JavaScript で数値を最も近い 10 に丸める
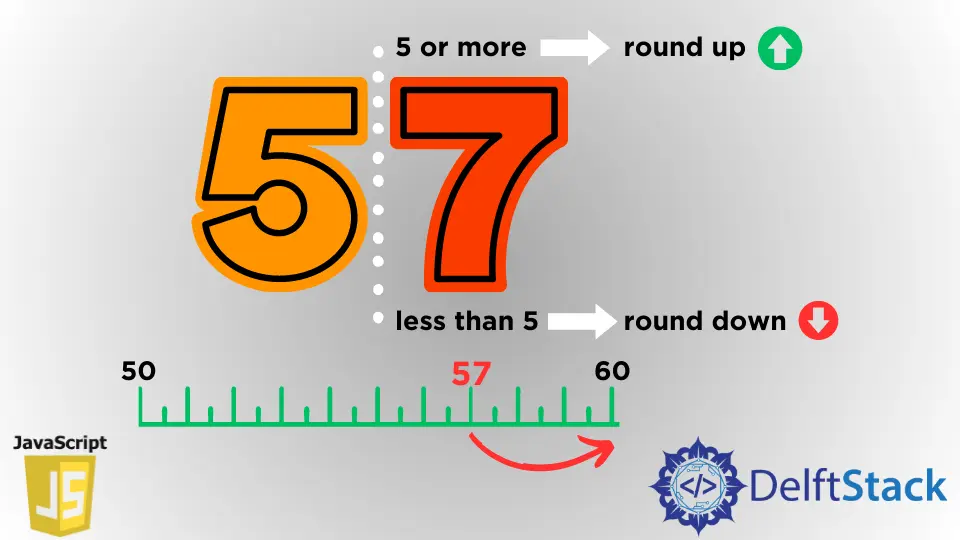
JavaScript には、数値を丸めるためのネイティブ メソッドがあります。 たとえば、数値を最も近い 10
に丸めるには、純粋な JavaScript 関数 Math.ceil()
を追加の除算と乗算と共に使用できます。
同様に、数値を最も近い 10
に丸めるには、純粋な JavaScript 関数 Math.round()
を追加の除算と乗算と共に使用できます。
この JavaScript 記事では、Math.ceil()
および Math.round()
関数を使用して、数値を切り上げる方法、または最も近い 10 に丸める方法を学びます。
Math.ceil()
を使用して、JavaScript で数値を最も近い 10 に丸める
数値を最も近い 10
の倍数に丸めるために、Math.ceil()
関数を呼び出すことができます。パラメータとして数値を 10
で割り、その結果に 10
を掛けます。例えば、Math.ceil(num / 10) * 10
となります。
Math.ceil
関数は、数値を次に大きい整数に丸め、結果を返します。
function roundUpNearest10(num) {
return Math.ceil(num / 10) * 10;
}
console.log(roundUpNearest10(101)); // 👉️ 110
console.log(roundUpNearest10(89.9)); // 👉️ 90
console.log(roundUpNearest10(80.01)); // 👉️ 90
console.log(roundUpNearest10(-59)); // 👉️ -50
console.log(roundUpNearest10(-60)); // 👉️ -60
以下のスクリーンショットは、上記のコードの出力を示しています。
Math.ceil
関数は、ユーザーの面倒な作業をすべて処理します。 たとえば、渡された数値に小数部分がある場合、Math.ceil
関数は数値を切り上げます。
一方、整数が渡された場合、関数は数値を返します。 以下は、Math.ceil
関数の使用例です。
console.log(Math.ceil(6.01)); // 👉️ 7
console.log(Math.ceil(61.00001)); // 👉️ 62
console.log(Math.ceil(60)); // 👉️ 60
console.log(Math.ceil(-33.99)); // 👉️ -33
console.log(Math.ceil(null)); // 👉️ 0
以下のスクリーンショットは、上記のコードの出力を示しています。
Math.ceil
関数が null
値で呼び出されると、0
が返されます。 コード例の段階的なプロセスを以下に示します。
console.log(31 / 10); // 👉️ 3.1
console.log(50 / 10); // 👉️ 5
console.log(Math.ceil(31 / 10)); // 👉️ 4
console.log(Math.ceil(50 / 10)); // 👉️ 5
console.log(Math.ceil(41 / 10) * 10); // 👉️ 50
console.log(Math.ceil(60 / 10) * 10); // 👉️ 60
以下のスクリーンショットは、上記のコードの出力を示しています。
コードには 2つのステップがあります。
- 数値を
10
で割り、結果を次に大きい整数に丸めます。 - 結果に
10
を掛けて、最も近い10
に切り上げられた数値を取得します。
Math.round
を使用して、JavaScript で数値を最も近い 10 に丸める
数値を最も近い 10
に丸めるには、Math.round()
関数を呼び出して、10
で割った数値を渡し、その結果に 10
を掛けます (例: Math.round(num / 10) \* 10
.
Math.round
関数は数値を受け取り、それを最も近い整数に丸め、結果を返します。
function roundToNearest10(num) {
return Math.round(num / 10) * 10;
}
console.log(roundToNearest10(34)); // 👉️ 30
console.log(roundToNearest10(35)); // 👉️ 40
console.log(roundToNearest10(64.9)); // 👉️ 60
console.log(roundToNearest10(-36)); // 👉️ -40
console.log(roundToNearest10(-35)); // 👉️ -30
以下のスクリーンショットは、上記のコードの出力を示しています。
Math.round
関数は、ユーザーの面倒な作業をすべて処理します。 この関数は、数値を最も近い整数に丸めます。
一方、数値の小数部分が正確に 0.5
の場合、数値は正の無限大方向に次の整数に丸められます。
以下は、Math.round
関数の使用例です。
console.log(Math.round(8.49)); // 👉️ 8
console.log(Math.round(8.5)); // 👉️ 9
console.log(Math.round(80)); // 👉️ 80
console.log(Math.round(-84.5)); // 👉️ -84
console.log(Math.round(-84.51)); // 👉️ -85
console.log(Math.round(null)); // 👉️ 0
以下のスクリーンショットは、上記のコードの出力を示しています。
Math.round
関数は、null
値で呼び出されると 0
を返します。 以下に例を示し、ステップバイステップで説明します。
console.log(65 / 10); // 👉️ 6.5
console.log(44 / 10); // 👉️ 4.4
console.log(Math.round(65 / 10)); // 👉️ 7
console.log(Math.round(44 / 10)); // 👉️ 4
console.log(Math.round(65 / 10) * 10); // 👉️ 70
console.log(Math.round(44 / 10) * 10); // 👉️ 40
以下のスクリーンショットは、上記のコードの出力を示しています。
これは 2 段階のプロセスです。
- 数値を
10
で割り、結果を最も近い整数に丸めます。 - 結果に
10
を掛けて、最も近い10
に丸められた数値を取得します。
したがって、この JavaScript 記事の助けを借りて、ユーザーは Math.ceil()
および Math.round()
関数を使用して数値を切り上げる方法、または最も近い 10 に丸める方法を学習しました。