BigDecimal in JavaScript
- Using BigDecimal with Libraries
- Step 1: Install bigdecimal.js
- Step 2: Importing the Library
- Step 3: Creating BigDecimal Instances
- Performing Arithmetic Operations
- Subtraction Example
- Multiplication Example
- Division Example
- Conclusion
- FAQ
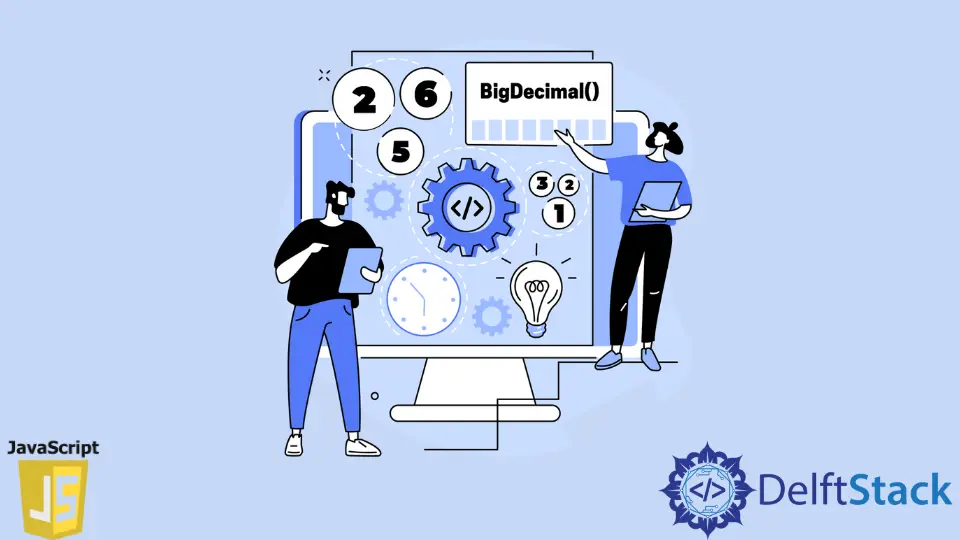
BigDecimal is a data type that provides a way to represent and manipulate decimal numbers with a high degree of accuracy. Unlike the standard Number type in JavaScript, which can introduce rounding errors in calculations, BigDecimal ensures that your decimal arithmetic is precise. This is particularly important in fields like finance, where even the smallest discrepancy can have significant repercussions.
BigDecimal is not natively available in JavaScript, but libraries such as bigdecimal.js provide this functionality. These libraries allow developers to create BigDecimal instances and perform operations such as addition, subtraction, multiplication, and division without losing precision.
Using BigDecimal with Libraries
To use BigDecimal in JavaScript, you’ll typically rely on third-party libraries. Below, we will explore how to implement BigDecimal using the bigdecimal.js library, which is one of the popular options available.
Step 1: Install bigdecimal.js
Before you can start using BigDecimal, you need to install the bigdecimal.js library. You can do this using npm, a package manager for JavaScript.
npm install bigdecimal.js
This command will download and install the bigdecimal.js library into your project, making it available for use.
Step 2: Importing the Library
Once bigdecimal.js is installed, you can import it into your JavaScript file. Here’s how to do that:
const { Big } = require('bigdecimal.js');
By importing the library, you can now create BigDecimal instances and perform calculations2.
Step 3: Creating BigDecimal Instances
Now that you have imported bigdecimal.js, you can create instances of BigDecimal. Here’s a simple example:
const num1 = Big('0.1');
const num2 = Big('0.2');
const sum = num1.add(num2);
console.log(sum.toString());
In this example, we create two BigDecimal instances, num1
and num2
, representing 0.1 and 0.2, respectively. We then add them together using the add
method and print the result2.
Output:
0.3
This output demonstrates that the addition of 0.1
and 0.2
using BigDecimal yields the expected result without any rounding errors.
Performing Arithmetic Operations
BigDecimal not only allows for precise addition but also supports various arithmetic operations. Let’s explore how to perform subtraction, multiplication, and division with BigDecimal.
Subtraction Example
To subtract two BigDecimal numbers, you can use the subtract
method. Here’s an example:
const num3 = Big('0.3');
const difference = num3.subtract(num1);
console.log(difference.toString());
In this code, we subtract num1
from num3
and log the result.
Output:
0.2
This example shows that the subtraction operation maintains precision, providing accurate results.
Multiplication Example
Multiplying two BigDecimal numbers is equally straightforward. You can use the multiply
method for this operation:
const num4 = Big('0.1');
const product = num4.multiply(num2);
console.log(product.toString());
Here, we multiply num4
by num2
and print the result.
Output:
0.02
The multiplication operation also preserves accuracy, demonstrating the strength of using BigDecimal for decimal arithmetic.
Division Example
Division can also be performed without losing precision. Here’s how:
const num5 = Big('1');
const quotient = num5.divide(num4);
console.log(quotient.toString());
In this example, we divide 1
by num4
and log the result.
Output:
10
This example illustrates that division with BigDecimal yields precise results, making it ideal for financial calculations.
Conclusion
BigDecimal in JavaScript is an invaluable tool for developers who need to perform precise decimal arithmetic. By utilizing libraries like bigdecimal.js, you can avoid the pitfalls of floating-point arithmetic and ensure that your calculations are accurate. Whether you’re working on financial applications, scientific computations, or any task that requires precision, BigDecimal can help you achieve your goals. Embracing this approach will not only enhance the reliability of your code but also improve user trust in your applications
FAQ
- Q: What is BigDecimal in JavaScript?
A: BigDecimal is a data type that allows for arbitrary-precision decimal arithmetic in JavaScript, ensuring accurate calculations without rounding errors typically associated with floating-point numbers. - Q: Why should I use BigDecimal instead of regular numbers in JavaScript?
A: Regular numbers in JavaScript can introduce rounding errors, especially with decimal arithmetic. BigDecimal provides a solution by maintaining precision, which is crucial for financial calculations, scientific computations, or any application requiring exact decimal representation. - Q: Which library should I use for BigDecimal functionality in JavaScript?
A: While there are several options, bigdecimal.js is a popular and reliable library for implementing BigDecimal functionality in JavaScript. Other alternatives include decimal.js and big.js. - Q: Can I perform all standard arithmetic operations with BigDecimal?
A: Yes, you can perform addition, subtraction, multiplication, and division with BigDecimal while maintaining precision. Most BigDecimal libraries also support additional operations like rounding, comparison, and exponentiation. - Q: Is BigDecimal suitable for performance-critical applications?
A: While BigDecimal provides high precision, it can be slower than native JavaScript number operations. For performance-critical applications, you should balance the need for precision with performance requirements. In many cases, the accuracy benefits outweigh the slight performance cost, especially in financial and scientific applications.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn