How to Calculate the Percentage in JavaScript
- Method 1: Basic Percentage Calculation
- Method 2: Using a Percentage Object
- Method 3: Percentage Change Calculation
- Conclusion
- FAQ
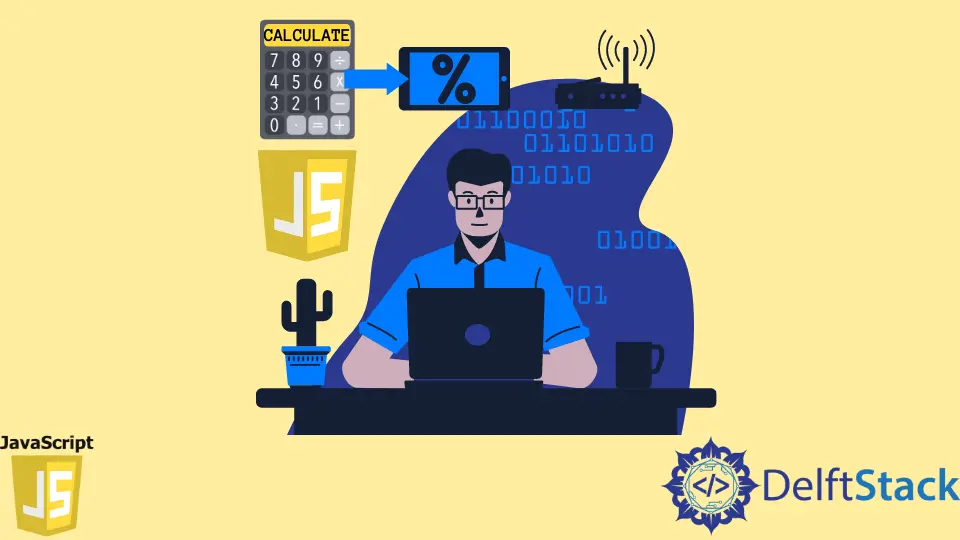
Calculating percentages is a common task in programming, especially when dealing with numerical data. Whether you’re building a financial application, a grading system, or just need to analyze data, understanding how to calculate percentages in JavaScript can be incredibly useful.
In this article, we’ll explore different methods to calculate percentages using JavaScript. We’ll break down each method, provide clear examples, and explain how everything works in a straightforward manner. By the end of this article, you’ll have a solid grasp of percentage calculations in JavaScript, empowering you to implement these techniques in your own projects.
Method 1: Basic Percentage Calculation
The simplest way to calculate a percentage in JavaScript is by using a basic formula. The formula for calculating a percentage is straightforward: divide the part by the whole and then multiply the result by 100. This method is particularly useful for quick calculations without the need for complex functions.
Here’s how you can implement this:
function calculatePercentage(part, whole) {
return (part / whole) * 100;
}
const part = 25;
const whole = 200;
const percentage = calculatePercentage(part, whole);
console.log(percentage);
Output:
12.5
In this example, we define a function called calculatePercentage
that takes two arguments: part
and whole
. The function divides the part by the whole, resulting in a decimal, and then multiplies that decimal by 100 to convert it into a percentage. When we call the function with a part of 25 and a whole of 200, it returns 12.5, indicating that 25 is 12.5% of 200. This method is quick and efficient for basic percentage calculations.
Method 2: Using a Percentage Object
For more complex applications, you might want to create an object that encapsulates the percentage calculation logic. This approach can make your code more organized and reusable, especially in larger projects.
Here’s a simple implementation:
const PercentageCalculator = {
calculate(part, whole) {
return (part / whole) * 100;
},
display(part, whole) {
const percentage = this.calculate(part, whole);
return `${part} is ${percentage}% of ${whole}`;
}
};
const result = PercentageCalculator.display(50, 400);
console.log(result);
Output:
50 is 12.5% of 400
In this example, we define a PercentageCalculator
object that contains two methods: calculate
and display
. The calculate
method performs the percentage calculation, while the display
method formats the output. When we call PercentageCalculator.display(50, 400)
, it returns a string that clearly states the percentage relationship between the two numbers. This method is particularly useful for applications where you need to display the results in a user-friendly manner.
Method 3: Percentage Change Calculation
Another common scenario is calculating the percentage change between two values. This is especially useful in financial applications or data analysis where you want to understand how much a value has increased or decreased.
Here’s how you can calculate percentage change:
function calculatePercentageChange(oldValue, newValue) {
const change = newValue - oldValue;
return (change / oldValue) * 100;
}
const oldValue = 50;
const newValue = 75;
const percentageChange = calculatePercentageChange(oldValue, newValue);
console.log(percentageChange);
Output:
50
In this example, the calculatePercentageChange
function takes two arguments: oldValue
and newValue
. It calculates the change by subtracting the old value from the new value and then divides that change by the old value. Finally, it multiplies by 100 to get the percentage change. When we call the function with an old value of 50 and a new value of 75, it returns 50, indicating a 50% increase. This method is vital for understanding trends in data and making informed decisions based on percentage changes.
Conclusion
Understanding how to calculate percentages in JavaScript is an essential skill for any developer. Whether you’re performing basic calculations, creating reusable objects, or analyzing percentage changes, the methods outlined in this article provide a solid foundation. By implementing these techniques, you can easily handle percentage calculations in your projects, making your code more efficient and effective. Remember, practice makes perfect, so don’t hesitate to experiment with these examples in your own JavaScript applications!
FAQ
-
How do I calculate a percentage in JavaScript?
You can calculate a percentage by dividing the part by the whole and multiplying by 100. -
Can I create a reusable function for percentage calculations?
Yes, you can create a function that takes parameters for the part and whole values to calculate percentages. -
What is percentage change, and how do I calculate it?
Percentage change measures how much a value has increased or decreased relative to its original value. It is calculated by dividing the change by the old value and multiplying by 100. -
Are there any libraries that can help with percentage calculations in JavaScript?
While you can perform percentage calculations with plain JavaScript, libraries like Lodash can simplify complex data manipulations. -
How can I display the percentage result in a user-friendly format?
You can create a function that formats the output as a string, clearly indicating the relationship between the part and whole values.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn