How to Add Two Numbers in JavaScript
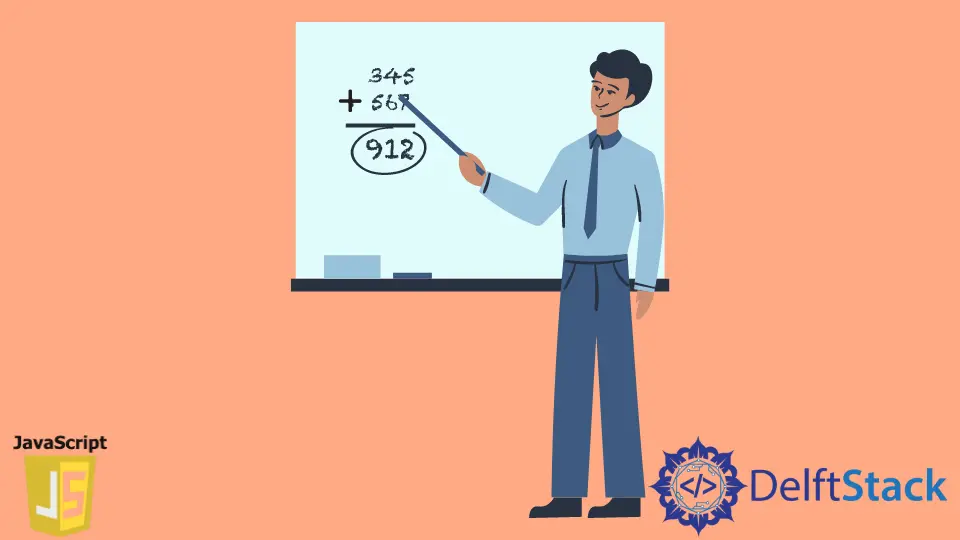
In this article, we will learn and use arithmetic operations in JavaScript source code and how to get the sum of multiple numeric values and use the default alert box and log box to display the result to the user.
Add Numbers in JavaScript
To find out arithmetic operations results like (addition, subtraction, multiplication, and division) we use operators in JavaScript code statements. Developers mostly use these operators to perform calculations and further mathematical problems in their programs.
To find the addition, programmers use the +
operator with numeric values.
Addition (+
) Operator in JavaScript
In JavaScript, the +
operator gets the sum result of numeric values. If we use the +
operator in string values, it will perform concatenation between strings.
Syntax:
let value1 = 10
let value2 = 5
let sum = value1 + value2 // addition of values
console.log(sum);
We need multiple number values assigned or values provided by the user to perform the addition operation. In the below example, we’ll get two values from user input and perform an addition operation, then display the result to the user.
Example Code:
<html>
<head>
<title> display JavaScript alert </title>
</head>
<script>
function createSum()
{
let data1 = document.getElementById("value1").value;
data1 = parseInt(data1)
let data2 = document.getElementById("value2").value;
data2 = parseInt(data2)
if(isNaN(data1) || isNaN(data2)) //check empty data field
{
alert("Please enter both values");
}else{
let sum = data1+data2;
alert("Your'e sum value is : "+sum);
}
}
</script>
<body>
<h1 style="color:blueviolet">DelftStack Learning</h1>
<h3>JavaScript Add two values</h3>
<form onsubmit ="return createSum()">
<!-- data input -->
<td> Enter 1st Value: </td>
<input id = "value1" type="number">
<td> Enter 2nd Value: </td>
<input id = "value2" type="number">
<br><br>
<input type = "submit" value = "Sum values">
</form>
</body>
</html>
Output:
We used the form element in the above HTML source code to create user input fields and submit button. The user will insert both values, and when the user clicks submit button, the function createSum()
will trigger.
In script tags, we have declared the createSum()
function, and inside that function, we are getting user input values in the data1
and data2
variables and used the conditional statement if
to check the input values.
If values are empty or do not contain a number, it will display an error message alert()
. If the user provides both numeric values, then, in the else
condition, we performed addition using the +
operator, stored the result in the sum
variable, and displayed it in the alert()
popup.