How to Format a Number in JavaScript
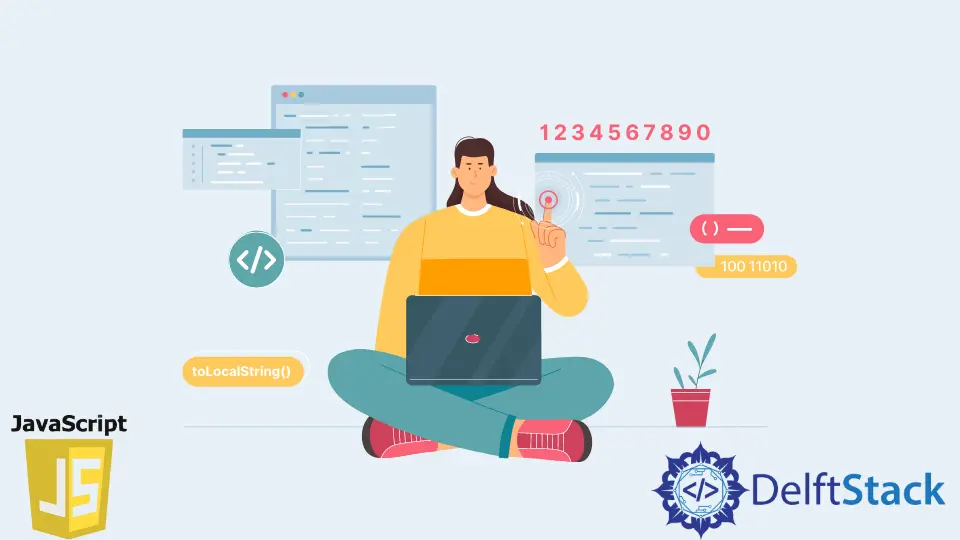
There are various ways people from different countries and regions follow different ways of formatting a number. And there could be cases where we want to format a number on our websites based on which part of the world the visitor accesses our website.
It could become challenging if you try to do this manually based on the user’s location. But no worry, you don’t have to do it manually since there is a better way of formatting a number in JavaScript, and that is with the help of the toLocalString()
method. This method returns a string with the language-specific representation of the number.
Format Numbers With the toLocaleString()
Method in JavaScript
It takes two parameters first is locales
, and the second is options
. Both these parameters are optional, meaning it’s not mandatory. You can directly run the method without passing those parameters as well. If you want to format the numbers, in this case, we do, then we need to pass those parameters.
Let’s first understand what each of these parameters means, and then later, we will see how to use them in our code.
1. The locales
Parameter
The locales
is the first parameter which the toLocalString()
method accepts. It represents a string of BCP 47 language tags or an array of such strings. The BCP 47 language tag is a code to identify human languages.
For example, the tag ar-SA
says that it is for the Arabic language represented by ar
, spoken in the region of Saudi Arabia SA
. So, If you pass this string as the first argument, it will format the number so that it is written in the Arabic language.
let eArabic = (number => {
return number.toLocaleString('ar-SA');
});
console.log(eArabic(12345));
Output:
١٢٬٣٤٥
Here, we have created an arrow function called eArabic
, which takes a number as a parameter. Now, we are passing the number 12345
to this arrow function. This function aims to convert the number 12345
into its Arabic equivalent format ar-SA
with the help of the toLocalString()
method.
You can directly copy and paste the entire code above and run it in your browser’s console window to see the output.
To learn more about all the BCP 47 languages tags, you can take a look at this resource.
You can also pass undefined
as locales. It will take the default language that is set in the visitor’s browser. So, If someone has changed the language of their browser to German, this will show the number in German format.
2. The options
Parameter
The options
is an object, and it is the second parameter which the toLocalString()
method accepts. Since it’s an object, this parameter can take various properties like currency
, currencySign
, style
, unitDisplay
, and many more.
Let’s take the same example which we have taken above, and let’s try to modify it to understand the options
parameter. Previously, We just take the number 12345
and then format it to the Arabic language. Now let’s also give the number a currency formatting by giving it a style of currency
with currency symbol and add some fraction digits at the end of that number with the help of options
.
let eArabic = (number => {
return number.toLocaleString(
'ar-SA', {style: 'currency', currency: 'SAR', minimumFractionDigits: 3});
});
console.log(eArabic(12345));
Output:
١٢٬٣٤٥٫٠٠٠ ر.س
In this case, to the toLocaleString()
method, we have now also added the options
parameter, which is an object. Currently, it takes 3 properties, the style
property will format the number in currency format, the currency
property will tell which currency it represents, in this case, Saudi Riyal SAR
and the minimumFractionDigits
property represents how many fractional digits you want to show at the end of the number.
Here, if you are setting the style
property with the value currency, you also have to set the property currency
with some value. Otherwise, you will get a type error: Currency code is required with currency style.
In the output of the above program, we first have the currency name Saudi Riyal ر.س.
. If it was USD, then it would be the $
symbol. Then we have the number itself in Arabic language ١٢٬٣٤٥
. If it was in USD, then it would be 12,345
. At last, we have the 3 fractional digits after the dot which is represented in the Arabic language as ٫٠٠٠
and USD as .000
.
// USD format
$12, 345.000
Here, we are also providing you with the output for the United States currency format to easily relate it with the output of the Saudi Arabian currency format since it might be difficult for people to understand the Arabian language.
You can use any of these parameters and object properties in your code depending upon how you want to format your number. You can also visit the various links provided in this article to learn more about the toLocalString()
to format a number in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn