How to Concatenate Strings in PowerShell
-
Using the
+
Operator to Concatenate Strings in PowerShell - Using Separators to Concatenate Strings in PowerShell
- Concatenating Strings With Integers in PowerShell
- Use String Substitution to Concatenate Strings in PowerShell
-
Using the
-f
Operator to Concatenate Strings in PowerShell -
Using the
-join
Operator to Concatenate Strings in PowerShell -
Using the String Builder and
append()
Function to Concatenate Strings in PowerShell -
Using the
concat()
Function to Concatenate Strings in PowerShell
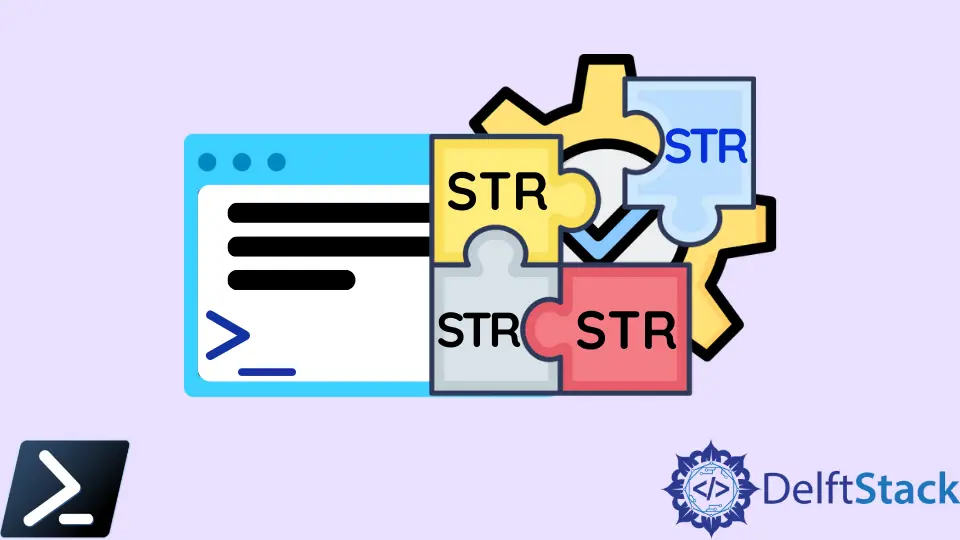
In PowerShell, we achieve string concatenation in multiple ways; however, while PowerShell has its own built-in concat()
function (which we will also discuss later on), there are more straightforward ways to join various strings variables.
Before we enumerate all of the string concatenation methods, it is better to declare some string variables that we will use as examples.
$str1 = "First"
$str2 = "Second"
$str3 = "Third"
Below are all the possible methods to concatenate your strings in PowerShell.
Using the +
Operator to Concatenate Strings in PowerShell
The most basic way of concatenating strings is by using the +
operator. Concatenation only works with the +
operator if both variables are strings variables. The script will process the expression mathematically if the +
operator is used on two or more integers type variables.
Example code:
$concatString = $str1 + $str2 + $str3
Write-Output $concatString
Output:
FirstSecondThird
Using Separators to Concatenate Strings in PowerShell
It is not only the +
operator that we must use to concatenate strings, but we can also use separators like commas (,
). Remember to enclose your string variables with double quotes ""
, or the variable will interpret your separated string values as a list property.
Example code:
$concatString = "$str1 , $str2 , $str3"
Write-Output $concatString
Output:
First , Second , Third
Also, remember that this doesn’t only work with commas. Enclosing your string valuables with double quotes makes your string variable a literal expression. WYSIWYG (What you see is what you get.)
Example code:
$concatString = "Who came in $str1 , $str2 , and $str3 Place?"
Write-Output $concatString
Output:
Who came in First , Second, and Third Place?
Concatenating Strings With Integers in PowerShell
If we try to concatenate strings with integers, an invalid type error will occur. In the example below, the new variable $concatString
will take $int1
variable’s type as its data type, which is an integer. This happens because $int1
is called first in the expression $int1 + $str1
.
Example code:
$int1 = 0
$concatString = $int1 + $str1 #int variable before string variable
Write-Output $concatString
Output:
Cannot convert value "First" to type "System.Int32". Error: "Input string was not in a correct format."
At line:3 char:1
+ $concatString = $int1 + $str1
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : InvalidArgument: (:) [], RuntimeException
+ FullyQualifiedErrorId : InvalidCastFromStringToInteger
If we take the string variable $str1
and place it first on the expression, the concatenation will be successful, and $int1
will be converted automatically into a string value.
Example code:
$int1 = 0
$concatString = $str1 + $int1 #str variable before int variable
Write-Output $concatString
Output:
First0
Use String Substitution to Concatenate Strings in PowerShell
Alternatively, we can perform another way of concatenation using string substitution. This method will also work when concatenating strings with different data types.
Example code:
$int1 = 0
$concatString = "$($int1) , $($str2)"
Write-Output $concatString
Output:
0 , Second
Using the -f
Operator to Concatenate Strings in PowerShell
Another way of concatenating strings is by using the -f
operator. The -f
operator makes use of passing string variables as arguments on a pre-built string value.
Example code:
$concatString = "{0}.{1}.{2}." -f $str1, $str2, $str3
Write-Output $concatString
Output:
First.Second.Third
Using the -join
Operator to Concatenate Strings in PowerShell
The -join operators acts similarly with separators, as we need to pass in a string separator as a parameter.
Example code:
$concatString = $str1, $str2, $str3 -join "!"
Write-Output $concatString
Output:
First!Second!Third
Using the String Builder and append()
Function to Concatenate Strings in PowerShell
The string builder is one of the complex ways of concatenating strings, as we need to call a separate object type System.Text.StringBuilder
to initiate this process. The object then uses the append()
function to concatenate the strings then converts the new object back to a string value using the ToString()
function.
Example code:
$concatString = New-Object -TypeName System.Text.StringBuilder
$null = $concatString.Append($str1)
$null = $concatString.Append($str2)
$null = $concatString.Append($str3)
$concatString = $concatString.ToString()
Write-Output $concatString
Output:
FirstSecondThird
Using the concat()
Function to Concatenate Strings in PowerShell
Lastly, we have PowerShell’s own concat()
function.
Example code:
$concatString = [System.String]::Concat($str1, ".", $str2, ".", $str3)
Write-Output $concatString
Output:
First.Second.Third
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell