How to Get Input Value in JavaScript
-
Use
document.getElementById(id_name)
to Get Input Value in JavaScript -
Use
document.getElementsByClassName('class_name')
to Get Input Value in JavaScript -
Use
document.querySelector('selector')
to Get Input Value in JavaScript
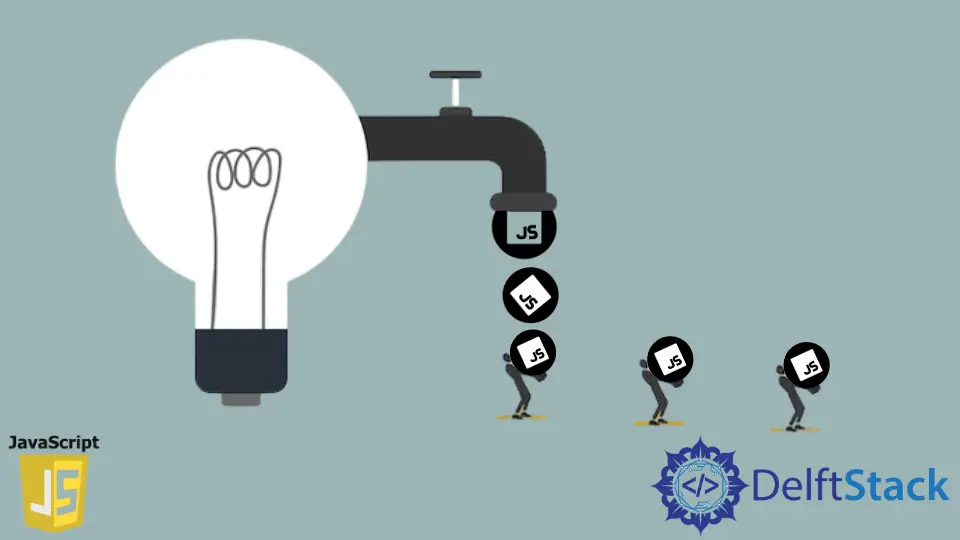
We can get the value of input without wrapping it inside a form element in JavaScript by selecting the DOM input element and use the value
property.
JavaScript has different methods for selecting the DOM input element. Every method below will have a code example, which you can run on your machine.
Use document.getElementById(id_name)
to Get Input Value in JavaScript
We give the id
property to our Dom input element, and then use document.getElementById(id_name)
to select DOM input element, you can simply use the value
property.
Example:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Use document.getElementsByClassName('class_name')
to Get Input Value in JavaScript
We give the class property to our Dom input element, and then use document.getElementsByClassName('class_name')
to select DOM input element, but if we have different Dom input elements with the same class name, then it will return an array of Dom inputs, so we should specify which one we will select by giving index number: document.getElementsByClassName('class_name')[index_number].value
.
Example:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Use document.querySelector('selector')
to Get Input Value in JavaScript
The document.querySelector('selector')
uses CSS
selectors which means, it can select elements by id, class, tag name, and name property of the DOM element.
Example:
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
Example:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>