How to Round Number to the Nearest 10 in JavaScript
-
Use
Math.ceil()
to Round a Number Up to the Nearest 10 in JavaScript -
Use
Math.round
to Round a Number to the Nearest 10 in JavaScript -
Use the Modulo Operator (
%
) to Round a Number to the Nearest 10 in JavaScript - Conclusion
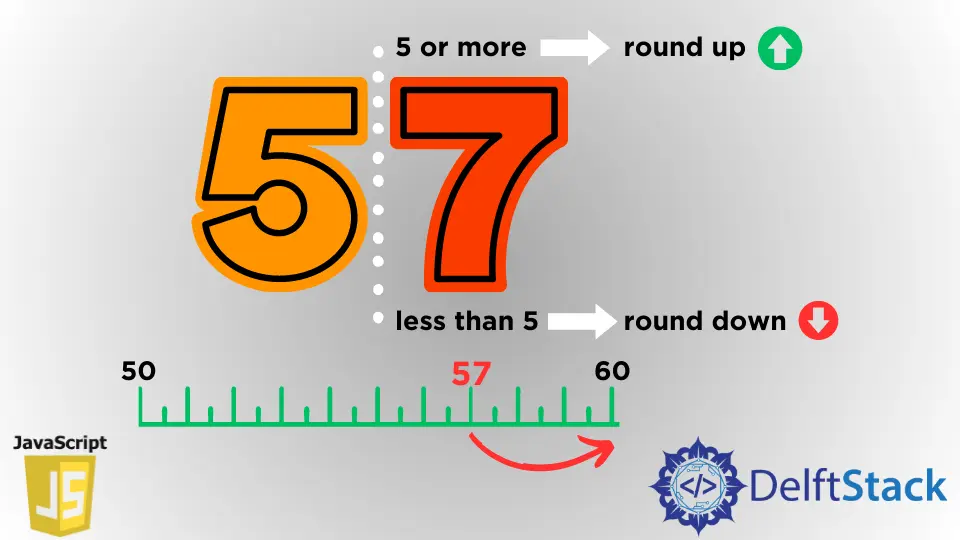
Rounding numbers is a common operation in JavaScript, and rounding to the nearest 10 is a specific case that often arises in practical programming scenarios.
In this article, we will learn different methods for achieving this task in JavaScript. Each method will be accompanied by example codes and detailed explanations of how it works.
Use Math.ceil()
to Round a Number Up to the Nearest 10 in JavaScript
The Math.ceil()
function in JavaScript returns the smallest integer greater than or equal to a given number. Essentially, it rounds a number up to the next whole number.
Syntax:
Math.ceil(x)
Here, x
is the number that you want to round up to the nearest integer. The Math.ceil()
function takes one argument, which is the number you want to round up.
For rounding a number up to the nearest 10, you can call the Math.ceil()
function, passing the number divided by 10
as a parameter and multiplying the result by 10
, e.g., Math.ceil(num / 10) * 10
.
The Math.ceil
function will round a number to the next largest integer and return the result. To round a number up to the nearest 10 using Math.ceil()
, we’ll apply a simple mathematical approach.
function roundUpNearest10(num) {
return Math.ceil(num / 10) * 10;
}
console.log(roundUpNearest10(101)); // 110
console.log(roundUpNearest10(89.9)); // 90
console.log(roundUpNearest10(80.01)); // 90
console.log(roundUpNearest10(-59)); // -50
console.log(roundUpNearest10(-60)); // -60
This code is an implementation of the roundUpNearest10
function in JavaScript. This function uses Math.ceil()
to round a number up to the nearest multiple of 10.
In the first example, roundUpNearest10(101)
, 101 / 10
equals 10.1
. Math.ceil(10.1)
returns 11
. Multiplying by 10
results in 110
. This is the same explanation for all the examples in that code.
Output:
Another example can be found below.
The Math.ceil
function rounds the number up if the passed-in number has a fractional part. On the other hand, if an integer is passed, the function returns the same number.
When the Math.ceil
function is invoked with a null
value, it will return 0
.
console.log(Math.ceil(6.01)); // 7
console.log(Math.ceil(61.00001)); // 62
console.log(Math.ceil(60)); // 60
console.log(Math.ceil(-33.99)); // -33
console.log(Math.ceil(null)); // 0
Output:
A step-by-step process is given in this code example below.
console.log(31 / 10); // 3.1
console.log(50 / 10); // 5
console.log(Math.ceil(31 / 10)); // 4
console.log(Math.ceil(50 / 10)); // 5
console.log(Math.ceil(41 / 10) * 10); // 50
console.log(Math.ceil(60 / 10) * 10); // 60
First, we divide the number by 10
and round the result to the next largest integer. Then, we multiply the result by 10
to get the number rounded up to the nearest 10.
Output:
Using Math.floor()
and Math.ceil()
The combination of Math.floor()
and Math.ceil()
allows us to round a number to the nearest 10 by first flooring it down and then ceiling it up.
Syntax:
Math.floor(x)
Here, x
is the number you want to round down to the nearest integer. The Math.floor()
function takes one argument, which is the number you want to round down.
