How to Split String Into Array in Bash
-
Using the
read
Command to Split a String Into an Array in Bash -
Using the
tr
Command to Split a String Into an Array in Bash - Using Parameter Expansion to Split a String Into an Array in Bash
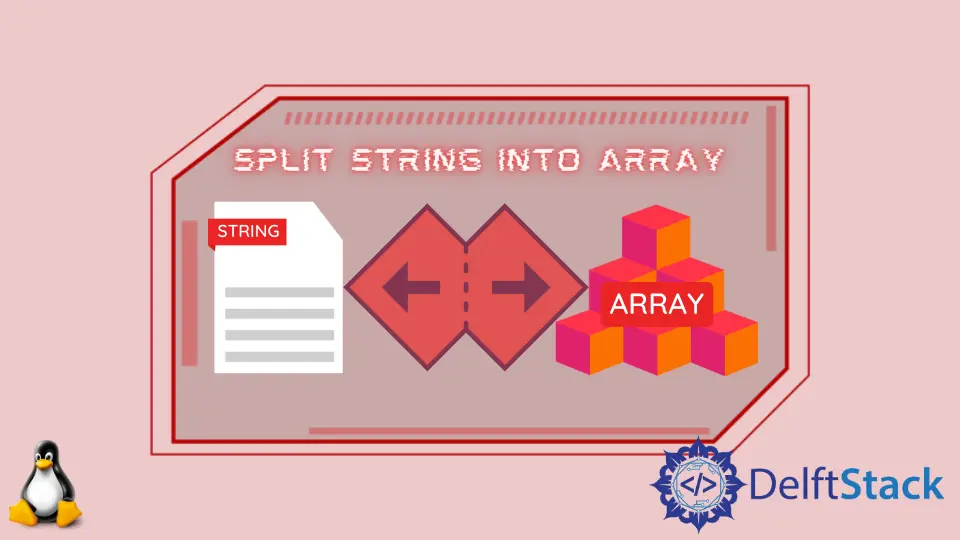
This tutorial explains splitting a string into an array in bash using the read
command, the tr
command, and parameter expansion.
Using the read
Command to Split a String Into an Array in Bash
The read
command is a built-in command on Linux systems.
It is used to read the content of a line into a variable. It also splits words of a string that is assigned to a shell variable.
In the script below, the variable $addrs
string is passed to the read
command. The IFS
sets the delimiter that acts as a word boundary on the string variable.
This means the -
is the word boundary in our case. The -a
option tells the read
command to store the words that have been split into an array, while the -r
option tells the read
command to treat any escape characters as they are and not interpret them.
The words that have been split are stored into the ip_array
variable. To access the individual elements inside the array, we use this syntax, ${array name[index]}
.
In the script below, ip_array
is the array name, and 0
is the index denoting the first element in the array.
#!/usr/bin/env bash
addrs="192.168.8.1,192.168.8.2,192.168.8.3,192.168.8.4"
IFS=',' read -ra ip_array <<< "$addrs"
printf "${ip_array[0]}\n"
The output displays the first element in the array.
192.168.8.1
The script below adds a for
loop iterating through the array and prints all the elements using the echo
command.
#!/usr/bin/env bash
addrs="192.168.8.1,192.168.8.2,192.168.8.3,192.168.8.4"
IFS=',' read -r -a ip_array <<< "$addrs"
for ip in "${ip_array[@]}"
do
echo "$ip"
done
The output below displays all the elements that are inside the array.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
Using the tr
Command to Split a String Into an Array in Bash
The tr
command is a short form for translate
.
It translates, deletes, and squeezes characters from the standard input and writes the results to the standard output. It is a useful command for manipulating text on the command line or in a bash script.
It can be used to remove repeated characters, convert lowercase to uppercase, and replace characters.
In the bash script below, the echo
command pipes the string variable, $addrs
, to the tr
command, which splits the string variable on a delimiter, ;
. Once the string has been split, the values are assigned to the ip_addrs
array.
The for
loop iterates through the $ip_addrs
array and prints out all the values using the printf
command.
#!/bin/bash
addrs="192.168.8.1,192.168.8.2,192.168.8.3,192.168.8.4"
ip_addrs=(`echo $addrs | tr ',' ' '`)
for ip in "${ip_addrs[@]}"
do
printf "$ip\n"
done
The output below displays all the elements that are inside the array.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
Using Parameter Expansion to Split a String Into an Array in Bash
The script below uses parameter expansion to search and replace characters.
The syntax used for for parameter expansion is ${variable//search/replace}
. It searches for a pattern that matches search
in the variable
and replaces it with the replace
.
In our case, the script searches for the pattern ,
and replaces it with a white space in the $addrs
string variable. The parenthesis around ${addrs//-/ }
are used to define an array of the new string, called ip_array
.
We use the for
loop to iterate over all the elements of the ip_array
and display them using the echo
command.
#!/bin/bash
addrs="192.168.8.1,192.168.8.2,192.168.8.3,192.168.8.4"
set -f
ip_array=(${addrs//,/ })
for ip in "${!ip_array[@]}"
do
echo "[$ip] ${ip_array[ip]}"
done
The output below displays all the elements that are inside the array.
[0] 192.168.8.1
[1] 192.168.8.2
[2] 192.168.8.3
[3] 192.168.8.4
Related Article - Bash String
- How to Remove First Character From String in Bash
- How to Remove Newline From String in Bash
- How to Split String Into Variables in Bash
- How to Get Length of String in Bash
- How to Convert String to Integer in Bash
- String Comparison Operator in Bash