How to Append New Data to an Array Without Specifying the Index in Bash
- Declare an Array in Bash
-
Use the
+=
Operator to Append Data to an Array Without Specifying the Index in Bash - Alternative Way to Append Data to an Array Without Specifying the Index in Bash
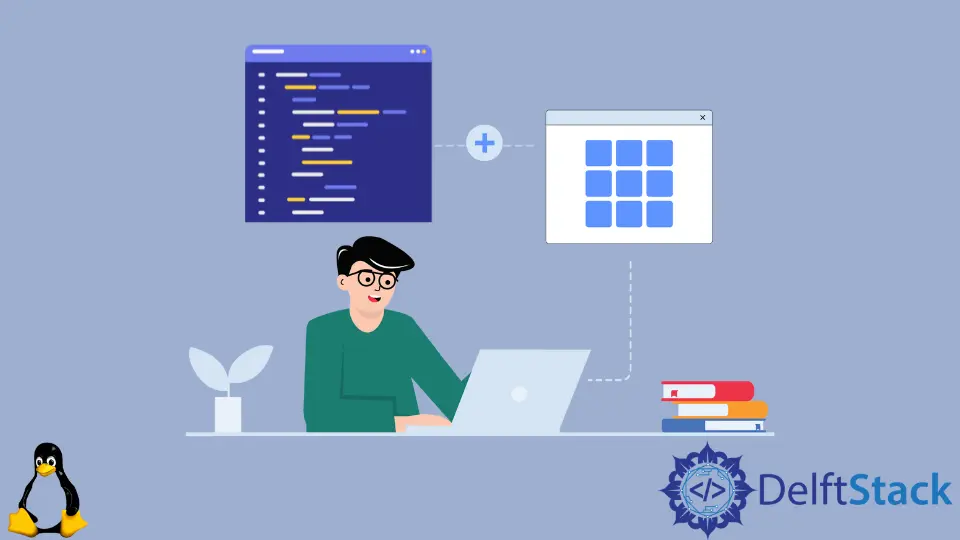
The array is the most common part of any programming language. In a Bash script, you also can work with the array; you can declare, modify, and operate on the array.
But in this article, we will see how we can declare an array and append new data to it step by step. We will see two different ways of appending new data in the array.
Also, we will see necessary examples and explanations to make the topic easier.
Declare an Array in Bash
This is the first step. In this step, we will see how to declare an array in Bash.
The general syntax to declare an array is:
ArrayName=('Data1' 'Data2' 'Data3')
Below, we declared an empty array, and the code for this is:
MyArray=()
Use the +=
Operator to Append Data to an Array Without Specifying the Index in Bash
We are done creating an array, so now we will input some data. This section will show how we can append data to an array without indexing.
The general syntax for this purpose is:
ArrayName+=('Your Data')
In the below example, we will include three data inside our array. The code for our example is:
MyArray=()
MyArray+=('A')
MyArray+=('B')
MyArray+=('C')
echo "Current array elements are: ${MyArray[@]}"
In the example above, we first declare an array and then include data in the array one by one. Lastly, we just displayed all the data in an array.
Now, after executing the above example of code, you will get an output like the one below:
Current array elements are: A B C
Alternative Way to Append Data to an Array Without Specifying the Index in Bash
In this method, we will see another alternative way to include data in an array without indexing. In the below example, we will include some data in our array without indexing.
The code for our example will look like the one below:
MyArray=('A' 'B' 'C')
MyArray=(${MyArray[@]} 'D')
MyArray=(${MyArray[@]} 'E')
MyArray=(${MyArray[@]} 'F' 'G')
echo "Current array elements are: ${MyArray[@]}"
In the example, we declared an array with some data by the line MyArray=('A' 'B' 'C')
. After that, we included all the data one by one.
Please note that in the part ${MyArray[@]}
, we attached all the previous data with the new one. This is the method where we repeatedly declare the array and update the data.
Now after executing the above example of code. You will get the output below:
Current array elements are: A B C D E F G
The two methods discussed above are the most reliable way to append data into an array without indexing.
Note: All the codes used in this article are written in Bash. It will only be runnable in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn