How to Check if Number Is Between Two Values in JavaScript
- Check if a Number Is Between Two Values Using Comparison and Logical Operators in JavaScript
-
Use Comparison and Logical Operator With the
if
Statement in JavaScript -
Use Comparison and Logical Operator With the Ternary Operator
?:
in JavaScript
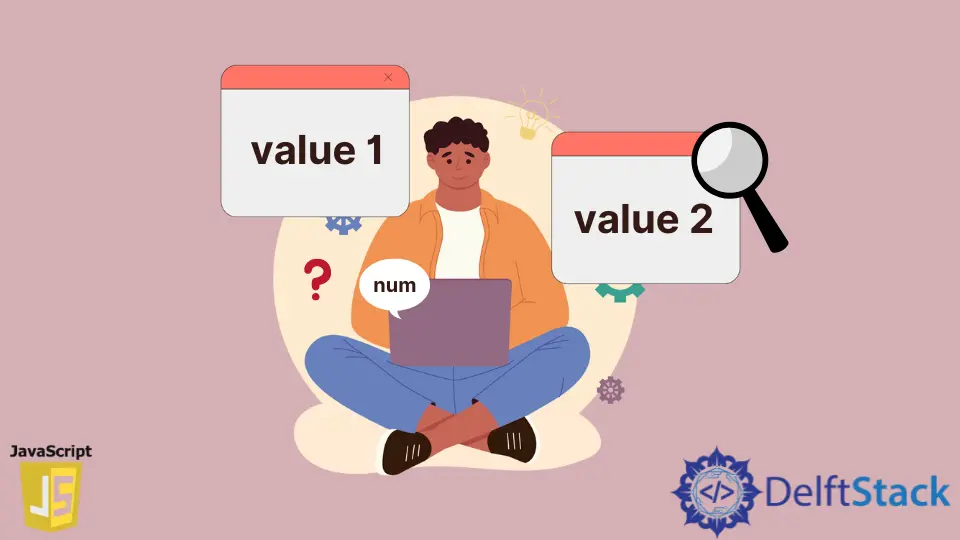
In this article, we will see how to check if a number is between two values in JavaScript. We will be using the comparison operator and the logical operators to achieve this.
We can use these operators either with the if
statement or the ternary operator ?:
in JavaScript.
For example, if you have a number, say 25, and you want to check if this number falls within a range of numbers 10 and 50 or not. Now you can see that the number 25 exist between the number 10 and 50.
We will see how to implement this in code.
Check if a Number Is Between Two Values Using Comparison and Logical Operators in JavaScript
There are various types of operators in JavaScript, out of which we will be dealing with only the comparison and logical operators.
The comparison operator allows you to compare two operands and returns true
if the condition satisfies and false
otherwise. The operands can be numerical, string, logical, or object values.
The comparison operator includes greater than >
, less than <
, equal ==
, less than or equal <=
, and many more. You can read the comparison operator’s documentation to get a full list of available operators within the comparison operator.
Out of these operators, we will use the greater than >
and less than <
operators to check if the number exists between two values.
Another operator that we will be using is the logical operator.
There are three logical operators: logical AND
&&
, logical OR
||
, and logical NOT
!
. And we will be using the logical AND
&&
operator.
These operators are typically used with Boolean values. Since the comparison operator provides a Boolean value, we can use the logical operator.
These operators can be used with either the if
statement or the ternary operator ?:
in JavaScript. Let’s now see how to implement this in practice.
Use Comparison and Logical Operator With the if
Statement in JavaScript
First, we will see how to use the comparison operator and the logical operator with the if
statement, and later we will see how to use them with the ternary operator ?:
. The logic and the code will remain the same for both of them, but there will be a slight difference in the syntax.
Here, we have created a function called numberInBetween()
that will take three parameters; the initial two parameters, startingNumber
and endingNumber
, will be the range of numbers, i.e., 10 and 50. And the third parameter, givenNumber
, is the number itself, i.e., 25, that we want to check if it falls within the range 10 and 50 or not.
Code Snippet - JavaScript:
function numberInBetween(startingNumber, endingNumber, givenNumber) {
if (givenNumber > startingNumber && givenNumber < endingNumber)
console.log(`Given number ${givenNumber} falls between ${
startingNumber} and ${endingNumber}`);
else
console.log('Given number does not fall between within the given range');
}
numberInBetween(10, 50, 25);
Output:
Inside the numberInBetween()
function, we have the if
statement. Here, we check if the givenNumber
is greater than the startingNumber
or not and if the givenNumber
is less than the ending number.
Suppose both these statements present on the left and right side of the &&
operator return true
. In that case, the overall expression will also be true
, and then we can enter the if
statement and print a message to the console that the given number falls between the two values.
If any one of the statements gives a false
value, then the overall expression’s value will result in false
, and in such a case, we will enter the else
statement.
Use Comparison and Logical Operator With the Ternary Operator ?:
in JavaScript
The if
statement and the ternary operator work the same way. The advantage of the ternary operator is that its syntax is concise and easy to read.
While using the ternary operator, we exclude the if
and else
keywords instead, using the ?
and :
operators. The ?
is used after the condition, and the :
is used within the two statements below.
Here, also you will get the same output.
Code Snippet - JavaScript:
function numberInBetween(startingNumber, endingNumber, givenNumber) {
(givenNumber > startingNumber && givenNumber < endingNumber) ?
console.log(`Given number ${givenNumber} falls between ${
startingNumber} and ${endingNumber}`) :
console.log('Given number does not fall between within the given range');
}
numberInBetween(10, 50, 25);
As you can see, we have a single console statement before and after the :
symbol. So, if the condition satisfies, then the statement which is present to the left of the :
symbol will be executed, and if the condition does not satisfy, then the statement which is present to the right of the :
symbol will be executed.
If you need to execute a single statement after a condition is satisfied, use the ternary operator; use the if
statement if you need to execute multiple statements after a condition is satisfied.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn