String Comparison Operator in Bash
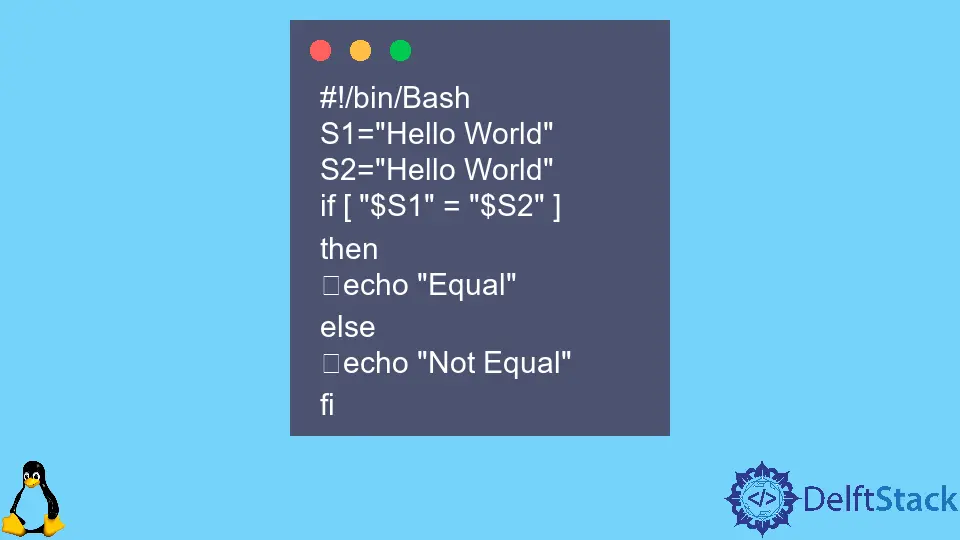
In this article, we will explain the string comparison in Bash using the if
statement.
A shell program running in Linux that provides the command line interface for users to execute different commands is called Bash shell. It is also used as a default shell in many distributions of Linux, known as GNU Bourne-Again Shell (Bash).
Bash Script
A series of Bash commands written in a file is called Bash script. The Bash shell executes these commands after reading from the file.
The file extension of a Bash script is .sh
. The following contents of a file named First.sh
are shown below.
#!/bin/Bash
echo "Hello World"
The above file First.sh
is a Bash script containing only one echo
command, which displays Hello world
on the terminal.
The first line of the file #!/bin/Bash
informs about the Bash program in the system, which works as an interpreter to run the command written in the script file.
The Bash shell provides different methods to execute the script on the terminal. Some methods are discussed below.
-
A
bash
command followed by the Bash script file is used to execute the script. The following command runs theFirst.sh
script.bash First.sh
The output of this command is:
Hello World
-
Run Bash script by specifying the path. After assigning the executable right to the script file using
chmod +x First.sh
, we can run the script file by specifying the path of the script.<path to the script file>/First.sh
We can use the absolute path using the above method or the relative path to the script using
./First.sh
to run the script.
String Variable in Bash
We can declare and initialize any string in a variable using the assignment operator (=
) in the Bash script.
For example:
#!/bin/Bash
S="Hello World"
echo $S
In the above example, we declare a string variable S
and initialize it with Hello World
as a value. The echo
command displays the value of the string variable on the terminal using the echo
command and $
operator.
String Comparison Operator in Bash Script
We can compare two strings using the =
(is equal to) operator in the Bash script. We also use the ==
operator to compare the string.
The ==
is a synonym of the =
operator for string comparison.
For example, consider a Bash script First.sh
containing the following contents.
#!/bin/Bash
S1="Hello World"
S2="Hello World"
if [ "$S1" = "$S2" ]
then
echo "Equal"
else
echo "Not Equal"
fi
The following script contains two strings, S1
and S2
have the same value. The if
condition compare the string using =
operator; however, we can also use if [ "$S1" == "$S2" ]
statement to compare these strings.
The following is the First.sh
script output.
Equal
Note: If we remove whitespace before and after the
=
operator in theif
statement, for example,if [ "$S1"="$S2" ]
, it will not compare the strings accurately.
The !=
(is not equal) operator is used for the inequality matching of two strings. We can use this operator in if
statement using if [ "$S1" != "$S2" ]
.
This statement returns true
if the strings S1
and S2
are not equal.