Functions in Bash
- Functions in Bash
- Defining Functions in Bash
- Sample Functions
- Variable Scope in Bash Function
- Return Values in Bash Function
- Passing Arguments to Bash Functions
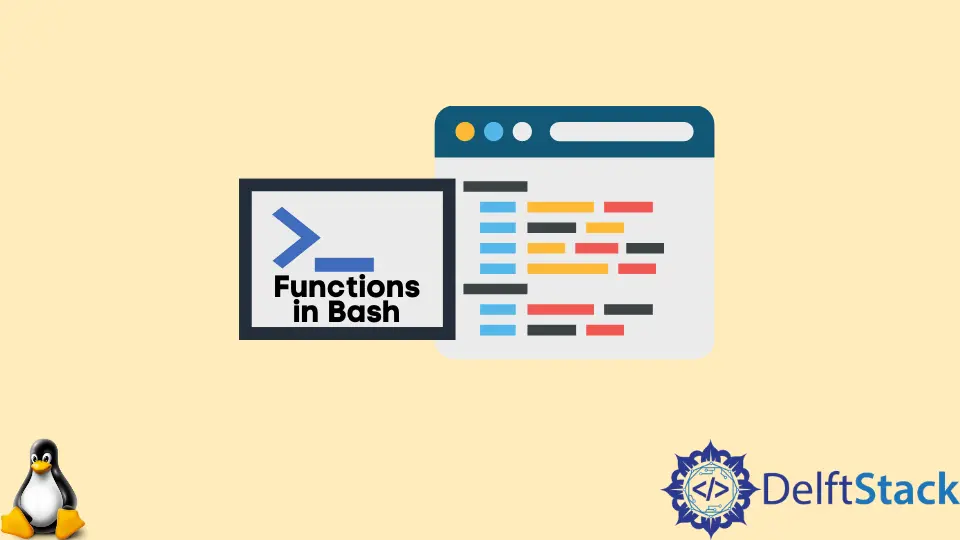
This tutorial explains the basic functions in Bash and their usage in Bash scripts by using functions without the function
keyword and functions with the function
keyword.
Functions in Bash
A function in Bash is a set of commands that achieve a specific task. We can use functions over and over again; this helps to avoid writing the same set of commands repeatedly. Functions also make scripts more readable.
Generally, functions take in data, process it, and return a value. However, in bash scripting, the functions only return the exit status of the last command.
The exit status is any value between 0
and 255
. The exit status, 0
, means the command was executed successfully.
Defining Functions in Bash
In Bash, there are two notations to declare functions. The first notation uses the function name with parentheses, followed by curly braces, as shown below.
name_of_function(){
command1
command2
}
The notation above can also be written in one line.
name_of_function(){command1; command2}
The second notation uses the function
keyword to declare the function, followed by parentheses and curly braces, as shown below.
function name_of_function(){
command1
command2
}
We also declare the function in one line as shown below.
function name_of_function(){command1; command2}
Sample Functions
The script below demonstrates using a function in a bash script. First, we define the function and later execute it by calling it. The below script uses the first syntax of using the function name followed by parentheses and curly brackets.
We define a function named greet
; the curly braces indicate the function’s body. We have two echo
statements inside the function’s body that print text to the standard output.
To call a function in Bash, you type the function’s name. The last line in the script below calls the function greet
.
#!/bin/bash
greet(){
echo "Hello, World!"
echo "Bash is Fun!"
}
greet
The script prints the following output to the standard output.
Hello, World!
Bash is Fun!
The bash script below defines a function using the function
keyword followed by the function name, add
. The add
function adds two numbers, 3
and 7
, using the expr
command and assigns the sum to the var
variable. The echo
command shows the var
variable’s value to the standard output.
The last line with the function’s name is used to call the function for execution.
#!/bin/bash
function add(){
var=`expr 3 + 4`
echo $var
}
add
Variable Scope in Bash Function
In Bash, all variables are global variables by default. We can access global variables anywhere in the Bash script, even inside a function. Variables defined inside a function are also global variables in Bash.
To define a local variable inside a function in Bash, we use the local
keyword.
The script below demonstrates variable scope by defining global variables, x
and y
, and one local variable inside the add
function called sum
.
#!/bin/bash
x=2
y=4
function add(){
local sum
sum=`expr $x + $y`
echo $sum
}
add
From the script above, the variables x
and y
are global variables. We can access these anywhere in the script. The variable sum
is a local variable because the local
keyword has been used to define it.
We can only access a local variable within the function’s body defined. In this case, the local variable sum
can only be used within the body of the add
function. Accessing the local variable, sum
, outside of the add
function body will throw an error.
Return Values in Bash Function
Bash functions do not return values when called like functions in other programming languages. Bash functions only return the exit status of the last command to be executed.
The exit status can be any value between 0
and 255
. The exit status, 0
, means that the command was executed successfully. We can access the exit status by printing the value of $?
.
When the add
function is called, it executes the commands inside its body. The first line assigns the result of the expr
command to the sum
variable.
The second line prints the sum
variable’s value to the standard output using the echo
command. The last line prints the exit status value to the standard output.
The exit status that is printed is that of the command that was executed previously; the exit status code is for the echo
command that displays the value of the sum
variable.
#!/bin/bash
add(){
sum=`expr 2 + 3`
echo "Sum: $sum"
echo "Exit Status: $?"
}
add
Executing the script displays the following output to the standard terminal.
Sum: 5
Exit Status: 0
Passing Arguments to Bash Functions
To pass arguments to a bash function, put the arguments next to the function name separated by a space when calling the function. The $n
notation can access the parameters.
The n
represents the position of the argument next to the function name. In our case, $1
represents the first argument, 1
, and $2
represents the second argument, 2
.
function add(){
sum=`expr $1 + $2`
echo $sum
}
add "1" "2"
The variable $0
is reserved to represent the function name, and the $#
stores the number of positional arguments being passed to the function.
The script above produces the following output to the standard output.
3