How to Print Array Elements in Bash
-
Use the
echo
Statement to Print Array Elements in Bash -
Use the
for
Loop to Print Array Elements in Separate Lines in Bash -
Use the
history
Keyword to Print Array Elements in Separate Lines in Bash -
Use the
basename
Keyword to Print Array Elements in Separate Lines in Bash -
Use the
shuf
Keyword to Print Array Elements in Separate Lines in Bash
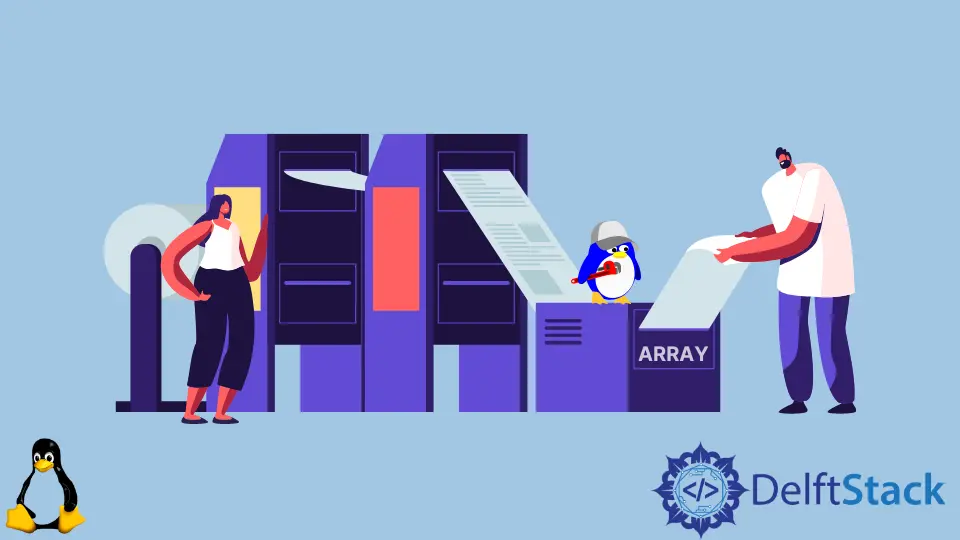
When working with an array, you may need to see what data is stored in the array. In a Bash script, there are several methods that we can use to print the array elements.
This article will show how we can print the array elements in Bash scripting. We are going to discuss 5 different methods for this purpose.
Also, we will see necessary examples and explanations to make the topic easier.
Use the echo
Statement to Print Array Elements in Bash
In this method, we will print all the elements of an array using the echo
keyword. However, this keyword will print all the array elements in a single line.
You can follow the below example code for this purpose:
MyArray=('A' 'B' 'C' 'D' 'E')
echo "The array elements are: ${MyArray[@]}"
After executing the above Bash script, you will get an output like the below,
The array elements are: A B C D E
Use the for
Loop to Print Array Elements in Separate Lines in Bash
In the below example, we will print the elements of an array using a loop. You can use any of the loops you prefer, but we will use the for
loop in our example.
The code for our example is shown below:
MyArray=('A' 'B' 'C' 'D' 'E')
for item in "${MyArray[@]}"
do
echo "$item"
done
In the example above, you can observe a part of the code that is ${MyArray[@]}
. This is for taking all the elements of the array in a loop.
After executing the above Bash script, you will get an output like the one below:
A
B
C
D
E
Use the history
Keyword to Print Array Elements in Separate Lines in Bash
Our next method will use the keyword history
to print the elements of an array. But please note that this method may fail if any array element contains a !
.
In the example below, we will use the history
keyword for printing the array elements. The code for our example will look like the following:
MyArray=('A' 'B' 'C' 'D' 'E')
echo "The array elements are: "
history -p "${MyArray[@]}"
After executing the above Bash script, you will get the output below:
The array elements are:
A
B
C
D
E
Use the basename
Keyword to Print Array Elements in Separate Lines in Bash
We can also print all the elements of an array using the keyword basename
. But please note that this method may fail if any array element contains a !
.
In our example below, we will use the basename
keyword for printing the array elements. The code for our example is given below:
MyArray=('A' 'B' 'C' 'D' 'E')
echo "The array elements are: "
basename -a "${MyArray[@]}"
After executing the above Bash script, you will get the below output:
The array elements are:
A
B
C
D
E
Use the shuf
Keyword to Print Array Elements in Separate Lines in Bash
There is another built-in keyword in Bash that we can use for the same purpose of printing the array elements, which is shuf
. But please note that this method may not provide the output in order.
In our example below, we will use the shuf
keyword for printing the array elements. The code for our example will look like the following:
MyArray=('A' 'B' 'C' 'D' 'E')
echo "The array elements are: "
shuf -e "${MyArray[@]}"
After executing the above Bash script, you will get the following output:
The array elements are:
B
A
C
E
D
You can choose any one of the above methods based on your needs.
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn