How to Replace Strings in PowerShell
- Introduction to PowerShell Strings
-
Use the
replace()
Function in PowerShell -
Remove Characters Using the
replace()
Function in PowerShell -
Replace Multiple Instance Strings Using the
replace()
Function in PowerShell - Use the PowerShell Replace Operator
- Remove Characters Using the Replace Operator in PowerShell
- Replace Multiple Instance Strings Using the Replace Operator in PowerShell
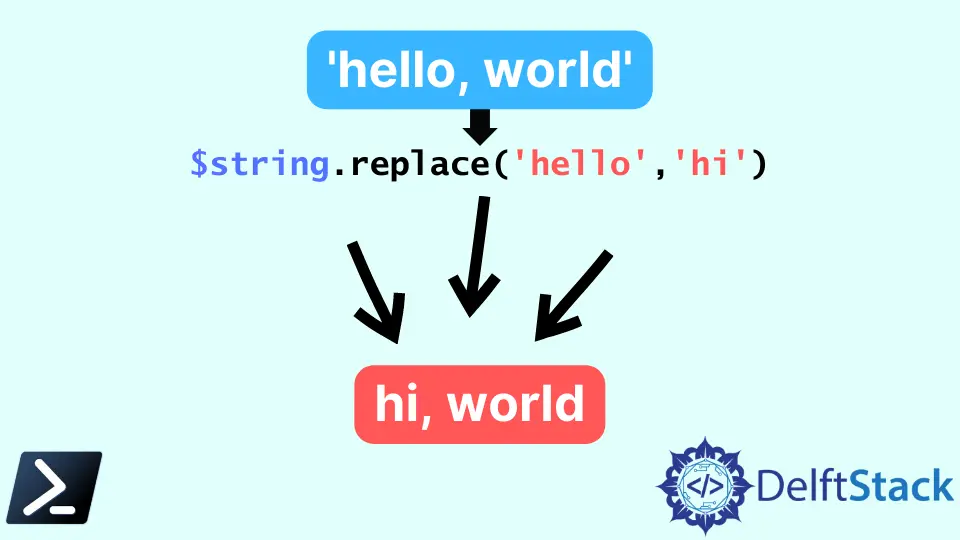
PowerShell can work with strings and text like many languages. One of those valuable features is using PowerShell to replace characters, strings, or text inside files.
This article will discuss the PowerShell replace()
method and PowerShell replace operator.
Introduction to PowerShell Strings
One of the most straightforward cases of using PowerShell is to replace characters in strings. Say we have a PowerShell string with a hello, world
value.
Example Code:
$string = 'hello, world'
We want to replace the string hello
inside that variable with the string hi
to change the $string
variable to have a final value of hi, world
.
To do this, PowerShell first needs to figure out where to find the specific text to replace, and once it’s located, it replaces the text with a custom user-defined variable value.
Use the replace()
Function in PowerShell
One of the convenient ways to replace strings in Windows PowerShell is to use the replace()
function, as shown below. The replace()
function has two arguments, the string to find and the string to replace the found text.
As we can see below, Windows PowerShell finds the string hello
and replaces that string with the string hi
. The method then returns the final result, hi, world
.
Example Code:
$string.replace('hello', 'hi')
Output:
hi, world
We can call the replace()
method on any string to replace any literal string with another. The replace()
method returns nothing if the string-to-be-replaced isn’t found.
It is worth noting that we don’t need to assign a string to a variable to replace text in a string. Instead, we can invoke the replace()
method directly on the string like: 'hello world'.replace('hello','hi')
.
Remove Characters Using the replace()
Function in PowerShell
Maybe we want to remove characters in a string from another string rather than replacing it with something else. We can do that too by specifying an empty string.
Example Code:
$string.replace('hello', '')
Output:
, world
Replace Multiple Instance Strings Using the replace()
Function in PowerShell
Since the replace()
function returns a string, to replace another instance, you can append another replace()
function call to the end. Windows PowerShell then invokes the replace()
method on the original output.
Example Code:
$string.replace('hello', '').replace('world', 'globe')
Output:
, globe
We can chain together as many replace()
function calls as necessary, but we should consider using the replace operator if we have many strings to replace.
Use the PowerShell Replace Operator
We can also use the Windows PowerShell -replace
operator to replace a text. The -replace
operator has similar to the method.
We provide a string to find and replace. Using the above example, we can use the replace operator to replace hello with hi similarly, as shown below.
Example Code:
$string -replace 'hello', 'hi'
Output:
hi, world
Remove Characters Using the Replace Operator in PowerShell
Like the replace()
function, you can also remove characters from a string using the replace operator. But, unlike the replace()
method, you can also wholly exclude the string as an argument to replace with, and you’ll discover the same effect.
Example Code:
$string -replace 'hello', ''
Output:
, world
Replace Multiple Instance Strings Using the Replace Operator in PowerShell
Like the replace()
method, you can also chain together usages of the replace operator. However, since the replace operator returns a string, as shown below.
Example Code:
$string -replace 'hello', 'hi' -replace 'world', 'globe'
Output:
hi, globe
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell