How to Mask Input Text Without a Plugin Using JavaScript
- Input Masking for Credit Card Numbers in JavaScript
- Zipcode Input Masking in JavaScript
- Telephone Number With Parenthesis Input Masking in JavaScript
- Customize the Input Masking for Different Fields in JavaScript
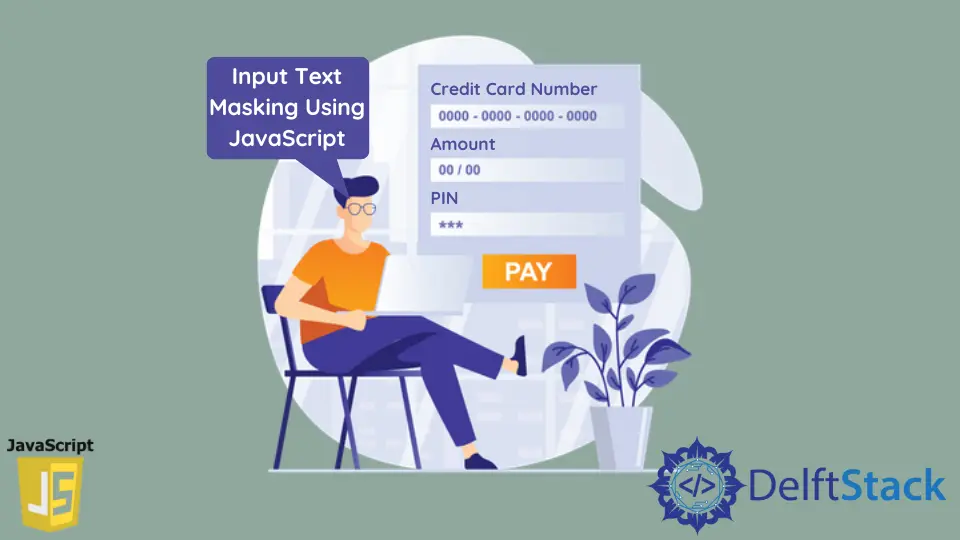
The JavaScript Input Mask or masked textbox is a control that provides an easy and reliable way for the user to collect input based on a standard mask. For example, it allows you to capture phone numbers, date values, credit card numbers, and other standard format values.
You may require enabling the users to enter only the specific data in a particular format in certain scenarios. For example, zip codes and credit card entries are within a specific pattern where you may restrict particular data formats.
The input-masking library allows the user to mask an input field using a particular data entry format.
This JavaScript module for masking input fields will make entries of spaces, dashes, and other characters automatically. As a result, users can concentrate solely on entering numbers or alphabets.
This is especially beneficial when data entry operators using a web interface are required to make many entries.
By going through the examples given below, you will learn how to set up this library on your web page.
Input Masking for Credit Card Numbers in JavaScript
In this example, a general format for the credit card is given. The user can try to enter numbers and alphabets without inputting the spaces.
You will see that the spaces are added automatically, as shown in the screenshot below.
You can access the code for this example below.
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="css/masking-input/masking-input.css"/>
<style>
/* additional styles */
li {line-height: 2; clear: both;}
label {display: inline-block; width: 200px;}
.shell span {color: pink;}
li {font-family: helvetica; font-size: 0.93rem;}
</style>
</head>
<body>
<ul>
<li>
<label for="cc">Credit Card Number</label>
<input id="cc" type="tel" name="ccnum" placeholder ="XXXX XXXX XXXX XXXX" pattern="\d{4} \d{4} \d{4} \d{4}" class="masked" title="Enter the 16 digits of credit card">
</li>
</ul>
<script src="js/maskinginput/maskinginput.js" data-autoinit="true"></script>
</body>
</html>
Zipcode Input Masking in JavaScript
The zip code is a mix of alpha-numeric, so this is an interesting case for input masking. The placeholder for entering the zip code is made so that a user knows if a character is required or a number.
See the screenshot below.
Rather than using X
in the placeholder, you saw that A1B2C3
was used, so you know the required format. You also do not need to enter the space; keep entering the code in the correct format.
The markup for creating the zip code input masked field is given below.
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="css/masking-input/masking-input.css"/>
<style>
/* additional styles */
li {line-height: 2; clear: both;}
label {display: inline-block; width: 200px;}
.shell span {color: pink;}
li {font-family: helvetica; font-size: 0.93rem;}
</style>
</head>
<body>
<ul>
<li>
<label for="zipca">Enter zip code</label>
<input id="zipca" type="text" name="zipcode" placeholder="A1B2C3" pattern="\w\d\w\d\w\d" class="masked" datacharset="_X_ X_X" title="Enter zip code">
</li>
</ul>
<script src="js/mask-input/mask-input.js" data-autoinit="true"></script>
</body>
</html>
Telephone Number With Parenthesis Input Masking in JavaScript
In this example, the telephone field will be masked, and the script will manage the parenthesis. This is shown in the screenshot below.
The code for this is given below.
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="css/masking-input/masking-input.css"/>
<style>
/* additional styles */
li {line-height: 2; clear: both;}
label {display: inline-block; width: 200px;}
.shell span {color: pink;}
li {font-family: helvetica; font-size: 0.93rem;}
</style>
</head>
<body>
<ul>
<li>
<label for="tel">Enter Phone</label>
<input id="tel" type="tel" name="phone number" placeholder="(XXX) XXX-XXXX" pattern="\(\d{3}\) \d{3}\-\d{4}" class="masked" title="Enter number without parenthesis">
</li>
</ul>
<script src="js/mask-input/mask-input.js" data-autoinit="true"></script>
</body>
</html>
Customize the Input Masking for Different Fields in JavaScript
The most important thing to notice in all of the instances above is the pattern definition. For example, the following pattern is placed in the input field in the telephone example above.
pattern = '\(\d{3}\) \d{3}\-\d{4}'
If you want to allow the users to enter four digits in parenthesis rather than 3, change it to 4. To show how to customize the input fields, you will also add another dash, so this is how the pattern looks:
pattern = '\(\d{4}\) \d{3}\--\d{4}'
The user will also need to make changes in the placeholder as follows.
placeholder = '(XXXX) XXX--XXXX'
The screenshot for this is given below.
The code for this is given below.
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="css/masking-input/masking-input.css"/>
<style>
/* additional styles */
li {line-height: 2; clear: both;}
label {display: inline-block; width: 200px;}
.shell span {color: pink;}
li {font-family: helvetica; font-size: 0.93rem;}
</style>
</head>
<body>
<ul>
<li>
<label for="tel">Enter Phone</label>
<input id="tel" type="tel" name="phone number" placeholder="(XXXX) XXX--XXXX" pattern="\(\d{4}\) \d{3}\--\d{4}" class="masked" title="Enter number without parenthesis">
</li>
</ul>
<script src="js/mask-input/mask-input.js" data-autoinit="true"></script>
</body>
</html>
Similarly, the user can also make the changes by adding the numbers or characters in the required format.