How to Remove Newline From String in Bash
- Create a String With Newline Characters in Bash
- Remove Newline Characters From a String Using Bashims in Bash
-
Remove Newline From a String Using the
tr
Command in Bash -
Remove Newline From a String Using the
extglob
Option in Bash -
Remove Newline From a String Using the
sed
Command in Bash -
Remove Newline From a String Using the
awk
Command in Bash - Conclusion
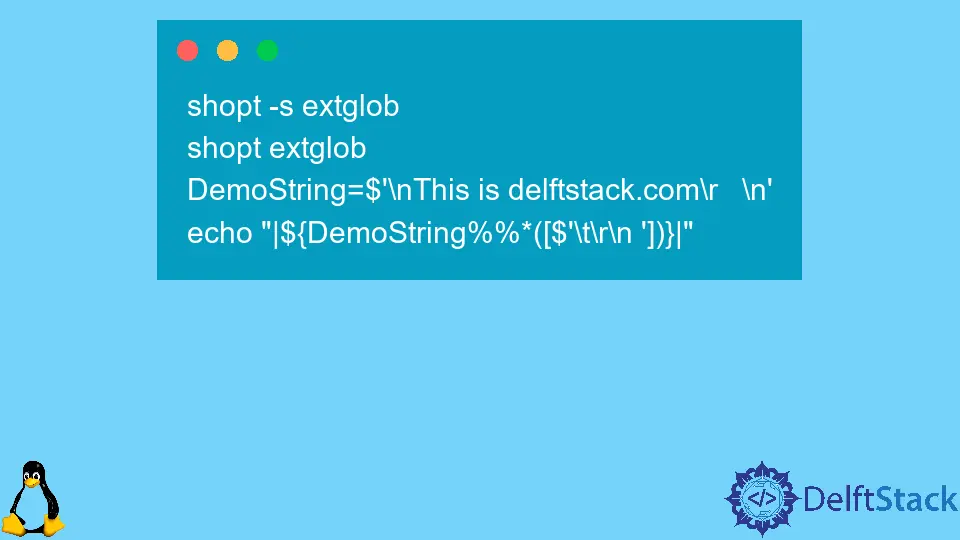
This tutorial demonstrates how to remove a newline from a string in Bash.
Create a String With Newline Characters in Bash
In certain situations, it’s necessary to eliminate newline characters from a string. Thankfully, Bash offers various techniques to achieve this.
To illustrate, let’s begin by generating a string that contains newline characters. We can create a new string using the following command:
DemoString=$'\nThis is delftstack.com\r \n'
echo "|${DemoString}|"
The command above initializes a string, incorporating newline characters represented by \n
. Here’s the resulting output:
|
This is delftstack.com
|
Remove Newline Characters From a String Using Bashims in Bash
Bash provides a set of built-in capabilities that enable us to efficiently manipulate strings and deal with newline characters. One of the useful features it offers is the //
operator, which allows us to remove newline characters from a string.
Let’s dive into the specifics of using //
to eliminate newline characters from a string. Below are two examples illustrating its usage:
echo "|${DemoString//[$'\t\n\r']}|"
echo "|${DemoString//[$'\t\n\r' ]}|"
In the above commands, we have two variations. The first one uses the parameters \t\n\r
, while the second includes these parameters along with a space character.
Both of these commands leverage bashims to effectively remove newline characters as well as space characters from the given string.
Now, let’s take a look at the output:
|This is delftstack.com |
|Thisisdelftstack.com|
The output demonstrates the successful removal of newline characters and space characters from the original string.
This approach leverages parameter expansion to achieve the desired outcome. In the line echo "|${DemoString//[$'\t\n\r']}|"
, parameter expansion is employed to replace all occurrences of the specified characters, namely tab (\t
), newline (\n
), and carriage return (\r
), with an empty string, effectively erasing them from the DemoString
.
Additionally, the line echo "|${DemoString//[$'\t\n\r' ]}|"
provides an example of how to remove not only tabs, newlines, and carriage returns but also spaces from the original string. This flexibility in string manipulation is a valuable feature offered by bash, making it a powerful tool for various text-processing tasks.
Remove Newline From a String Using the tr
Command in Bash
The tr
command, similar to bashims, serves the purpose of both deleting and substituting characters within a given string. However, we will primarily focus on its deletion functionality, achieved by using the -d
option in the command.
Let’s illustrate this with an example where we want to remove newline characters from a given string using the tr
command:
DemoString=$'\nThis is delftstack.com\r \n'
echo "|$DemoString|" | tr -d '\n\r'
In the above command, our objective is to eliminate newline characters from the provided string. Here is the output:
|This is delftstack.com |
Furthermore, the tr
command can also be used to remove additional spaces from the string. Take a look at this example:
DemoString=$'\nThis is delftstack.com\r \n'
echo "|$DemoString|" | tr -d '\n\r '
In this case, the command not only removes newline and carriage return characters but also any extra spaces within the string. The output is as follows:
|Thisisdelftstack.com|
Remove Newline From a String Using the extglob
Option in Bash
Moreover, the extglob
option in Bash provides a versatile set of functionalities, including the ability to remove white spaces and newline characters. Before utilizing this option, it’s essential to ensure that it’s enabled in your Bash environment.
This feature offers various options tailored for different operations. Let’s take a look at the available options in the table below:
Option | Description |
---|---|
? |
Matches zero or one occurrence of the specified patterns. |
* |
Matches zero or more occurrences of the specified patterns. |
+ |
Matches one or more occurrences of the specified patterns. |
@ |
Matches one of the provided patterns. |
! (pattern-list) |
Matches anything except the specified patterns. |
To demonstrate how the extglob
option can remove newline characters from a given string, let’s walk through an example:
shopt -s extglob
shopt extglob
DemoString=$'\nThis is delftstack.com\r \n'
echo "|${DemoString%%*([$'\t\r\n '])}|"
In this example, we first enable the extglob
option with shopt -s extglob
. The subsequent command, shopt extglob
, displays the current status of the extglob
option.
Finally, the last command effectively removes the newline character using the extglob
.
Here’s the resulting output:
extglob on
|
This is delftstack.com|
Remove Newline From a String Using the sed
Command in Bash
The sed
command, short for stream editor, is a powerful tool in the UNIX and Linux environments used for parsing and transforming text. One common operation is removing newline characters from a string or a file.
Here’s how to accomplish this task using sed
:
DemoString=$'\nThis is delftstack.com\r \n'
cleaned_string=$(echo "$DemoString" | sed ':a;N;$!ba;s/\n//g')
echo "$cleaned_string"
See the output:
extglob on
|
This is delftstack.com|
Let’s break down the code to understand how it works.
As you can see, we start by defining a variable string_with_newlines
that holds the input string containing newline characters.
Then, the sed
command is used to perform text transformations. In this case, we are using the following expression to remove newline characters:
:a
: This label defines a point in thesed
script to which we can branch.N
: This command appends the next line of input into the pattern space.$!ba
: This constructs a loop that branches back to labela
unless it’s the last line.s/\n//g
: This is the substitution command (s
), which replaces newline characters (\n
) with nothing (an empty string). Theg
flag is used to replace all occurrences on each line.
Finally, we echo
the cleaned string to the standard output, showing the result of removing newline characters.
Remove Newline From a String Using the awk
Command in Bash
Removing newline characters from a string in Bash can also be efficiently achieved using awk
. This command operates on a line-by-line basis, processing each line of input data according to a set of rules.
These rules, also known as patterns and actions, determine what awk
does with each line of data.
Here’s the basic syntax of an awk
command:
awk 'pattern { action }' input-file
Parameters:
pattern
: A condition or expression that defines when the specified action should be performed. If omitted, the action is applied to every line.{ action }
: The action or series of commands to execute on lines that match the pattern.input-file
: The file or data source to process. If omitted,awk
reads from standard input (usually provided via a pipeline or redirection).
Here’s how we can use the awk
command to remove newline characters in the Bash shell.
DemoString=$'\nThis is delftstack.com\r \n'
cleaned_string=$(echo "$DemoString" | awk '{ printf "%s", $0 }')
echo "$cleaned_string"
Again, we start by defining a variable DemoString
that holds the input string containing newline characters and other special characters like carriage return and extra spaces.
Then, the awk
command is used to process text based on pattern-action rules. In this case, we’re using a simple rule to remove newline characters.
{ printf "%s", $0 }
: This awk
rule prints the entire input line without the newline character. $0
represents the entire input line.
Finally, we echo the cleaned string to the standard output, showing the result of removing newline characters from the original string.
Conclusion
This tutorial has demonstrated various methods to remove newline characters from a string in Bash. Whether through the //
operator, the tr
command, the extglob
option, the sed
command, or the line-by-line processing of awk
, Bash provides a range of tools for string manipulation.
These techniques enable users to effectively handle newline characters and other special characters within strings, making Bash a valuable resource for text-processing tasks. Depending on your specific requirements, you can choose the method that best suits your needs to clean and manipulate strings in Bash.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook