JavaScript Number toLocaleString() Method
-
Syntax of JavaScript Number
toLocaleString()
Method -
Example 1: Use the
number.toLocaleString()
Method Without Parameters -
Example 2: Use the
number.toLocaleString()
to Convert Number to SpecificLocales
-
Example 3: Use the
number.toLocaleString()
Method With Array ofLocales
-
Example 4: Use the
number.toLocaleString()
WithOptions
Parameter to Format the Output
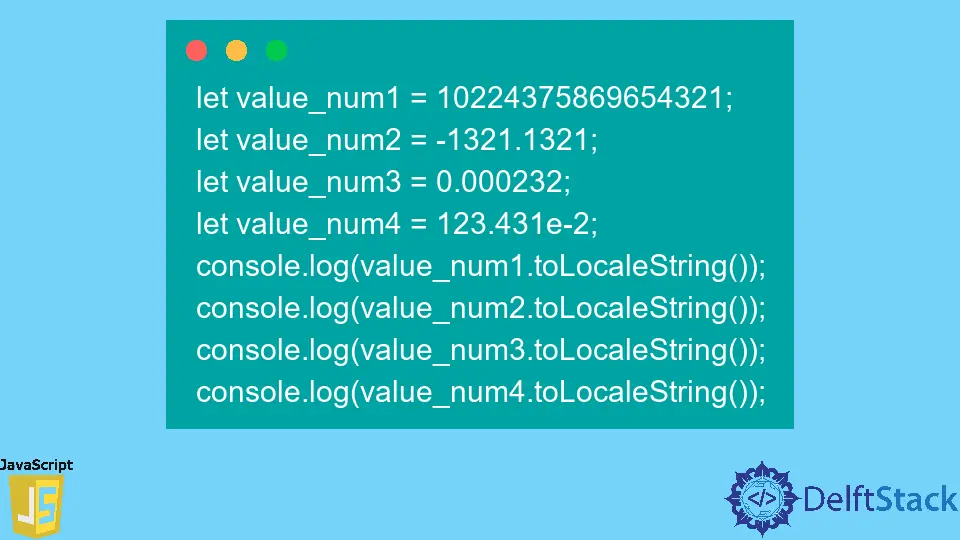
We can use the number.toLocaleString()
method to format the number string according to a specific locale. The simple meaning of the locale is the language of a particular region.
So, every language has a different format for writing numbers, and that is what we can achieve using the number.toLocaleString()
method.
Syntax of JavaScript Number toLocaleString()
Method
let num = 987651213;
num.toLocaleString();
num.toLocaleString(locales);
num.toLocaleString(locales,options);
Parameters
locales |
It is an optional parameter. The locales is the string or array of strings representing the language tags to convert the number to specific locales . |
options |
It is also an optional parameter. The options is used to format the output properly, and it can contain hundreds of properties. |
Return
The Number.toLocaleString()
method returns the number string after converting the number value to a particular locale and formatting according to the options
.
Example 1: Use the number.toLocaleString()
Method Without Parameters
When we don’t pass any parameter to the number.toLocaleString()
method, it formats the number according to the default locale.
In the example below, we have taken the different numbers and invoked the toLocaleString()
method by taking them as a reference. Also, we have used the exponential notion of the number in the value_num4
.
let value_num1 = 10224375869654321;
let value_num2 = -1321.1321;
let value_num3 = 0.000232;
let value_num4 = 123.431e-2;
console.log(value_num1.toLocaleString());
console.log(value_num2.toLocaleString());
console.log(value_num3.toLocaleString());
console.log(value_num4.toLocaleString());
Output:
10,224,375,869,654,320
-1,321.132
0
1.234
Example 2: Use the number.toLocaleString()
to Convert Number to Specific Locales
When we pass the Locales
as a parameter to the number.toLocaleString()
method, it represents the number in the regional language.
Here, we have created the single number variable and used the different locales
to represent it in the different regional languages. In this example, we have used the locale of Netherlands
, India
, and USA
.
let value_num1 = 10224375869654321;
console.log(value_num1.toLocaleString('nl-NL'));
console.log(value_num1.toLocaleString('en-IN'));
console.log(value_num1.toLocaleString('en-US'));
Output:
10.224.375.869.654.320
10,22,43,75,86,96,54,320
10,224,375,869,654,320
Example 3: Use the number.toLocaleString()
Method With Array of Locales
Rather than using the single locale as a parameter, we can use the array of locales
as a parameter.
When the method cannot format the number object according to the first locale, it will format the number according to the second locale, and so on. In the array of locales
, priority is from start to end.
In the below example, we have printed the number according to the Netherlands and India’s locales. After that, we passed the array of locales to the number.toLocaleString()
method, which contains the wrong locale tag of Netherlands
, and after that right locale code for India
.
So, in the output, users can see that it formats the number according to India’s locale.
let value1 = 3421231;
console.log(value1.toLocaleString('nl-NL'));
console.log(value1.toLocaleString('en-IN'));
console.log(value1.toLocaleString(['nlp-NL','en-IN']));
Output:
3.421.231
34,21,231
34,21,231
Example 4: Use the number.toLocaleString()
With Options
Parameter to Format the Output
The options
parameters allow us to format the output. For example, we can format the number as a currency and the digits of the number.
In this example, we have taken a single number and formatted it as a Euro
and Indian Rupee
currency. Users can also see the symbols of the currency in the output.
let value1 = 6434;
let options = { style: 'currency', currency: 'EUR' }
console.log(value1.toLocaleString('nl-NL',options));
options.currency = 'INR';
console.log(value1.toLocaleString('en-IN',options));
Output:
€ 6.434,00
₹6,434.00
When we don’t pass any locales
to the number.toLocaleString()
method, it gives you the output according to your computer’s default language. Users can try various locales and get different number strings according to regions.