JavaScript Number.toExponential() Method
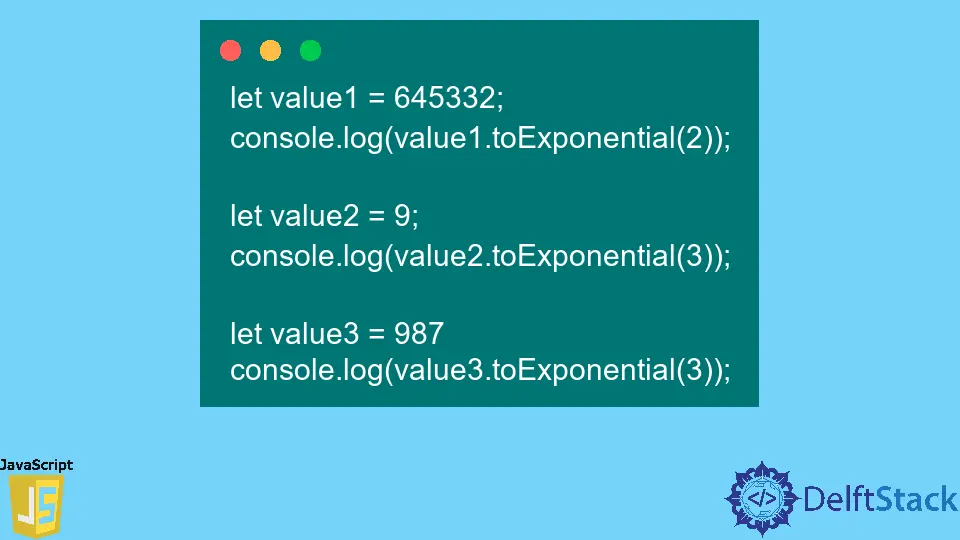
In JavaScript, we use the Number.toExponential()
method to get the exponential notation of the number object, which helps us to represent the large numbers in a correctly readable format.
We can use this method with/without specifying the x
parameter, which we will see later in this tutorial.
The exponential notation of the number object means converting the number in terms of the power of 10
. For example, 1.23e+2
means 1.23*(10^2) = 123
, and here e+2
represents the 10^2
.
Syntax
let num = 12.32;
num.toExponential(x);
Parameters
x |
It is an optional parameter used to specify the number of digits between 0 and 20 after the decimal point in the exponential notation of the number. |
Returns
The Number.toExponential()
returns the number’s exponential notation (1
digit before the decimal point and x
digits after a decimal point) in the string format.
Example Codes
Let’s explore different code examples with/without specifying the x
parameter, which must be between 0
and 20
, representing the number of digits required after a decimal point.
Use Number.toExponential()
Without x
Parameter
The following code accepts three different numeric values and converts them to exponential notation. We haven’t passed the x
parameter, so it will show the default number of digits after the decimal point to represent the number uniquely.
let num1 = 12342;
console.log(num1.toExponential());
let num2 = 2;
console.log(num2.toExponential());
let num3 = 423
console.log(num3.toExponential());
Output:
"1.2342e+4"
"2e+0"
"4.23e+2"
Use Number.toExponential()
With x
Parameter
We have passed the x
as a parameter of the Number.toExponential()
method. It will round up the number and show the exact x
number of digits after the decimal point.
If the value of x
is larger than the total digits in the number object, Number.toExponential(x)
will add zeros at the end of the number, which we can observe in the output of the example below.
let value1 = 645332;
console.log(value1.toExponential(2));
let value2 = 9;
console.log(value2.toExponential(3));
let value3 = 987
console.log(value3.toExponential(3));
Output:
"6.45e+5"
"9.000e+0"
"9.870e+2"