How to Convert String to Integer in Bash
- Mathematical Operations on String
-
Use the
expr
Command to Convert String to Integer -
Use the Double Parenthesis
((...))
Construct to Convert String to Integer -
Use the Basic Calculator (
bc
) Command to Convert String to Integer -
Use the
awk
Command to Convert String to Integer -
Use
perl...print
orperl...say
to Convert String to Integer - Use Python to Convert String to Integer
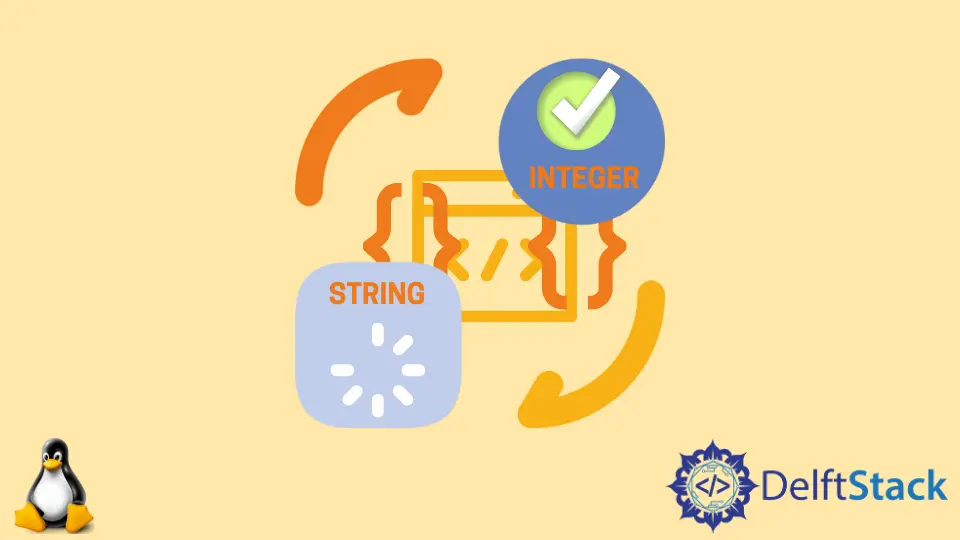
This tutorial will discuss string to integer conversion in Bash script. First, we will discuss issues with the mathematical operations on strings, and then we will see how to convert a string to an integer.
Mathematical Operations on String
Let’s start our discussion with this simple code:
x_value=1000
y_value=20
echo $x_value+$y_value
The output of this code might be unexpected. See Output:
1000+20
The command echo
concatenates the variable x_value
value with a plus sign and the variable y_value
. Probably, you were not interested in getting this output; rather, you were expecting 1020
as output.
After discussing the issue, let’s explore ways to avoid it and get the desired outputs.
Use the expr
Command to Convert String to Integer
This command is used to evaluate expressions and to get the results. For example:
$ expr 12 - 8
The output of this command will be 4
. We can use this command to get our required result:
x_value=1000
y_value=20
expr $x_value + $y_value
The output of this script is 1020
; however, please note that spaces are required between the variable and the operator. Otherwise, this command will also perform concatenation, and the result will be the same.
Note: It is compulsory to add proper spacing among the operands and operators. It makes it easier for
expr
to recognize each token separately.
Use the Double Parenthesis ((...))
Construct to Convert String to Integer
The double parenthesis constructor (( ... ))
permits arithmetic expansion and evaluation; for example, x_value=$(( 6 - 2 ))
would set x_value
to 4
. We can use this construct to get our required result.
Let us look at an example script:
x_value=1000
y_value=20
echo $(( x_value * y_value ))
The output of this script will be 20000
.
Use the Basic Calculator (bc
) Command to Convert String to Integer
In Bash, the basic calculator (bc
) is used to perform fundamental arithmetic calculations directly from the Bash command interface.
For example, we can write:
$ echo "12-4" | bc
The output is 8
. We can use this basic calculator to get our required results.
The code to solve our original problem using bc
looks like this:
x_value=1000
y_value=20
echo $x_value-$y_value | bc
The output is 980
. In this expression, spaces are not required like these were mandatory in the expr
command.
Use the awk
Command to Convert String to Integer
The awk
is a pre-compiled command allowing the user to print formatted expressions containing variables, numeric functions, string functions, and logical operators. You can use this command in a variety of ways.
For example, if you want to print some message with some formatted numeric calculations, you can use the echo
command with the awk
command like:
$ echo salary= 40000 10 | awk '{print $1 $2+$2*$3/100}'
In this command, we want to print salary, where salary includes a 10% bonus. Here, salary=
is our first parameter. We have used it by writing $1
.
Similarly, 40000
is the second parameter used by writing $2
; similarly, $3
is a placeholder for the third parameter (i.e., 10
).
The output of this command is:
salary=44000
We can use the awk
command to solve our original problem as:
x_value=1000
y_value=20
echo $x_value $y_value | awk '{print $1 + $2}'
The output will be 1020
, where x
and y
are two parameters received by the awk
command. The calculations are performed accordingly.
Use perl...print
or perl...say
to Convert String to Integer
We have the print
or say
options in the perl
command. The difference between print
and say
is that the print
will not feed the line, and the next output will come on the same line, whereas the say
command will feed the line.
For example:
x_value = 5
perl -E "print $x_value"
echo "*"
perl -E "say $x_value"
echo "*"
The output will be:
5*5
*
Please note that the second asterisk is printed on the next line because of the line feed by say
instead of print
.
Therefore, we can use the perl
with print
or say
to get our required Output:
x_value=1000
y_value=20
perl -E "print $x_value+$y_value"
The output will be 1020
.
Use Python to Convert String to Integer
Python has a print
statement that can evaluate and print an expression.
For example:
python -c "print 5 * 4"
The output will be 20
. We can use this to get our required results.
The code is shown below:
x_value=1000
y_value=20
python -c "print $x_value * $y_value"
The output is 20000
.
We have a variety of ways to convert a string into an integer variable to perform mathematical operations. Here, we have presented six different ways, and you can use anyone as per your choice.