How to Pass an Array to a Function in Bash
- Functions in Bash Script
- Call a Function in Bash
- Function Arguments in Bash
- Pass an Array to a Function in Bash
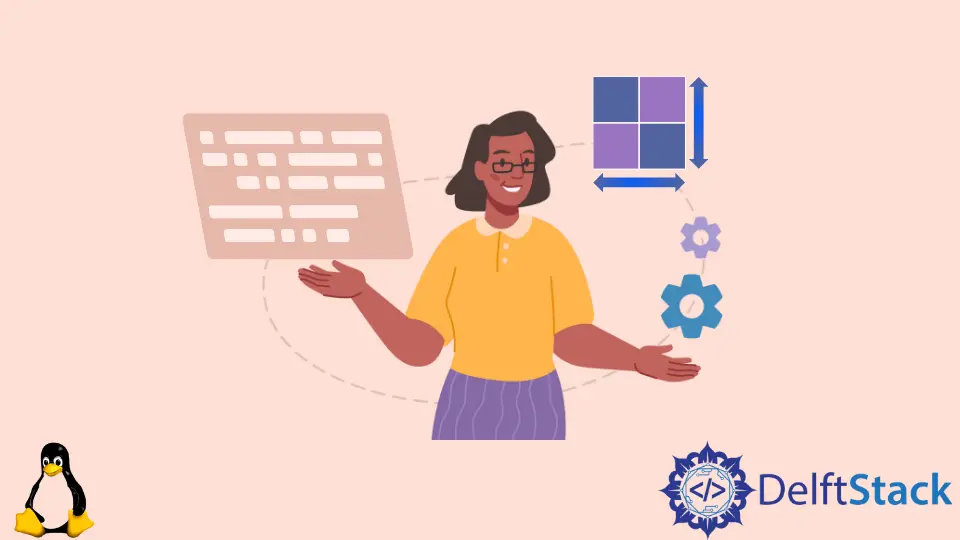
This small programming tutorial is about using functions in Bash scripts and passing arrays to them. Before moving toward the actual topic, we will briefly introduce Bash scripting.
A Bash script file contains a series of Bash commands. These commands are a combination of the ones we frequently type on the command line (like ls
or cp
) and the ones we could type on the command line.
Any command that can be entered and executed on the command line will perform the same action if it is included in a script file and runs.
Functions in Bash Script
In shell scripts, reusable code blocks are grouped using Bash functions. Most programming languages support this feature, which is also referred to by other names like procedures, methods, or subroutines.
A method for storing reusable code sections under one name is called a Bash function. There are two advantages to using functions when writing Bash scripts:
- Direct reading of a function into the shell’s memory allows it to be saved for later use. Today’s computers have plenty of memory, so using functions is quicker than repeatedly writing the same code.
- Long shell scripts can be broken up into reusable, modular code blocks with the aid of functions. The chunks are simpler to create and keep up with.
Declare a Function in Bash
There are two ways to declare a function in Bash:
-
One way to declare a function is by using the name of the function only like this:
<function_name> () { <set of commands> }
-
Another way is by using the
function
keyword like this:function <function_name> { < set of commands> }
For both types of declaration, we can also use a one-line declaration like this:
function <function_name> { <set of commands>; }
When using functions, you must keep the following facts in mind:
- Whether using Bash scripts or the terminal directly, commands written in a single line must end with a semicolon (
;
). - Parentheses are optional when the
function
reserved word is added. - The function’s body comprises the commands that appear between curly braces or
. Any number of declarations, variables, loops, or conditional statements are permitted in the body. - Try to give descriptive function names. Descriptive names are helpful when other developers look at the code, though they are not required when testing functions and commands.
Call a Function in Bash
To call a function, we use its name. Make sure that the function is declared before it is called.
Let’s look at the code below:
#!/bin/Bash
func1 () {
echo Hello from function
echo Good Bye!
}
func1
This code will give the following output:
Function Arguments in Bash
The parameters should be added after the function call and separated by spaces if you want to pass arguments to a function. Working with Bash function arguments has several options listed in the table below.
Argument | Purpose |
---|---|
$0 |
When a function is defined in the terminal, it saves its name. When specified in a Bash script, $0 outputs the script’s name and location. |
$1 , $2 , etc. |
This corresponds to the position of the argument following the function name. |
$# |
This tells the count of the total no. of arguments passed. |
$@ and $* |
This saves the array or list type of arguments passed. |
"$@" |
It breaks the list passed into separate arguments, for example, "$1", "$2" , etc. |
Pass an Array to a Function in Bash
Consider an example below in which we will pass an array list to the function, and the function will break it into separate variables and print them on the screen.
#!/bin/Bash
function printArray() {
a=("$@")
for b in "${a[@]}";
do
echo "$b"
done
}
array=("first" "second" "third")
printArray "${array[@]}
Note that we passed the arguments as a list enclosed in parenthesis when we called the function. This will make them an array, and the function will save them in $@
.
We then printed that array in a loop.
Output: