How to Generate Random Number in a Specified Range in JavaScript
- Understanding Math.random()
- Generating a Random Integer in a Specified Range
- Generating a Random Floating-Point Number in a Specified Range
- Conclusion
- FAQ
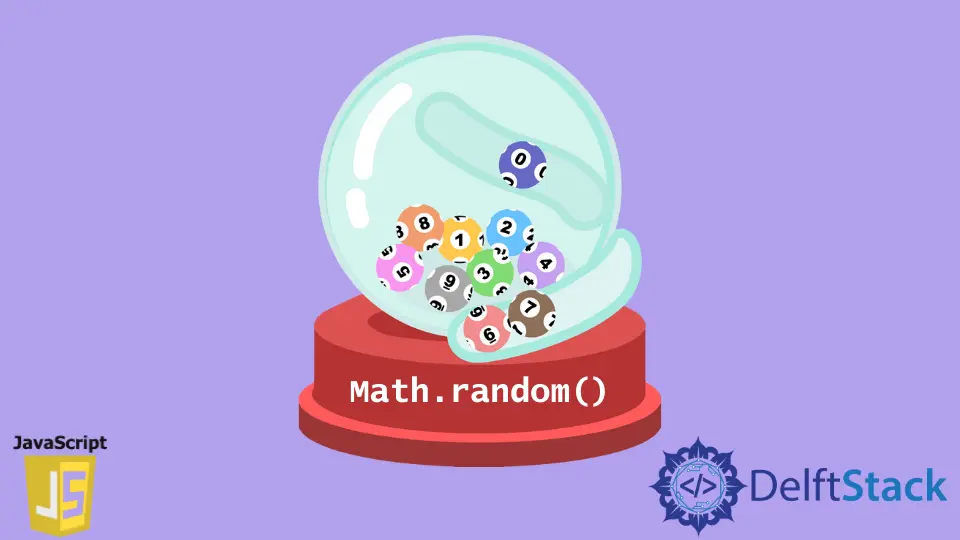
Generating random numbers is a common requirement in programming, especially when it comes to creating games, simulations, or any application that requires randomness. In JavaScript, the built-in Math.random()
function is a powerful tool for generating random numbers. However, it generates numbers between 0 (inclusive) and 1 (exclusive). To create a random number within a specified range, you’ll need to do a bit of math.
This tutorial will guide you through the process of generating random numbers in a defined range using JavaScript, ensuring you have all the tools you need to implement this functionality effectively.
Understanding Math.random()
Before diving into generating random numbers in a specified range, let’s take a moment to understand how the Math.random()
function works. This function returns a floating-point, pseudo-random number between 0 and 1. This means you can generate a number like 0.123456 or 0.987654, but you can’t get a number like 1 or -0.5.
To generate a number in a specific range, you’ll need to scale and shift the output of Math.random()
. For example, if you want a random number between min
and max
, you can use the following formula:
randomNumber = Math.random() * (max - min) + min;
This formula scales the random number to the desired range and shifts it to start from min
. Let’s explore how to implement this in practice.
Generating a Random Integer in a Specified Range
If you’re looking to generate a random integer within a specific range, the approach is slightly different. You can use the following code snippet:
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(getRandomInt(1, 10));
Output:
7
In this example, the getRandomInt
function takes two parameters: min
and max
. The Math.ceil(min)
ensures that the minimum value is rounded up to the nearest integer, while Math.floor(max)
rounds down the maximum value. The expression Math.random() * (max - min + 1)
generates a random number in the range of 0
to max - min + 1
, and adding min
shifts it to the desired range. Finally, Math.floor()
converts the floating-point number to an integer.
This method is particularly useful for scenarios like generating random dice rolls or selecting random items from a list, where you need whole numbers rather than decimals.
Generating a Random Floating-Point Number in a Specified Range
If you need a random floating-point number within a specified range, the code is even simpler. Here’s how you can do it:
function getRandomFloat(min, max) {
return Math.random() * (max - min) + min;
}
console.log(getRandomFloat(1.5, 5.5));
Output:
3.276
In this case, the getRandomFloat
function works similarly to the integer version but does not require rounding. The formula Math.random() * (max - min) + min
produces a floating-point number between min
and max
. This is ideal for scenarios where precision is key, such as in simulations or scientific computations.
The beauty of using Math.random()
is its simplicity and efficiency, making it a go-to solution for generating random numbers in JavaScript.
Conclusion
Generating random numbers in JavaScript is a straightforward task when you understand how to manipulate the Math.random()
function. Whether you need integers or floating-point numbers, the methods outlined in this tutorial give you the flexibility to produce random values within any specified range. With just a few lines of code, you can enhance your applications, making them more dynamic and engaging. Embrace the power of randomness in your JavaScript projects, and watch your creativity flourish.
FAQ
-
How does Math.random() work in JavaScript?
Math.random() generates a pseudo-random floating-point number between 0 (inclusive) and 1 (exclusive). -
Can I generate random numbers in a negative range?
Yes, you can generate random numbers in a negative range by adjusting the min and max values accordingly. -
Is Math.random() truly random?
Math.random() is pseudo-random, meaning it uses algorithms to produce numbers that appear random but are not truly random. -
Can I use Math.random() for cryptographic purposes?
No, Math.random() is not suitable for cryptographic purposes. For secure random numbers, consider using the Web Crypto API. -
How can I generate a random array of numbers?
You can use a loop to call the random number generation function multiple times and store the results in an array.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Math
- How to Convert Radians to Degrees in JavaScript
- BigDecimal in JavaScript
- How to Add Two Numbers in JavaScript
- How to Use Scientific Notation in JavaScript
- How to Calculate the Percentage in JavaScript
- How to Round Number to the Nearest 10 in JavaScript