Bash Associative Array
- Bash Associative Array
- Access the Associative Array
- Add New Members to the Associative Array
- Delete a Member From the Associative Array
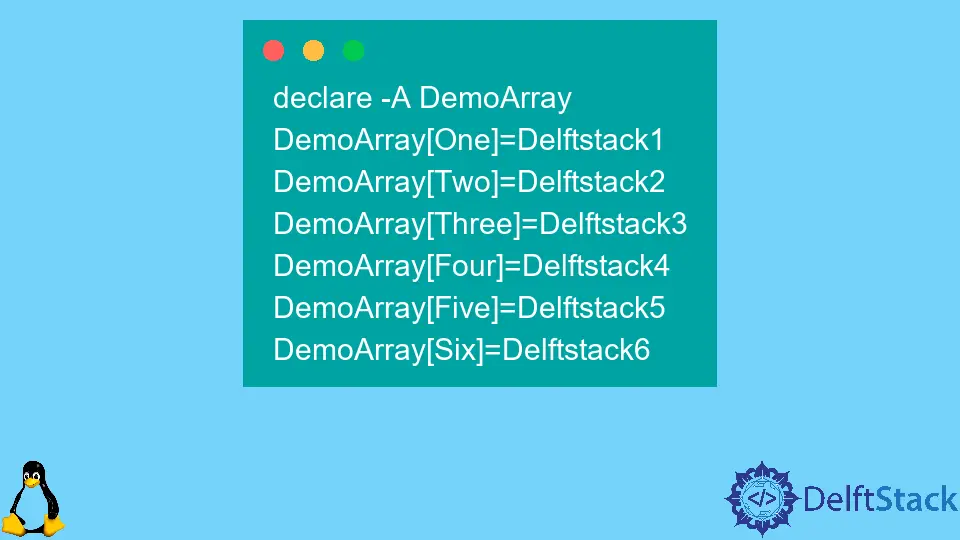
This tutorial demonstrates how to create an associative array in Bash.
Bash Associative Array
Arrays can be very usable in Bash to store data, and the array with a string index can also be created in Bash, which is called an associative array. The associative array was added in Bash 4 and cannot be declared in the version before that.
First, check the version of Bash because if the version is less than 4, then the associative array cannot be declared. We use the declare
command to declare an associative array.
Follow the steps below to declare an associative array:
-
First, check the version of Bash, and run the following command:
bash --version
The above command will check the version of Bash. The output is:
GNU bash, version 5.0.17(1)-release (x86_64-pc-linux-gnu) Copyright (C) 2019 Free Software Foundation, Inc. License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
-
Now use the
declare
command to declare an associative array:declare -A DemoArray DemoArray[One]=Delftstack1 DemoArray[Two]=Delftstack2 DemoArray[Three]=Delftstack3 DemoArray[Four]=Delftstack4 DemoArray[Five]=Delftstack5 DemoArray[Six]=Delftstack6
The above commands will declare an associative array first and then assign indexes and values.
-
Also, there is another command where we can declare an associative array in one line:
declare -A DemoArray1=( [One]=Delftstack1 [Two]=Delftstack2 [Three]=Delftstack3 )
The above command will create an associative array in one line.
Access the Associative Array
We can either access the elements of the associative array individually or by using an array. We can directly echo them or put the array into a loop.
See the commands to access the values individually:
echo ${DemoArray[One]}
echo ${DemoArray[Two]}
echo ${DemoArray[Three]}
echo ${DemoArray[Four]}
echo ${DemoArray[Five]}
echo ${DemoArray[Six]}
The above command will print the values of the associative array on given indexes. See the output:
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Delftstack6
We can also access all values of an associative array using a loop. See the command:
for ArrayKey in "${!DemoArray[@]}"; do echo $ArrayKey; done
echo "${!DemoArray[@]}"
Here !
can be used to read the keys of an associative array. The first command will print the keys of the associative array one by one, and the second command will print all the keys at once.
See the output:
Four
Six
One
Five
Two
Three Four Six One Five Two
Similarly, to print the value, we will delete the !
operator from the above commands:
for ArrayValue in "${DemoArray[@]}"; do echo $ArrayValue; done
echo "${DemoArray[@]}"
Now, these commands will print all the values of the given associative array. See the output:
Delftstack4
Delftstack6
Delftstack1
Delftstack5
Delftstack2
Delftstack3 Delftstack4 Delftstack6 Delftstack1 Delftstack5 Delftstack2
Finally, we can print the keys with value using the for
loop. See the command:
for ArrayKey in "${!DemoArray[@]}"; do echo "$ArrayKey => ${DemoArray[$ArrayKey]}"; done
The above command prints all the key-value pairs of the given array. See the output:
Three => Delftstack3
Four => Delftstack4
Six => Delftstack6
One => Delftstack1
Five => Delftstack5
Two => Delftstack2
Add New Members to the Associative Array
Adding a new element to an associative array is a very easy operation. We use the +=
concatenation operator between the array and the new key-value pair.
We create a key-value pair and then assign it to our associative array. See the command:
echo "${DemoArray[@]}"
DemoArray+=([Seven]=Delftstack7)
echo "${DemoArray[@]}"
The above command will print the original array, add a new member, and then print the modified array. See the output
Delftstack3 Delftstack4 Delftstack6 Delftstack1 Delftstack5 Delftstack2
Delftstack3 Delftstack4 Delftstack7 Delftstack6 Delftstack1 Delftstack5 Delftstack2
Delete a Member From the Associative Array
Deleting a member of an associative array is also an easy operation. We use the unset
command on the array member, which will be deleted from the array.
See the commands.
echo "${DemoArray[@]}"
unset DemoArray[Seven]
echo "${DemoArray[@]}"
The above command will first display the original array, then delete the member [Seven]
of the array, then again display the modified array. See the output:
Delftstack3 Delftstack4 Delftstack7 Delftstack6 Delftstack1 Delftstack5 Delftstack2
Delftstack3 Delftstack4 Delftstack6 Delftstack1 Delftstack5 Delftstack2
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook