How to Change Input Value in JavaScript
-
Change the Input Value Using the
value
Property in JavaScript -
Change the Input Value Using the
setAttribute()
Function in JavaScript
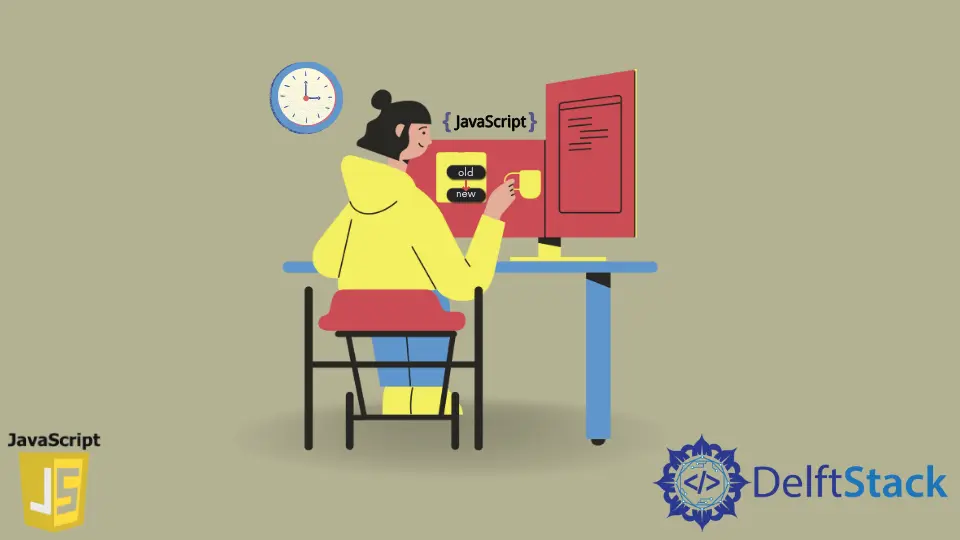
This tutorial will discuss changing the input value using the value
property or the setAttribute()
function in JavaScript.
Change the Input Value Using the value
Property in JavaScript
We use an input tag to get input from a user, and we can use the value property to change the input value. First of all, we need to get the element whose value we want to change using its id or name, and then we can use the value property to set its value to our desired value. To get an element in JavaScript, we can use the getElementById()
or the querySelector()
function. For example, let’s make a form with input and give it an id to get the element in JavaScript using the getElementById()
and set its value using the value
property. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<form>
<input type="text" id= "123" name="ABC" value="Some Value">
</form>
</body>
<script type="text/javascript">
var Myelement = document.getElementById("123");
console.log(Myelement.value);
Myelement.value = "New value";
console.log(Myelement.value);
</script>
</html>
Output:
Some Value
New value
In the above code, we used the document.getElementById()
function to get the element using its id, and on the next line, we printed the current input value using the console.log() function. After that, we used the value property to set the input value to our desired value, and after that, we printed the new value on the console. You can also use the querySelector()
function to select the element whose input value you want to change. For example, let’s repeat the above example using the querySelector()
function. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<form>
<input type="text" id= "123" name="ABC" value="Some Value">
</form>
</body>
<script type="text/javascript">
var Myelement = document.querySelector('input[name="ABC"]');
console.log(Myelement.value);
Myelement.value = "New value";
console.log(Myelement.value);
</script>
</html>
Output:
Some Value
New value
In the above code, we used the querySelector()
function to get the element.
Change the Input Value Using the setAttribute()
Function in JavaScript
We can also use the setAttribute()
function instead of the value
property to set the input value. We can also use the forms()
function instead of the getElementById()
or querySelector()
function to get the element using the form name and input name. For example, let’s repeat the above example with the setAttribute()
and froms()
function. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<form name="FormABC">
<input type="text" id= "123" name="ABC" value="Some Value">
</form>
</body>
<script type="text/javascript">
var Myelement = document.forms['FormABC']['ABC'];
console.log(Myelement.value);
Myelement.setAttribute('value','New value');
console.log(Myelement.value);
</script>
</html>
Output:
Some Value
New value
As you can see, the output of all these methods is the same, so you can use whatever method you like depending on your requirements.