Example Code:
function roundToNearest10(num) {
let floorNum = Math.floor(num / 10) * 10;
let ceilNum = Math.ceil(num / 10) * 10;
return (ceilNum - num) < (num - floorNum) ? ceilNum : floorNum;
}
let roundedNumber = roundToNearest10(27);
console.log(roundedNumber); // Output: 30
In this method, we first use Math.floor()
to round down the number to the nearest multiple of 10. Next, we use Math.ceil()
to round up to the nearest multiple of 10.
Finally, we compare the differences between the original number and the floor and ceiling values. The smaller difference determines the nearest 10.
Use Math.round
to Round a Number to the Nearest 10 in JavaScript
The Math.round()
function in JavaScript returns the nearest integer to a given number. It applies the standard rounding rules, rounding to the nearest integer. If the fractional part is exactly 0.5, it rounds towards the nearest even number.
Syntax:
Math.round(x)
Here, x
is the number that you want to round to the nearest integer. The Math.round()
function takes a single argument, which is the number you want to round.
To round a number to the nearest 10 using Math.round()
, we can leverage a simple mathematical approach by passing the number divided by 10
and multiplying the result by 10
, e.g., Math.round(num / 10) * 10
.
The Math.round
function will take a number, round it to the nearest integer, and return the result.
function roundToNearest10(num) {
return Math.round(num / 10) * 10;
}
console.log(roundToNearest10(34)); // 30
console.log(roundToNearest10(35)); // 40
console.log(roundToNearest10(64.9)); // 60
console.log(roundToNearest10(-36)); // -40
console.log(roundToNearest10(-35)); // -30
In the first example, roundToNearest10(34)
, 34 / 10
is 3.4
, Math.round(3.4)
is 3
, multiply it by 10
gives 30
. So, roundToNearest10(34)
returns 30
.
This is the same explanation for all the examples in the code.
Output:
If the number has a fractional part of exactly 0.5
, the number will get rounded off to the next integer in the direction of positive infinity.
Below are more examples of using the Math.round
function. The Math.round
function returns 0
when called with a null
value.
console.log(Math.round(8.49)); // 8
console.log(Math.round(8.5)); // 9
console.log(Math.round(80)); // 80
console.log(Math.round(-84.5)); // -84
console.log(Math.round(-84.51)); // -85
console.log(Math.round(null)); // 0
Output:
A step-by-step process is given in this code example below.
console.log(65 / 10); // 6.5
console.log(44 / 10); // 4.4
console.log(Math.round(65 / 10)); // 7
console.log(Math.round(44 / 10)); // 4
console.log(Math.round(65 / 10) * 10); // 70
console.log(Math.round(44 / 10) * 10); // 40
First, we divide the number by 10
and round the result to the nearest integer. Multiply the result by 10
to get the number rounded to the nearest 10.
Output:
Use the Modulo Operator (%
) to Round a Number to the Nearest 10 in JavaScript
The modulo operator (%
) returns the remainder of a division operation. For example, a % b
gives the remainder when a
is divided by b
.
This operator is particularly useful when you want to perform operations related to cycles, repetitions, or remainders.
To round a number to the nearest multiple of 10 using the modulo operator, we’ll follow these steps:
-
Divide by 10
Divide the original number by
10
. This gives us the quotient, which represents how many times 10 fits into the original number. -
Get the Remainder
Use the modulo operator (
%
) to find the remainder when the original number is divided by10
. This is the key step in rounding to the nearest 10. -
Conditional Rounding
Depending on the remainder, we’ll decide whether to round up or down.
- If the remainder is less than 5, we’ll round down.
- If the remainder is 5 or greater, we’ll round up.
-
Adjustment and Multiplication
Add the remainder to or subtract it from the original number to bring it to the nearest 10. Then, multiply by
10
to get the final rounded value.
Let’s put these steps into JavaScript code. We’ll create a function called roundToNearest10
:
function roundToNearest10(num) {
// Step 1: Divide by 10
let quotient = Math.floor(num / 10);
// Step 2: Get the Remainder
let remainder = num % 10;
// Step 3: Conditional Rounding
if (remainder < 5) {
remainder = 0; // Round down
} else {
remainder = 10; // Round up
}
// Step 4: Adjustment and Multiplication
return (quotient * 10) + remainder;
}
Let’s apply our function to some test cases:
console.log(roundToNearest10(34)); // Output: 30
console.log(roundToNearest10(35)); // Output: 40
console.log(roundToNearest10(64.9)); // Output: 60
console.log(roundToNearest10(-36)); // Output: -40
Conclusion
In JavaScript, rounding numbers to the nearest 10 can be achieved through various methods:
Math.ceil()
rounds up a number to the next multiple of 10.- Combining
Math.floor()
andMath.ceil()
allows for a dual approach, rounding down and then up, to determine the nearest 10. Math.round()
provides standard rounding rules, rounding to the nearest integer, and can be used for rounding to the nearest 10.- The modulo operator (
%
) offers an efficient way to round to the nearest 10 by leveraging remainders.
By mastering these techniques, you’ll have versatile tools at your disposal for precise numerical operations in JavaScript.