How to Convert Radians to Degrees in JavaScript
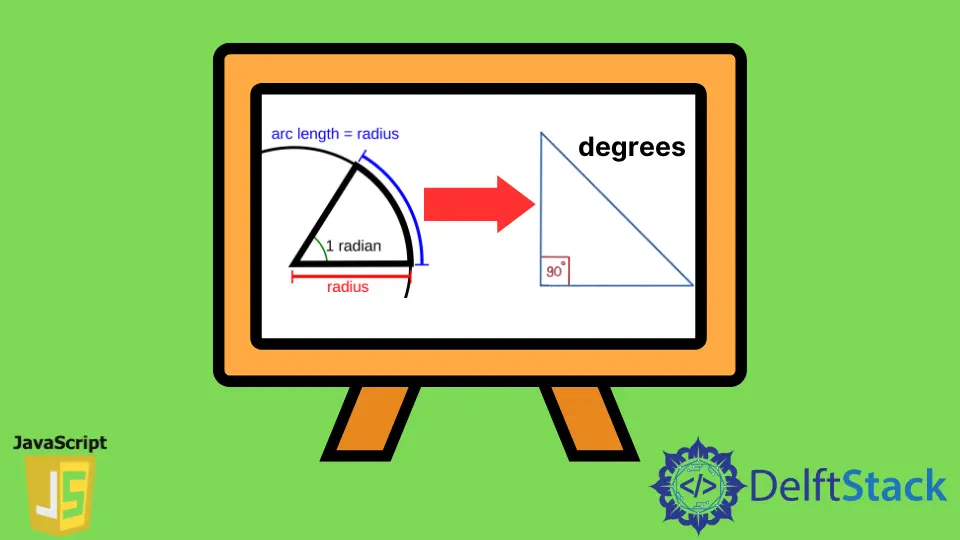
In this article, we will learn Mathematical calculations in JavaScript source code to get the degree value from radian value using formula and using the default alert box or log box to display the result to the user.
Convert Radians to Degrees in JavaScript
In JavaScript, we can perform arithmetic operations and calculations like addition, subtraction, multiplication, and divisions using arithmetic operators (+
, -
, *
, /
). And with the help of these operators, we can create any mathematical formula in a JavaScript program.
To convert radian to a degree in JavaScript, we can calculate and find out the degree value using the formula which is:
radian value * (half circle angle/value of pi )
In JavaScript, there is a default Math.PI
keyword for the pi value, which returns 3.14. This default value is useful and efficient in our radians to degrees conversion formula rather than writing 3.14.
Basic Formula:
let radian = 1
let degree = radian * (180 / Math.PI);
As shown above, we only need a pre-defined radian value from the user to determine the degree value. We can get the default Math.PI
value which is 3.14.
Example:
In the following example, we will get the radian value from user input and perform the arithmetic operation with the radian to degree formula. We will display the result to the user in an alert box.
<html>
<head>
<title> convert radian to degree </title>
</head>
<script>
function convertToDegree()
{
let data = document.getElementById("value").value;
data = parseInt(data)
if(isNaN(data)) //check empty data field
{
alert("Please enter value");
}
else{
let degree = data * (180 / Math.PI); //Math.PI = 3.14
degree = degree.toFixed(5)
alert("Converted value into degree : "+degree+"°");
}
}
</script>
<body>
<h1 style="color:blueviolet">DelftStack Learning</h1>
<h3>JavaScript Convert radian to degree</h3>
<form onsubmit ="convertToDegree()">
<!-- data input -->
<td> Enter Radian ualue: </td>
<input id = "value" type="number">
<br><br>
<input type = "submit" value = "Convert">
</form>
</body>
</html>
Output:
We used the form element in the above HTML source code to create user input fields and the submit
button. The user will insert the radian value, and when the user clicks the submit
button, the function convertToDegree()
will trigger.
In the script tags, we have declared the convertToDegree()
function. Inside that function, we got the user input value in the data
variable and used the conditional statement if
to check the input values.
If the variable is empty or contains no value, it will display the alert()
function with an error message.
If the user provides the value, we perform the arithmetic operation in the else
condition using the radians to degrees conversion formula and store the result in the degree
variable. We round the float value to 5 digits and display the result in the alert()
popup.