JavaScript Number toFixed() Method
-
Syntax of JavaScript Number
toFixed()
Method -
Example 1: Use the
number.toFixed()
Method to Remove Decimal Part From Numbers -
Example 2: Use the
number.toFixed()
Method to Round the Number to a FixedDecimals
Point -
Example 3: Use the
number.toFixed()
Method to Add Padding to Decimal Digits -
Example 4: Use the
number.toFixed()
Method to Round the Number Represented via Exponential Notation - Exceptions
-
Example 5: Use the
number.toFixed()
Method With Non-Numeric Values
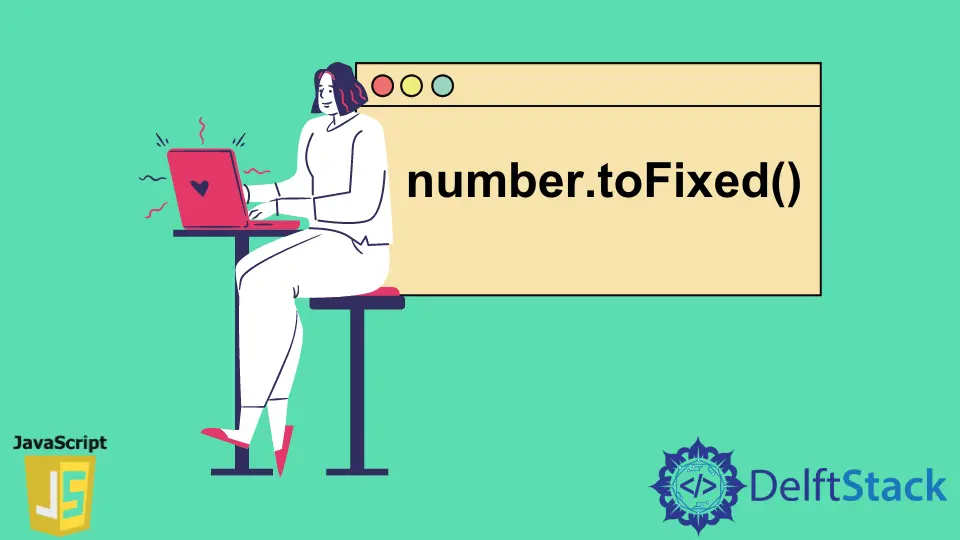
The number.toFixed()
method is used to round the number to the specific decimal point.
The number.toFixed()
method converts the number to a string and increases or decreases the decimal point notations according to the value of the decimals
parameter.
Syntax of JavaScript Number toFixed()
Method
let number = 302;
number.toFixed();
number.toFixed(decimals);
Parameters
decimals
- an optional parameter; representing the number of digits you want to keep after the decimal point. The default value of decimals
is 0.
Return
It returns the string representing the number after rounding a number to the decimals
decimal point.
Example 1: Use the number.toFixed()
Method to Remove Decimal Part From Numbers
When we don’t pass the decimals
parameter, it takes its default value of 0 and rounds down the number after removing the decimal part of the number.
In the example below, we have taken two numeric values and used the number.toFixed()
method to round them down. Also, we have checked the type of the positive_num
variable before invoking the number.toFixed()
method, which is the number
.
After invoking the method, its type changed to the string
that users can observe in the output.
let positive_num = 20.4352;
let negative_num = -6535.42424;
console.log(typeof positive_num);
positive_num = positive_num.toFixed();
console.log(positive_num);
console.log(negative_num.toFixed());
console.log(typeof positive_num);
Output:
number
20
-6535
string
Example 2: Use the number.toFixed()
Method to Round the Number to a Fixed Decimals
Point
In this example, we have used the decimals
optional parameter with the number.toFixed()
method. In the output, users can see that string of numbers contains the exact decimals
digits after the decimal point.
let positive_num = 20.4352;
let negative_num = -6535.42424;
positive_num = positive_num.toFixed(2);
console.log(positive_num);
console.log(negative_num.toFixed(4));
Output:
20.44
-6535.4242
Example 3: Use the number.toFixed()
Method to Add Padding to Decimal Digits
If users want to add padding to decimal places, they should pass the larger value of decimals
than the total number of decimal digits given in the current number.
Let’s understand it by the below example. The positive_value
contains 0 decimal digits.
So, we should pass the decimals
value greater than 0, and it will add the total (decimals-0)
number of zeros at the end. In the output, users can see how we have added the padding of 5 zeros after 20.
let positive_value = 20;
let negative_value = -32.12;
console.log(positive_value.toFixed(5));
console.log(negative_value.toFixed(4));
Output:
20.00000
-32.1200
Example 4: Use the number.toFixed()
Method to Round the Number Represented via Exponential Notation
The exponential notation of the number means the number is formatted with the e
. For example, we can write 435.32434342 to 4.3532434342e+2 in the exponential notation.
Here, e
represents the power of 10 that needs to multiply by the number.
In this example, we have taken different exponential numbers and rounded them to a fixed decimal point using the number.toFixed()
method.
let positive_value = 76.23e+0;
let negative_value = -3.76435321e+1;
console.log(positive_value.toFixed(0));
console.log(negative_value.toFixed(1));
Output:
76
-37.6
Exceptions
TypeError
- When we invoke the Number.toFixed()
method on the non-numeric values, it returns the TypeError
.
Example 5: Use the number.toFixed()
Method With Non-Numeric Values
When users try to use the number.toFixed()
method with non-numeric values, the method will always give the TypeError
.
We have to use the string, and NaN
values in the example below with the toFixed()
method and users can see in the output what error occurs.
let positive_value = "Delft";
let negative_value = NaN;
console.log(positive_value.toFixed(10));
console.log(negative_value.toFixed(2));
Output:
TypeError: positive_value.toFixed is not a function
We can use the number.toFixed()
method with any numeric values but can’t use it with non-numeric values as it gives the TypeError
. Also, when a user tries to round a large number, the method will return the output in the exponential notation.