How to Get User Input in JavaScript
- Getting User Input From the Browser Console
- Getting User Input From the NodeJS Console
-
Getting User Input From NodeJS Using
prompt-sync
Module
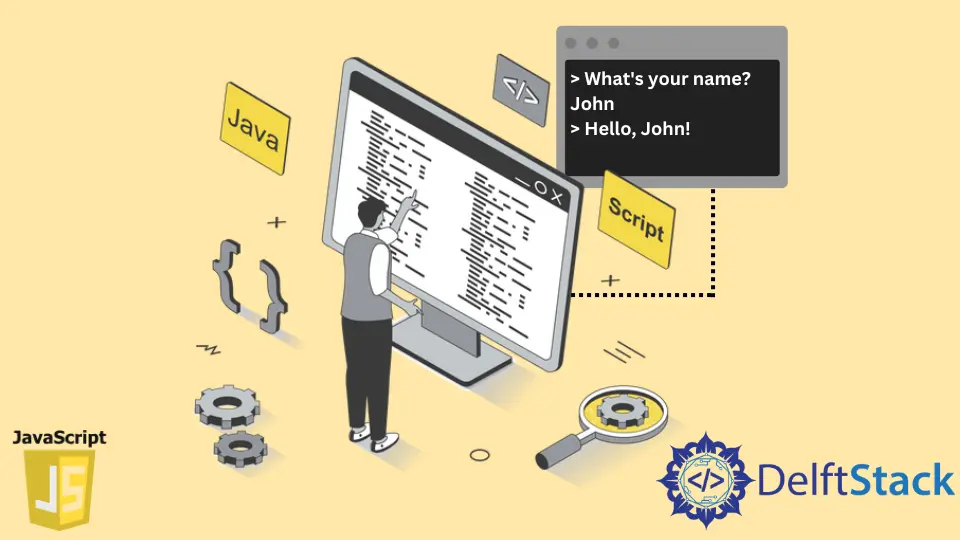
This article will help you understand how to get user input in JavaScript.
There are two ways to get user input in JavaScript, depending on whether you want input from the browser or NodeJS. This guide will help you learn both.
Getting User Input From the Browser Console
To require user input from the browser, you must use the prompt()
method provided by the browser. The method allows you to accept user input as a string and store it on a variable like so:
const input = prompt();
This method also accepts a string as additional information to know what input the application expects.
For example, the following code to prompt for a user name is written below:
const input = prompt('What\'s your name?');
alert(`Your name is ${input}`);
The alert()
method shows the result. You can style the line text as a question or hint per your requirement.
Getting User Input From the NodeJS Console
To accept user input from the NodeJS console, you must use the provided readline
module.
You can use the following commands like require()
from the module as shown below:
const readline = require('readline');
Then you need to instantiate the interface attached to the input stream. Use the readline.createInterface()
method to create an interface and pass input and output parameters as object arguments.
Writing input and output requires writing input to process.stdin
and output to process.stdout
.
Here is an example of creating a readline
interface.
const readline = require('readline');
const ql = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
The question()
method must be called on the interface instance assigned to the q1
variable in the code above to request user input.
The question()
method takes two parameters.
- String question you want to ask the user.
- A callback function to be executed when a response is received and the response is passed to the function
You can skip the parameter object and pass the callback function as the second parameter.
Finally, you can close the q1
interface by calling the q1.close()
method within the callback function.
arr.js
:
const readline = require('readline');
const q1 = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
q1.question("Where do you live? ", function (answer) {
console.log(`Oh, so you live in ${answer}`);
console.log("Interface Closed");
q1.close();
});
Getting User Input From NodeJS Using prompt-sync
Module
First, you need to install the prompt-sync
module using NPM or Yarn as follows:
npm install prompt-sync
or
yarn add prompt-sync
Then, you need the require()
to prompt-sync
module.
Take a look at the code below.
pm.js
:
const prompt = require('prompt-sync')();
const input = prompt('Where do you live? ');
console.log(`Oh, so you live in ${input}`);
Because this method is synchronous, the Node instance waits for input before executing the next line.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - JavaScript Event
- How to Call Multiple JavaScript Functions in Onclick Event
- How to Simulate a Click With JavaScript
- How to Add Onclick Event on HTML Image Tag in JavaScript
- How to Trigger Event in JavaScript
- How to Use Event Target in JavaScript
- Difference Between Event Bubbling and Capturing in JavaScript