How to Remove First Character From String in Bash
-
Use the
sed
Command to Remove the First Character From the String in Bash -
Use the
cut
Command to Remove the First Character From the String in Bash -
Use the
substring
Parameter to Remove the First Character From the String in Bash
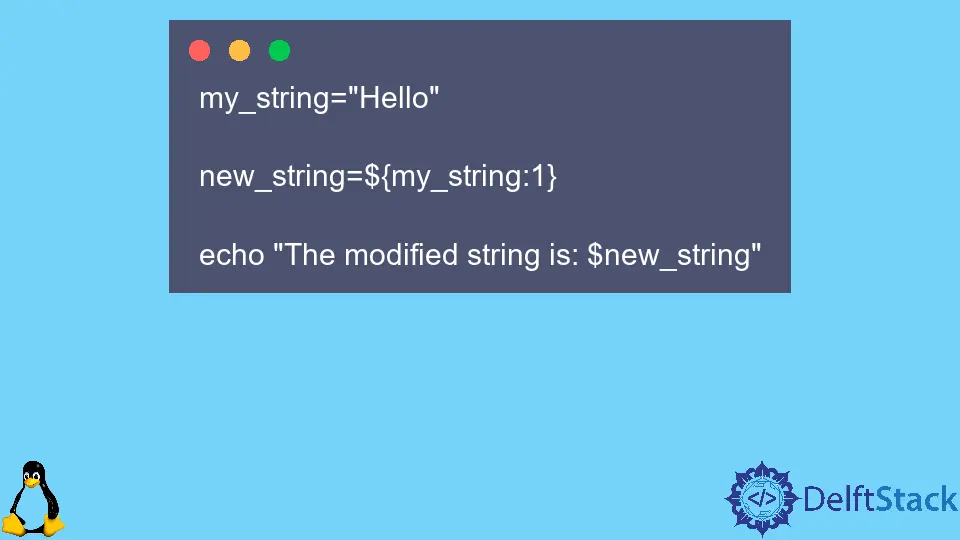
Removing the first character of a string in Bash can be tricky, as there is no built-in function that can do this directly. However, there are several ways to achieve this, such as using the sed
command, the cut
command, or the substring
parameter expansion.
This post will review how to use each approach to remove the initial character from a string in Bash.
Use the sed
Command to Remove the First Character From the String in Bash
In Bash, you can delete the initial character of a string using the sed
command, and we can use the s
(substitute) command with the ^
(start of line) regular expression. The s
command enables us to look for a pattern in a string and swap out that string for another string, while the ^
regular expression matches the start of the line.
For example, let’s say we have a string called my_string
that contains the word Welcome
, and we want to remove the first character (the letter W
). We can use the sed
command to achieve this:
my_string="Welcome"
new_string=$(echo "$my_string" | sed 's/^.//')
echo "The modified string is: $new_string"
Output:
The modified string is: elcome
In the code above, we use the sed
command to search for the pattern ^.
in the my_string
variable and replace it with an empty string. The ^
character matches the start of the line, while the .
character matches any single character.
The sed
command will remove the string’s first character, the letter W
.
The sed
command output is then stored in the new_string
variable, and we use the echo
command to print the modified string. The output of the code should be The modified string is: elcome
.
Use the cut
Command to Remove the First Character From the String in Bash
Another Bash method for removing the first character from a string is to use the cut
command, which allows us to extract a specified number of characters from a string. The cut
command takes a string as input and outputs only the specified characters.
To remove the first character of a string using the cut
command, we can use the -c
option to specify the character positions to be extracted. For example, let’s say we have the same my_string
variable containing the word “Hello”, and we want to remove the first character (the letter “H”). We can use the cut
command to achieve this:
my_string="Hello"
new_string=$(echo "$my_string" | cut -c 2-)
echo "The modified string is: $new_string"
Output:
The modified string is: ello
In the code above, we use the cut
command with the -c
option to extract the characters from the second position (2
) to the end of the string (-
). This means that the cut
command will remove the string’s first character, which is the letter “H” in this case.
The output of the cut
command is then stored in the new_string
variable, and we use the echo
command to print the modified string. The output of the code should be “The modified string is: ello”.
Use the substring
Parameter to Remove the First Character From the String in Bash
Finally, we can remove the first character of a string in Bash using the substring
parameter expansion. This special syntax allows us to perform string manipulation operations on variables directly in the Bash script without using external commands like sed
or cut
.
To remove the first character of a string using the substring
parameter expansion, we can use the ${string:position}
syntax, where string
is the variable containing the string, and position
is the starting position of the substring to be extracted. For example, let’s say we have the same my_string
variable containing the word Hello
, and we want to remove the first character (the letter H
). We can use the substring
parameter expansion to achieve this:
my_string="Hello"
new_string=${my_string:1}
echo "The modified string is: $new_string"
Output:
The modified string is: ello
In the code above, we use the ${my_string:1}
syntax to extract the substring starting from the second position (1
) of the my_string
variable. The first character (the letter H
) will be removed from the string.
The substring
parameter expansion output is then stored in the new_string
variable, and we use the echo
command to print the modified string. The output of the code should be The modified string is: ello
.
In conclusion, there are several ways to remove the first character of a string in Bash, such as using the sed
command, the cut
command, or the substring
parameter expansion. Each of these methods has its advantages and disadvantages, and the best choice will
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn