How to Return an Array in Bash
- Command Substitution
- Use Command Substitution to Return an Array in Bash
-
Use
IFS
to Return an Array in Bash
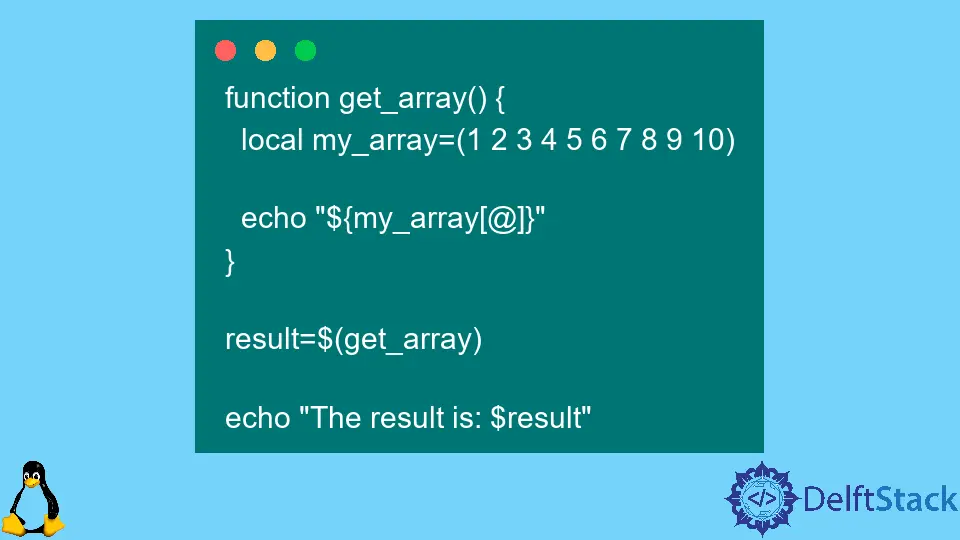
Since Bash does not handle arrays, returning an array can be a little hard. However, it is feasible to get past this restriction by utilizing a global variable or a command substitution workaround to return an array from a function.
This article will cover command substitution, a method for returning an array in bash without needing global variables.
Command Substitution
To understand how this works, we first need to understand what command substitution is and how it works in Bash.
The Bash feature called command substitution enables the output of a command to be used in place of the command itself. This is done by enclosing the command in a pair of backticks (``) or $()
, like this:
echo "The current date is: `date`"
Output:
The current date is: Fri Nov 29 16:14:23 PST 2022
The date
command is executed in the example above, and its output is substituted in place of the command itself. This allows us to embed the output of a command directly in a string, which can be useful in many situations.
Use Command Substitution to Return an Array in Bash
Now, let’s see how we can use command substitution to return an array from a function in Bash.
First, let’s create a function called get_array
that returns an array of numbers from 1 to 10 and uses command substitution. Then, call the function and store the output in a variable - result
- which we will print.
function get_array() {
local my_array=(1 2 3 4 5 6 7 8 9 10)
echo "${my_array[@]}"
}
result=$(get_array)
echo "The result is: $result"
Output:
The result is: 1 2 3 4 5 6 7 8 9 10
In the function above, we create an array called my_array
that contains the numbers from 1 to 10. We then use echo
to print the array and enclose it in quotes to ensure that the array elements are preserved as a single string. Afterward, we call the get_array
function and store its output in the result
variable. We then print the contents of the result
variable, which should be the array of numbers from 1 to 10.
Use IFS
to Return an Array in Bash
Let’s now see how we can get the array’s elements. Since the array elements are returned as a single string, we need to use the IFS
(Internal Field Separator) variable to split the string into individual elements.
The IFS
variable controls how bash splits a string into words or tokens. By default, IFS
is set to space
, tab
, and newline
, which means that bash will split a string into words at any of these characters.
To split the string returned by the get_array
function into individual elements, we need to set IFS
to a space character:
IFS=' '
result=$(get_array)
result_array=($result)
for i in "${!result_array[@]}"; do
echo "Element $i: ${result_array[$i]}"
done
Output:
Element 0: 1
Element 1: 2
Element 2: 3
Element 3: 4
Element 4: 5
Element 5: 6
Element 6: 7
Element 7: 8
Element 8: 9
Element 9: 10
In the code above, we set IFS
to a space character, which allows us to split the string returned by the get_array
function into individual elements. We then store the result in the result_array
variable and use a for
loop to print the elements of the array.
Let’s now look at how this method can return an array of strings rather than an array of numbers.
First, let’s modify the get_array
function to return an array of strings:
function get_array() {
local my_array=("one" "two" "three" "four" "five" "six" "seven" "eight" "nine" "ten")
# Return the array using the command substitution
echo "${my_array[@]}"
}
In the function above, we create an array called my_array
that contains the strings one
to ten
. We then use echo
to print the array and enclose it in quotes to ensure that the array elements are preserved as a single string.
Let’s now examine how we can utilize the get_array
function to return the array of strings:
IFS=' '
result=$(get_array)
result_array=($result)
for i in "${!result_array[@]}"; do
echo "Element $i: ${result_array[$i]}"
done
Output:
Element 0: one
Element 1: two
Element 2: three
Element 3: four
Element 4: five
Element 5: six
Element 6: seven
Element 7: eight
Element 8: nine
Element 9: ten
In the code above, we call the get_array
function and store its output in the result
variable. We then split the string into individual elements and store them in the result_array
variable.
Finally, we use a for
loop to print the elements of the array.
In conclusion, returning an array in bash can be tricky, as bash does not support arrays. However, a method known as command substitution can be used to have a function.
This involves enclosing the array in quotes and using echo
to print it and then splitting the resulting string into individual elements using the IFS
variable.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn