JavaScript Number.isFinite() Method
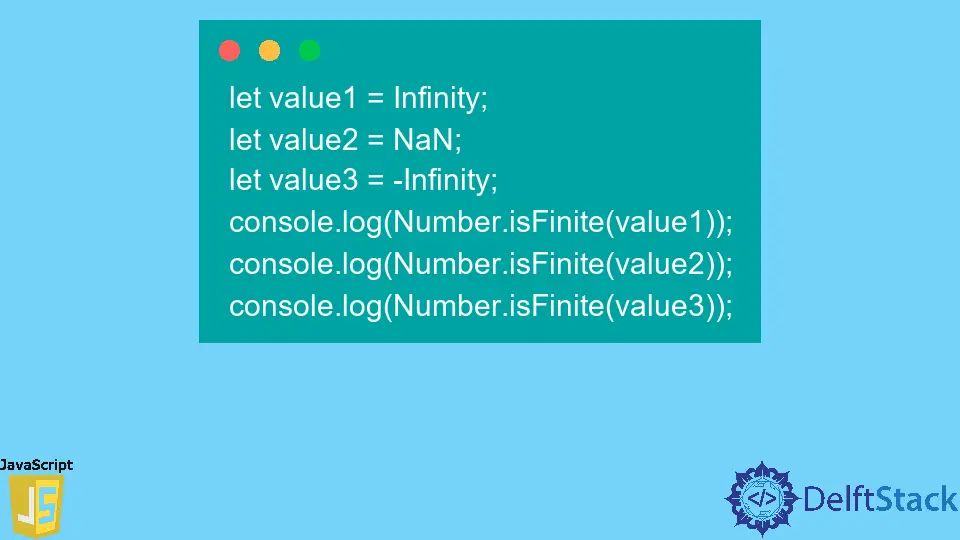
The number.isFinite()
method determines whether the specified argument for this method is a number or not. Also, if the value is a number, it checks whether the number is finite or not.
In JavaScript, all numeric values are finite except Positive Infinity
, Negative Infinity
, and NaN
.
Syntax
let num = 10l;
Number.isFinite(num);
Parameters
num |
The num is the number or any variable value for which we need to check whether it is finite or not. |
Returns
It returns true
and false
Boolean values based on whether the number is finite or infinite.
Example Codes
Let’s learn the use of Number.isFinite()
method using different input values.
Use the Integer Values as a Parameter for Number.isFinite()
If we pass the integer values as a parameter to the Number.isFinite()
method, it always returns the true
.
The following code fence declares two variables and assigns them positive and negative integer values. This code returns true
or false
as both positive and negative values are numeric. See the output for this code snippet below.
let referenceNumber = 300;
let referenceNumber2 = -300;
console.log(Number.isFinite(referenceNumber));
console.log(Number.isFinite(referenceNumber2));
Output:
true
true
Use the Float Values as a Parameter for Number.isFinite()
If we pass the floating point values as a parameter to the Number.isFinite()
method, it always returns the true
.
We have declared two variables in the code fence below and assigned the positive and negative floating point values. We can see that it returns true
as output because both positive and negative values are numeric.
let referenceNumber = 300.50;
let referenceNumber2 = -150.75;
console.log(Number.isFinite(referenceNumber));
console.log(Number.isFinite(referenceNumber2));
Output:
true
true
Use null
& '1'
as the Parameter for Number.isFinite()
Note that, the Number.isFinite()
method returns false
if null
or a string type value such as '1'
is passed as a parameter. It is because the null
and '0'
are not the Number
type.
The following code fence declares two variables and assigns the positive and negative floating point values. In the outcome, we can see that it returns true
as both positive and negative values are numeric.
let referenceNumber = null;
let referenceNumber2 = '1';
console.log(Number.isFinite(referenceNumber));
console.log(Number.isFinite(referenceNumber2));
Output:
false
false
Use the Infinite & NaN
as a Parameter for Number.isFininte()
When we pass the Positive Infinity
, Negative Infinity
, or NaN
as a parameter to the Number.isFinite()
, it always returns the false
values, and we can see that in the output of the example below.
let value1 = Infinity;
let value2 = NaN;
let value3 = -Infinity;
console.log(Number.isFinite(value1));
console.log(Number.isFinite(value2));
console.log(Number.isFinite(value3));
Output:
false
false
false
The Number.isFinite()
method is compatible with all modern browsers, and we can use it to check if the given number is a finite number value.