. -
What is the difference between the Substring method and the Split method?
TheSubstring
method extracts a specific portion of a string based on index and length, while theSplit
method divides a string into an array based on delimiters.
-
How can I extract a substring based on a specific character?
You can use theSplit
method to separate the string by the specific character and then access the resulting array’s elements. -
Is it possible to extract multiple substrings at once?
Yes, you can use methods likeSplit
to create an array of substrings or use regex to capture multiple matches from a string.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell