How to Format Phone Number in JavaScript
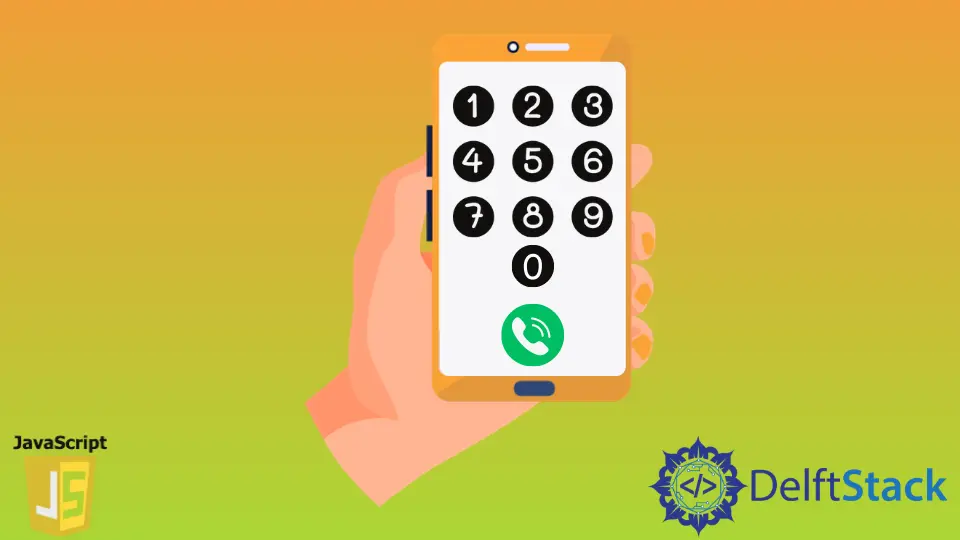
In this article, we will learn the best way to format the phone number in JavaScript source code and the benefits of formatted numbers in our JavaScript code.
Phone Number Format in JavaScript
In JavaScript, we have multiple options to perform number formatting effectively. Mostly, developers use regular expression and the default substr()
method for number formatting in applications.
Use Regular Expression
We use RegEx to replace the original phone number with a human-readable format. For example, the regular expression pattern /(\d{3})(\d{3})(\d{4})/,'$1-$2-$3'
can be used with replace method to separate the first three digits, next three digits and last four digits and concatenate them with -
among them at the end.
Basic Syntax:
let result = phoneNo.replace(/(\d{3})(\d{3})(\d{4})/, '$1-$2-$3');
Example Code:
function formatNumber(number) {
console.log('Original number : ' + number);
let result = number.replace(/(\d{3})(\d{3})(\d{4})/, '$1-$2-$3');
console.log('Formatted number using regular expression : ' + result);
}
var originalNumber = '4445556678';
formatNumber(originalNumber)
Output:
"Original number : 4445556678"
"Formatted number using regular expression : 444-555-6678"
In the above JavaScript source example, we created a function formatNumber()
, which takes the number as a parameter. Inside that function, we print the original passed number in logs using console.log()
.
Then, we used the default replace()
method of string on passed numbers with a regular expression pattern. The output is stored in a separate variable and displayed in console.log()
.
We just initialized the number string in the original format and passed that number in the function formatNumber()
.
Use the substr()
Method in JavaScript
This default string method, substr()
, can be used to format the number. We can divide the number string into three different portions, and then we can concatenate all the portions with -
among them.
Basic Syntax:
let data = 'hello world'
let result = data.substr(0, 5) // it will separate "hello" from hello world
Example Code:
function formatNumber(number) {
console.log('Original number : ' + number);
let result = number.substr(0, 3) + '-' + number.substr(3, 3) + '-' +
number.substr(6, 4);
console.log('Formatted number using substring method : ' + result);
}
var originalNumber = '6665559978';
formatNumber(originalNumber)
Output:
Original number : 6665559978
Formatted number using substring method : 666-555-9978
In the above JavaScript source example, we used the substr()
method on the passed number to divide into portions and used concatenation with the +
operator to join the string again with -
.
The output is stored in a separate variable and displayed in console.log()
, and passed the number in function formatNumber()
.